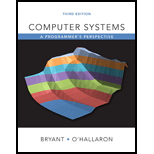
Concept explainers
For a function with prototype
long decoda2(long x, long y, long z);
GCC generates the following assembly code:
Parameters x, y, and z are passed in registers %rdi, %rsi, and %rdx. The code stores the return value in register %rax.
Write C code for decode2 that will have an effect equivalent to the assembly code shown.

Explanation of Solution
Given assembly code:
x in %rdi, y in %rsi and z in %rdx
decode2:
subq %rdx, %rsi
imulq %rsi, %rdi
movq %rsi, %rax
salq $63, %rax
sarq $63, %rax
xorq %rdi, %rax
ret
Load Effective Address:
- The load effective address instruction “leaq” is a variant of “movq” instruction.
- The instruction form reads memory to a register, but memory is not been referenced at all.
- The first operand of instruction is a memory reference; the effective address is been copied to destination.
- The pointers could be generated for later references of memory.
- The common arithmetic operations could be described compactly using this instruction.
- The operand in destination should be a register.
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Comparison Instruction:
- The “CMP” instruction sets condition code according to differences of their two operands.
- The working pattern is same as “SUB” instruction but it sets condition code without updating destinations.
- The zero flag is been set if two operands are equal.
- The ordering relations between operands could be determined using other flags.
- The “cmpl” instruction compares values that are double word.
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination.
- It can either be a memory location or a register.
- The instruction “incq” causes 8 byte element on stack top to be incremented.
- The instruction “decq” causes 8 byte element on stack top to be decremented.
- The details of binary operations includes:
- The first operand denotes the source.
- The second operand works as both source as well as destination.
- The first operand can either be an immediate value, memory location or register.
- The second operand can either be a register or a memory location.
Corresponding C code:
// Define method decode
long decode(long x, long y, long z)
{
// Declare variable
long tmp = y - z;
//Return
return (tmp * x)^(tmp << 63 >> 63);
}
Explanation:
- The register “%rdi” has value for “x”, register “%rsi” has value for “y” and register “%rdx” has value for “z”.
- The details of assembly code is shown below:
- The instruction “subq %rdx, %rsi” performs operation “y - z” and stores result in register “%rsi”.
- The statement “long tmp = y - z” corresponds to C code.
- The instruction “imulq %rsi, %rdi” multiplies result of operation with “x” and stores result in register “%rdi”.
- The statement “(tmp * x)” corresponds to C code.
- The instruction “movq %rsi, %rax” moves value in register “%rsi” to register “%rax”.
- The instruction “salq $63, %rax” performs left shift on value in register “%rax”.
- The statement “tmp << 63” corresponds to C code.
- The instruction “sarq $63, %rax” performs right shift on value in register “%rax”.
- The statement “tmp << 63 >> 63” corresponds to C code.
- The instruction “xorq %rdi, %rax” performs “XOR” operation on values in registers “%rax” and “%rdi”.
- The statement “return (tmp * x)^(tmp << 63 >> 63)” corresponds to C statement.
- The instruction “subq %rdx, %rsi” performs operation “y - z” and stores result in register “%rsi”.
Want to see more full solutions like this?
Chapter 3 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
C How to Program (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
Database Concepts (8th Edition)
- Consider the following C functions and assembly codeAssume that long is 64 bits, int is 32 bits, short is 16 bits, and therepresentation is two’s complement.Assembly Code: imulq %rsi, %rdiimulq %rdx, %rsiaddq %rsi, %rdileaq (%rdi,%rdi,2), %raxsalq $3, %raxret long functionA(long a, long b, long c){long d = a*b;long e = b*c;return 18 * (d+e);} long functionB(long a, long b, long c){long d = a*b;long e = b*c;return 24 * (d*e);} long functionC(long a, long b, long c){long d = a*b;long e = b*c;return 24 * (d+e);} long functionD(long a, long b, long c){long d = a*b;long e = b*c;return 32 * (d*e);} Which of the functions compiled into the assembly code shown?arrow_forwardFor the following assembly code that is vaguely MIPS-like, trace itsexecution in both the R3000 and R4000 pipelines using a Gantt chart (ortable of some kind).LOAD R1, Memory(12340)LOAD R2, Memory(12350)ADD R3, R1, R2 // R3 = R1 + R2ADD R3, R3, R3 // R3 = R3 + R3STORE R3, Memory(12360)arrow_forwardNASM CODE, modify the code below Write an assembly program for the processor family x86-32 that reads three signed interger numbers from the standard input and writes the greatest of them on the console. Use the C functions scanf and printf for the management of data input and data output. section .datamsg db "The largest number is: %d", 0xa,0v1 equ -47v2 equ 32v3 equ 21num1 times 4 db 0num2 times 4 db 0num3 times 4 db 0 section .textextern printfglobal main ;must be declared for using gcc main: ;tell linker entry point mov dword [num1], v1mov dword [num2], v2mov dword [num3], v3 mov ecx, [num1]cmp ecx, [num2]jg check_third_nummov ecx, [num2]check_third_num: cmp ecx, [num3]jg _exitmov ecx, [num3]_exit:;Print the greatest numberpush ecxpush msgcall printf ;Exit process mov eax, 1int 80harrow_forward
- Consider a RISC-V assembly function func1. func1 has three passing arguments stored in registers a0, a1 and a2, uses temporary registers t0-t3 and saved registers s4–s10. func1 needs to call func2 and other functions may call func1 func2 has two passing arguments stored in registers a0 and a1, respectively. In func1, after the program returns to func1 from func2, the code needs the original values stored in registers t1 and a0 before it calls func2. How many words are the stack frames of function func1? Indicate which registers are stored on the stack of func1? pseudo-instructions are not allowed except “j target_label” and “jr ra”.arrow_forwardDescribe the implementation of the TestandSet instruction. Show how the followingalgorithm using TestandSet does not satisfy the bounded wait requirement?Shared data: boolean lock = false;Process Pi:do {while (TestAndSet(lock)) ;critical sectionlock = false;remainder section}arrow_forwardImplement a shared-memory pthread program on in C to find Prefix Sum of n integers. Keep theparallel overheads to a minimum. (One barrier synchronization may be enough.) Vary n as 210, 215, and 220 (or moreif desired), and p, the number of processes, from 1 to 8, and obtain the speedup relative to the sequential timingswithout any overheads. Produce a speedup plot with p on x-axis and Sp on y-axis. Each n will result in a separatespeedup curve. Use same plot for all the curves to see how speedup varies as problem size increases.arrow_forward
- Write a program in MIPS assembly that allows replacing a substring with another substring. Use any function and variable of your choice. For example: Input: - Initial text: XXabXXcd - Word to replace : XX - Replacement word: YY Output: YYabYYcdarrow_forwardHere is my question from homework: int x; short y, z; cin>>x; y=x; z= y+2; cout<<"First number is: "<<x<<endl; cout<<"Second number is: "<<y<<endl; cout<<"Third number is: "<<z<<endl; End of HW problem My question is how do I utilize short variable type in MIPS assembly code and get it to work with int data type?arrow_forwardWrite code to implement the expression A = (B + C) × (D + E) on three-, two-, one-, and zero-address machines. In accordance with programming language practice, computing the expression should not change the values of its operands.arrow_forward
- Translate the following C code to MIPS assembly (in two separate files). Run the program step by step and observe the order of instructions being executed and the value of $sp. int main() { int x=2; z=Subfunc(x); printf(“Value of z is: %d”, z); } int Subfunc(int x) { return x+1;}arrow_forwardBinary Lab Phase 3 I need help getting the inputs to diffuse phase 3. Here is the assembly code: Dump of assembler code for function phase_3:=> 0x0000000000400f53 <+0>: sub $0x18,%rsp0x0000000000400f57 <+4>: lea 0x8(%rsp),%r80x0000000000400f5c <+9>: lea 0x7(%rsp),%rcx0x0000000000400f61 <+14>: lea 0xc(%rsp),%rdx0x0000000000400f66 <+19>: mov $0x4025de,%esi0x0000000000400f6b <+24>: mov $0x0,%eax0x0000000000400f70 <+29>: callq 0x400c30 <__isoc99_sscanf@plt>0x0000000000400f75 <+34>: cmp $0x2,%eax0x0000000000400f78 <+37>: jg 0x400f7f <phase_3+44>0x0000000000400f7a <+39>: callq 0x401624 <explode_bomb>0x0000000000400f7f <+44>: cmpl $0x7,0xc(%rsp)0x0000000000400f84 <+49>: ja 0x401086 <phase_3+307>0x0000000000400f8a <+55>: mov 0xc(%rsp),%eax0x0000000000400f8e <+59>: jmpq *0x402600(,%rax,8)0x0000000000400f95 <+66>: mov $0x66,%eax0x0000000000400f9a <+71>: cmpl…arrow_forwardWrite the following c/c++ code into RISC-V assembly please. It has to follow the conventions about functions and stacks. void f(char* x, char* y) { char *srcPtr = x; char *dstPtr = y; if (strlen(x) > strlen(y) { srcPtr = y; dstPtr = x; } for (int i = 0; i < strlen(srcPtr); i++) { dstPtr[i] = srcPtr[i]; } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
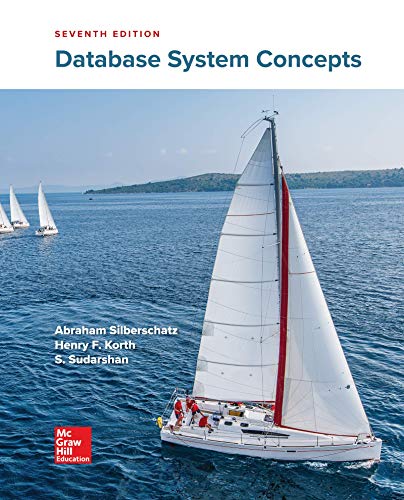
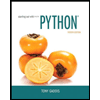
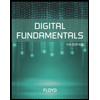
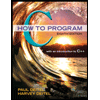
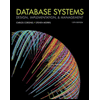
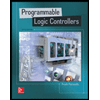