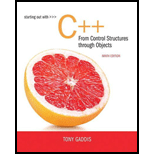
Concept explainers
// This program uses a switch-case statement to assign a
// letter grade (A, B, C, D, or F) to a numeric test score.
#include <iostream>
using namespace std;
int main()
{
double testScore;
cout << “Enter your test score and I will tell you\n";
cout << "the letter grade you earned:
cin >> testScore;
switch (testScore)
{
case (testScore < 60.0):
cout << “Your grade is F.\n”;
break;
case (testScore < 70.0):
cout << "Your grade is D.\n";
break;
case (testScore < 80.0):
cout << "Your grade is C.\n";
break;
case (testScore < 90.0):
cout << "Your grade is B.\n";
break;
case (testScore <= 100.0):
cout << "Your grade is A.\n";
break;
default:
cout << “That score isn't valid\n";
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Management Information Systems: Managing The Digital Firm (16th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Introduction To Programming Using Visual Basic (11th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- #include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward
- #include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forwardCircle Properties Write a program that prompts for and accepts the diameter of a circle as a floating point number. The program should calculate and output the area and circumference of the circle. A sample run of the program should look like this: Enter circle diameter: 2.5 The area is 4.91 and the circumference is 7.85. Use 3.14159 as your constant for pi. global main ; exposes program entry point to the linkerextern printf ; declare external functionextern scanf section .text ; start of code segment main: push rbp ; preserve base pointer mov rbp,rsp ; copy stack pointer to base pointer pop rbp ; restore base pointer mov rax, 0 ; exit status (0 = success) ret section .data ; start of initialized data segment section .bss ; start of uninitialized data segment section .dataprompt db "Enter circle diameter: ", 0format db "The area is %0.2f and the circumference is %0.2f.", 0pi dq 3.14159…arrow_forwardConvert totalMeters to hectometers, decameters, and meters, finding the maximum number of hectometers, then decameters, then meters. Ex: If the input is 815, then the output is: Hectometers: 8 Decameters: 1 Meters: 5 Note: A hectometer is 100 meters. A decameter is 10 meters. 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 18 int totalMeters; int numHectometers; int numDecameters; int numMeters; cin >>totalMeters; cout << "Hectometers: cout << "Decameters: cout << "Meters: " << numMeters << endl; " << numHectometers << endl; << numDecameters << endl; 2 3arrow_forward
- Static Variable: a variable whose lifetime is the lifetime of the program (static int x;) Dynamic Variable: It is a pointer to a variable (int *x;) Is this comparison true?arrow_forwardLowest Score Drop Write a program that calculates the average of a group of test scores, where the lowest score in the group is dropped. It should use the following functions: void getScore() should ask the user for a test score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five scores to be entered. void calcAverage() should calculate and display the average of the four highest scores. This function should be called just once by main and should be passed the five scores. int findLowest() should find and return the lowest of the five scores passed to it. It should be called by calcAverage, which uses the function to determine which of the five scores to drop. Input Validation: Do not accept test scores lower than 0 or higher than 100.arrow_forwarddebug and rewrite the codearrow_forward
- Programming Assignment: Car Repair For this programming assignment, create a car repair program that prompts the user to enter information about a customer, the customer's vehicle, and the work performed on the customer's vehicle. Your program should then display the final car repair bill on the computer screen. Your program should accomplish the following: 1. Prompt the user to enter the following information: a. Information about the customer (name, street address, city, state, zip code, phone number). b. Information about the customer's vehicle (make, model, year). c. Information about the work performed (brief description, labor hours needed, cost of parts). 2. Use a labor cost amount of $100.00 per hour and a sales tax rate of 7.0%. 3. Calculate the following: Variable subtotal sales tax total repair bill amount Formula subtotal = labor cost per hour * number of labor hours sales tax = sales tax rate * subtotal total repair bill amount = subtotal + sales tax 4. A screen shot of a…arrow_forwardC++ please #include <iostream> #include <string> using namespace std; int DateParser(string month) { int monthInt = 0; if (month == "January") monthInt = 1; else if (month == "February") monthInt = 2; else if (month == "March") monthInt = 3; else if (month == "April") monthInt = 4; else if (month == "May") monthInt = 5; else if (month == "June") monthInt = 6; else if (month == "July") monthInt = 7; else if (month == "August") monthInt = 8; else if (month == "September") monthInt = 9; else if (month == "October") monthInt = 10; else if (month == "November") monthInt = 11; else if (month == "December") monthInt = 12; return monthInt; } int main () { while (std::getline(std::cin, date)) { if (date == "-1") { break; } std::string month = date.substr(0, date.find(" ")); int firstComma = date.find(","); if (firstComma == std::string::npos) { continue; } int day; try { day = std::stoi(date.substr(month.length() + 1, firstComma - month.length() - 1)); } catch…arrow_forwardUsernames An online company needs your help to implement a program that verifies the username chosen by a new user. Their rules is described below: Username MUST contain at least 6 characters; Username cannot start with a number; Username can only contain letters or numbers. If valid, the username may be resgistered if it doesn't already exist in the system. You should not use built-in functions to determine the character type such as isnumeric() or islower(). Use the strings given alphabet and numeric to determine if each character is valid. Use the list registered to help you determine if the username is already registered. Don't forget to execute the cell below to use these strings # run this cell to create these variablesalphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'numeric = "0123456789"registered = ["john87", "topmage", "light4ever", "username2"] Write a function nameValidation which receives a String argument name. This function: has name String…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
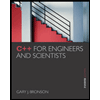