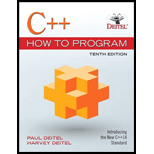
Concept explainers
(Date Create a class called Date that includes three pieces of information as data members-a month (type int), a day (type int) and a year (type int). Your class should have a constructor with three parameters that uses the parameters to initialize the three data members. For the purpose of this exercise, assume that the values provided for the year and day are correct, but ensure that the month value is in the range 1-12; if it isn't, set the month to 1. Provide a set and a get function for each data member. Provide a member function display Date that displays the month, day and year separated by forward slashes (/). Write a test

Learn your wayIncludes step-by-step video

Chapter 3 Solutions
C++ How to Program (10th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
C How to Program (8th Edition)
Starting Out with Python (4th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- Language: C++ Next a thirst level (as double value) should be added to the properties of a critter. Add a new constructor that takes five parameters for setting all properties of a critter. Make also sure that the existing constructors will still work. For the existing constructors, the thirst level should be set to the same level as the hunger level. Your existing testcritter.cpp must still be able to run in its unchanged form. So the already existing constructors need to support the change. Name the files Critter.h, Critter.cpp and testcritter.cpp. Finally, you should adapt the print method for printing on the screen also the value of the thirst level as a double. The client program testcritter.cpp may contain one additional line, where the constructor taking five parameters is being called. You can assume that the setting values are always valid.arrow_forwarda) Implement a class Point with three attributes, x, y, and z.b) Implement an init method with an optional parameter type: 1) Set the default value of x, y, and z to 0.c) Implement a display method to print the values of x, y, and z as the example output below.d) Instantiate two objects of type Point, one with arguments,1, 2, and 3, and the other one without any arguments.e) Call display() to print x, y, and z. Example Output(x, y, z): (1, 2, 3)(x, y, z) : (0, 0, 0)arrow_forwardQuestion 2 (Marks: 30) Write a Java console application to print a report and determine if a hospital is due for a health inspection. Make use of an abstract class named Inspection that contains variables to store the hospital location, hospital name and the years since the last inspection. This class also creates methods to get the hospital location, name, and years since the last hospital inspection by using the get methods. Create a constructor that accepts the hospital location, name and years since the last inspection as parameters. The Inspection class must implement an iInspection interface that contains the following: interface iInspection { public String getLocation(); public String getHospitalName(); public int getYearsSinceInspection(); public String getInspectionNeeded(); } Create a subclass called Hospital Inspections that extends the Inspection class. The Hospital Inspection class must contain a constructor to accept…arrow_forward
- (After reading the instructions given in the pictures attached, read the following) Class membersdoctorType Class must contain at least these functionsdoctorType(string first, string last, string spl); //First Name, Last Name, Specialty void print() const; //Formatted Display First Name, Last Name, Specialtyvoid setSpeciality(string); //Set the doctor’s Specialtystring getSpeciality(); //Return the doctor’s SpecialtypatientType Class must contain at least these functionsvoid setInfo(string id, string fName, string lName,int bDay, int bMth, int bYear,string docFrName, string docLaName, string docSpl,int admDay, int admMth, int admYear,int disChDay, int disChMth, int disChYear);void setID(string);string getID();void setBirthDate(int dy, int mo, int yr);int getBirthDay();int getBirthMonth();int getBirthYear();void setDoctorName(string fName, string lName);void setDoctorSpl(string);string getDoctorFName();string getDoctorLName();string getDoctorSpl();void…arrow_forwardPlease written by computer source Problem Description:(The Account class) Design a class named Account that contains:A private int data field named id for the account (default 0).A private string data filed named first name for customer first name.A private string data filed named last name for customer last name.A private double data field named balance for the account (default 0).A private double data field named annualInterestRate that stores thecurrent interest rate (default 0). Assume all accounts have the sameinterest rate.A private Date data field named dateCreated that stores the date whenthe account was created.A no-arg constructor that creates a default account.A constructor that creates an account with the specified id, firstname, last name and initial balance.The accessor and mutator methods for id, name, balance, andannualInterestRate.The accessor method for dateCreated.A method named getMonthlyInterestRate() that returns the monthlyinterest rate.A method named withdraw…arrow_forward(Triangle class) Design a new Triangle class that extends the abstract Geometricobject class. Draw the UML diagram for the classes Triangle and Geometricobject then implement the Triangle class. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filed. The program should create a Tri- angle object with the se sides, and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.arrow_forward
- (The Person, Student, Employee, Faculty, and Staff classes) Design a class named Person and its two subclasses named Student and Employee. Make Faculty and Staff subclasses of Employee. A person has a name, address, phone number, and e-mail address. A student has a class status (freshman, sophomore, junior, or senior). Define the status as a constant. An employee has an office, salary, and date hired. Use the MyDate class defined in Programming Exercise 10.14 – see the textbook - to create an object for date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString method in each class to display the class name and the person's name. Draw the UML diagram for the classes and implement them. Write a test program that creates a Person, Student, Employee, Faculty, and Staff, and invokes their toString() methods.arrow_forwardIt is possible for a class to have more than one constructor.A) This is correct. B) The answer is False.arrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ALSO ADD SCREENSHOTS OF OUTPUT. Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file. Make the data members and functions in your program const where applicablearrow_forward
- Question 12 Write ONLY Header (No body0) of function for each question. You can use any valid variable name for the input parameters. Assume that the following is a tester program of GameObject class, and answer the questions. int main (0{ GameObject player (3, 100, 'M'); GameObject enemy (4, 100, 'F'); player.print(); Guess the GameObject class and functions from the code above, and write the header of constructor. You can use any valid variable name for the input parametersarrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward(Triangle class) Design a new Triangle class that extends the abstract GeometricObject class. Draw the UML diagram for the classes Triangle and GeometricObject and then implement the Triangle class. Write a test program that prompts the user to enter three sides of the triangle, a colour, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the colour and filled properties using the input. The program should display the area, perimeter, colour, and true or false to indicate whether it is filled or not.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
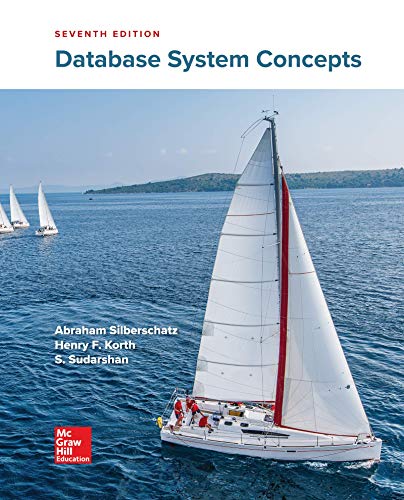
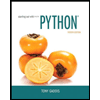
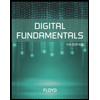
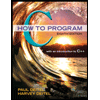
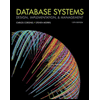
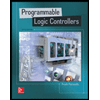