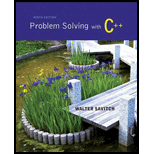
Explanation of Solution
The function “unitPrice()” with “static_cast<double>(2)” is executed in the C++ language which gives the output as follows,
// Include required header files
#include <iostream>
using namespace std;
// Declaration of unitPrice() function
double unitPrice(int diameter, double price);
// Definition of unitPrice() function
double unitPrice(int diameter, double price){
// Define a constant value for PI
const double PI = 3.14159;
// Declare the variables
double radius, area;
// Compute radius
radius = diameter/static_cast<double>(2);
// Compute area
area = PI * radius * radius;
// Compute the unit price and return the result
return (price/area);
}
// Definition of main() function
int main() {
// Call the unitPrice() function and display the result
cout << "Price: " << unitPrice(13, 14) <<"\n";
// Return 0
return 0;
}
Output for the above function is as follows,
Price: 0.105476
The function “unitPrice()” with the constant “2...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Problem Solving with C++ (9th Edition)
- Problem 1: Given the API rand7 () that generates a uniform random integer in the range [1, 7], write a function rand10 () that generates a uniform random integer in the range [1, 10]. You can only call the API rand7 (), and you shouldn't call any other API. Please do not use a language's built-in random API.arrow_forwardWrit a program with c++ language to do the following tasks : The user can input one original image and target scales . According to the user’s requirement, this image can resized to the target scale. The program can show the original image and the resized image. *The core function should be Bilinear_interpolation(original image, target height, target width) The output of this function is the resized image and then the user can save it. *You can use image processing libraries to assist your codes, such as Opencv. The functions included in the libraries which are off‐the‐shelf can be used to read images, operate images and save images.arrow_forwardGiven the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()? Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4][1]);return 0;}arrow_forward
- Given the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4]);return 0;}arrow_forwardpython Define stubs for the functions get_user_num() and compute_avg(). Each stub should print "FIXME: Finish function_name()" followed by a newline, and should return -1. Each stub must also contain the function's parameters.Sample output with two calls to get_user_num() and one call to compute_avg():FIXME: Finish get_user_num() FIXME: Finish get_user_num() FIXME: Finish compute_avg() Avg: -1 i keep getting errors on this onearrow_forwardUsing c++ In main.cpp, complete the function RollSpecificNumber() that takes in three parameters: a GVDie object, an integer representing a desired face number of a die, and an integer representing the goal amount of times to roll the desired face number. The function RollSpecificNumber() then rolls the die until the desired face number is rolled the goal amount of times and returns the number of rolls required. Note: For testing purposes, the GVDie objects are created in the main() function using a pseudo-random number generator with a fixed seed value. The program used during development uses a seed value of 15, but when submitted, different seed values will be used for each test case. Refer to the textbook section on random numbers to learn more about pseudo-random numbers. Ex: If the GVDie objects are created with a seed value of 15 and the input of the program is: 3 20 the output is: It took 140 rolls to get a "3" 20 times. #include <iostream>#include "GVDie.h"using…arrow_forward
- Define stubs for the functions get_user_num() and compute_avg(). Each stub should print "FIXME: Finish function_name()" followed by a newline, and should return -1. Each stub must also contain the function's parameters.Sample output with two calls to get_user_num() and one call to compute_avg(): FIXME: Finish get_user_num() FIXME: Finish get_user_num() FIXME: Finish compute_avg() Avg: -1 ''' Your solution goes here ''' user_num1 = 0user_num2 = 0avg_result = 0 user_num1 = get_user_num()user_num2 = get_user_num()avg_result = compute_avg(user_num1, user_num2) print('Avg:', avg_result)arrow_forwardWrite a function called number_matrix(r,c) that takes two parameters r and c and PRINTS ON THE SCREEN a matrix of numbers as follows: 1 2 3 4 5 .... 2 3 4 5 6 .... 3 4 5 6 7 .... ... In this pattern, each row contains c numbers and there are r rows. For example, number_matrix(4,3) results in: 1 2 3 2 3 4 3 4 5 4 5 6arrow_forwardDefine stubs for the functions get_user_num() and compute_avg(). Each stub should print "FIXME: Finish function_name()" followed by a newline, and should return -1. Each stub must also contain the function's parameters. Sample output with two calls to get_user_num() and one call to compute_avg(): FIXME: Finish get_user_num( ) FIXME: Finish get_user_num() FIXME: Finish compute_avg() Avg: -1 Learn how our autograder works 461710.3116374.qx3zqy7 1234 3 111 Your solution goes here ** 4 user_num1 = 0 5 user_num2 = 0 6 avg_result = 0 7 8 user_num1 = get_user_num() 9 user_num2 = get_user_num() 10 avg_result = compute_avg(user_num1, user_num2) 11 12 print (f'Avg: {avg_result}')arrow_forward
- Write a function in C++ language which takes two parameters as arguments, the first being an integer array and the second being the size of this integer array, and sorts this integer array in descending order. Write a similar function which takes the same arguments, but sorts the integer array in ascending order. Write a C++ program which takes integer values from user, inserts them into an integer array, uses these two functions to sort the array in ascending and descending order and prints the results on screen. You can take any size of the integer arrayarrow_forwardIn C programming language: Write a function that takes one double parameter, and returns a char. The parameter represents a grade, and the char represents the corresponding letter grade. If you pass in 90, the char returned will be ‘A’. If you pass in 58.67, the char returned will be an ‘F’ etc. Use the grading scheme on the syllabus for this course to decide what letter to return.arrow_forwardConsider the function definition: void Demo (int intVal, float& floatVal ) { intVal = intVal * 2; floatVal = float(intVal) + 3.5; } a. Suppose that the caller has variables int myInt and float myFloat whose values are 20 and 4.8, respectively. What are the values of myInt and myFloat after return from the following function call? Demo(myInt, myFloat); myInt: myFloat: b. Also, describe what happens in MEMORY when the Demo function is called. Think about the by reference and by value parameters.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
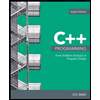