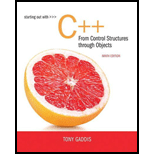
Concept explainers
Rewrite the following
#include <iostream> using namespace std; int main()
{
int selection;
cout << “Which formula do you want to see?\n\n”;
cout << “ 1. Area of a circle\n”;
cout << “2. Area of a rectangle\n”;
cout << “3. Area of a cylinder\n”;
cout << “4. None of them\n”;
cin >> selection; if (selection == 1)
cout << “Pi times radius squared\n”;
else if(selection == 2)
cout << “Length times width\n”;
else if(selection == 3)
cout << “Pi times radius squared times height\n”;
else if(selection == 4)
cout << “Well okay then, good bye!\n”;
else
cout << “Not good with numbers, eh?\n”;
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Modern Database Management
Computer Science: An Overview (12th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Problem Solving with C++ (9th Edition)
- char letter1; char letter2; letter1 = 'y'; while (letter1 <= 'z') { letter2 = 'a'; while (letter2 <= 'c'){ System.out.print("" + letter1 + letter2 + " "); ++letter2; } ++letter1; } For this code why did we use "" before and after in this line? System.out.print("" + letter1 + letter2 + " "); ++letter2; What if we don't use the blank quotation would the output be same? because I don't see the reason for putting those quotation mark.arrow_forward17. Math Tutor Write a program that can be used as a math tutor for a young student. The program should display two random numbers to be added, such as 247 +129 The program should then pause while the student works on the problem. When the student is ready to check the answer, he or she can press a key and the program will display the correct solution: 247 +129 376arrow_forwardExercise I- Medicine Write a program that asks the user to enter the name of the medicine (t for ThroX, f for FeliX), his mass. The user should enter as well if he has a prescription or not. The program will calculate the dosage using: age and his ThroX without prescription -The max dosage should be 0.75 mL -The dosage = mass / 150 -The number of pills per day to take is 1 ThroX with prescription -The dosage =mass * 3 / 150 -The number of pills per day to take is 5 FeliX with prescription -The dosage = 0.77 mL FeliX without prescription -The dosage should be between 0.3 and 0.7 -The dosage = mass * 0.009 -The number of pills per day to take is 2 -The number of pills per day to take is 6 The program should display the dosage and the number of pills to be taken per day if the requirements are full filled, otherwise it display "Please check your doctor for a prescription". In case the medicine does not exist in the database, the program display “Your medicine is not in our database!"…arrow_forward
- Programming Assignment: Car Repair For this programming assignment, create a car repair program that prompts the user to enter information about a customer, the customer's vehicle, and the work performed on the customer's vehicle. Your program should then display the final car repair bill on the computer screen. Your program should accomplish the following: 1. Prompt the user to enter the following information: a. Information about the customer (name, street address, city, state, zip code, phone number). b. Information about the customer's vehicle (make, model, year). c. Information about the work performed (brief description, labor hours needed, cost of parts). 2. Use a labor cost amount of $100.00 per hour and a sales tax rate of 7.0%. 3. Calculate the following: Variable subtotal sales tax total repair bill amount Formula subtotal = labor cost per hour * number of labor hours sales tax = sales tax rate * subtotal total repair bill amount = subtotal + sales tax 4. A screen shot of a…arrow_forwardRemaining Time: 54 minutes, 49 seconds. * Question Completion Status: QUESTION 19 Write a Java program that prompts the user to enter the School Grade Level of a student and then calculate their discount amount in the regular fees structure offered during this pandemic situation. By using the below table, calculate their discount amount from the amount of the regular fee and then print the discount amount in OMR by using the appropriate selection structure. (Assume the user is entering a maximum Grade level is 12) discount amount = Regular Fees * percentage of discount rate School Grade Level Regular Fees in OMR Discount Rate >9 and 6 and 3 and 0 and <=3 30.500 5% otherwise 20.400 No discount T T T Arial 3 (12pt) v T -E E- 只i ン Click Save and Submit to save and submit. Click Save All Answers to save all answers. Save All Answers TOSHIBA F5 F6 F7 F8 F9 F10 F11 F12 INS SCNULL LOCK AD & 67 7 V 8A9 4 0arrow_forward3- Enter a=3; b-5; c=7, then clear the variable b onlyarrow_forward
- def area(side1, side2): return side1 * side2s1 = 12s2 = 6 Identify the statements that correctly call the area function. Select ALL that apply. Question options: A print(f'The area is {area(s1,s2)}') B answer = area(s1,s2) C result = area(side1,side2) D area(s1,s2)arrow_forwardc## please Write a Console Application that reads two integers, determines whether the first is a multiple of the second and displays the result. [Hint: Use the remainder operator.]arrow_forwardChallenge Problem (pyhton) T E S T S C O R E S Write a program that implements a test scores program. Valid test score entries are between 0 and 100 inclusive. The program should display a welcome message and run everything through the "main" function. have the ability to enter several test scores (try a loop) and print out the total score, as well as, the average score. continuously ask for test scores until the number 99.9 has been entered. test for valid entries and the value 99.9. If a test score is valid, the program should add the current score to the total score and update the number of test scores by one (+1), otherwise it displays an error message. Note : This assignment involves the use of a while loop and if-else decision making controls. You CANNOT use the reserved keywords break and continue for any portion of this program or any program for that matter throughout this course.arrow_forward
- Background: Game Rules The rules to the (dice) game of Pig: You will need 2 dice. To Play: a. The players each take turns rolling two die. b. A player scores the sum of the two dice thrown (unless the roll contains a 1): If a single number 1 is thrown on either die, the score for that whole turn is lost (referred to as “Pigged Out”). A 1 on both dice is scored as 25. c. During a single turn, a player may roll the dice as many times as they desire. The score for a single turn is the sum of the individual scores for each dice roll. d. The first player to reach the goal score wins unless a player scores higher subsequently in the same round. Therefore, everyone in the game must have the same number of turns. Execution and User Input This program is quite interactive with the user(s) and will take in the following information; please review the sample input / output sessions for details; we describe them again here emphasizing input. The program will prompt for the number of…arrow_forwardGuessing game: Read a number until it's equal to a chosen number (first it can be a hard-coded constant or #define, or you can check rand() later). In case of wrong guessing help the user by printing whether the guessed number is to small or too big. ( in C language)arrow_forwardFor the following code: for ( a = 1, b = 5, c = 3; a < 4; a++, b++ ) { c = a * b + c; } Indicate what values for the following variable will be when the code endsa=b=c=arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
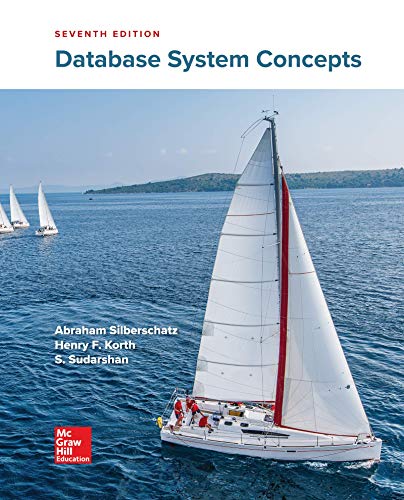
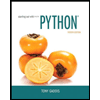
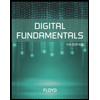
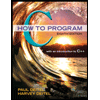
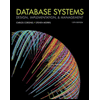
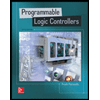