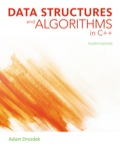
EBK DATA STRUCTURES AND ALGORITHMS IN C
4th Edition
ISBN: 9781285415017
Author: DROZDEK
Publisher: YUZU
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 3, Problem 17E
Program Plan Intro
Implementation of circular singly linked list
Program plan:
- Create a class IntCircularSLLNode for list node.
- Create a class IntCircularSLList for circular list using singly linked list.
- Define a function “createList()” for creating a circular singly linked list.
- Define a function named “displayList()” for printing the list.
- Define a function named “addToHead()” to add a node at head position of the circular singly linked list.
- Define a function named “addToTail()” to add a node at the tail position of the circular singly linked list.
- Define a function named “isInList()” for checking the availability of the node in the list.
- Define a function named “deleteNode()” to delete a node at a specific position in the list.
- Define a function named “deleteFromHead()” to delete a node at a head position of the circular singly linked list.
- Define a function named “deleteFromTail()” for deleting a node at a tail position of the circular singly linked list.
- Define a function named “isEmpty()” for checking the availability of nodes in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A-
Declare a self-referential structure
for a linked list having one data
StudentNode
field called GPA (float), and one pointer to
StudentNode called next.
B-
Write a non-recursive function
-
that counts all the GPAS that are less than or
equal to 2 in your linked list starting from the
head of the list.
Example: If the list is 1.9->2->3.5->4->1.8, the
function should return 3.
C-
Write a recursive function that
prints all the GPAS that are higher or equal to 3.5
in your linked list starting from the head of the
list.
Example: If the list is 1.9->2->3.5->4->1.8, the
function should print: 3.5->4.
Every time you write a non-const member function for a linked list, you should always think about if that function is preserving your class invariants.
Group of answer choices
A. True
B. False
C++ programming
design a class to implement a sorted circular linked list. The usual operations on a circular list are:Initialize the list (to an empty state).
Determine if the list is empty.
Destroy the list.
Print the list.
Find the length of the list.
Search the list for a given item.
Insert an item in the list.
Delete an item from the list.
Copy the list.
Write the definitions of the class circularLinkedList and its member functions (You may assume that the elements of the circular linked list are in ascending order). Also, write a program to test the operations of your circularLinkedList class.
NOTE: The nodes for your list can be defined in either a struct or class. Each node shall store int values.
Chapter 3 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
Ch. 3 - Prob. 1ECh. 3 - Prob. 2ECh. 3 - Prob. 3ECh. 3 - Prob. 4ECh. 3 - Prob. 5ECh. 3 - Prob. 6ECh. 3 - Prob. 7ECh. 3 - Prob. 8ECh. 3 - Prob. 9ECh. 3 - Prob. 10E
Ch. 3 - Prob. 11ECh. 3 - Prob. 12ECh. 3 - Prob. 13ECh. 3 - Prob. 14ECh. 3 - Prob. 15ECh. 3 - Prob. 16ECh. 3 - Prob. 17ECh. 3 - Prob. 18ECh. 3 - Prob. 19ECh. 3 - Prob. 20ECh. 3 - Prob. 21ECh. 3 - Prob. 22ECh. 3 - Prob. 23ECh. 3 - Prob. 24ECh. 3 - Prob. 25ECh. 3 - Prob. 1PACh. 3 - Prob. 2PACh. 3 - Prob. 3PACh. 3 - Prob. 5PACh. 3 - Prob. 7PA
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For this assignment, you need to implement link-based List and derivative ADTs in Java. To complete this, you will need the following: A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1. A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private. The attribute names for the Node and Linked List are the words in bold in #1 and #2. For the Linked List, implement the most common linked-list behaviors as explained in class - getters/setters/constructors/destructors for the attributes of the class, (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the…arrow_forwardJAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forwardPlease,help me by providing C++ programing solution. Do not use the LinkedList class or any classes that offers list functions. Implement a LinkList in C++. Pease,implement with an ItemType class and a NodeType structThe program should read a data file,and the data file has two lines of data as follow:100, 110, 120, 130, 140, 150, 160100, 130, 160The program will first add all of the numbers from the file,then display all of them,then delete.arrow_forward
- Java - Write a non-member method for a "enqueue" that utilizes a doubly linked list. There are three variables available (can be sent) to the method; a “front” reference, a “rear” reference, and a data element (string) (you decide what you need). Empty case is when front and rear are NULL. The node is defined as follows: class node{ node after; node before string data }arrow_forwardQuestion 5.1 Write a Python program that creates an unordered, singly-linked list consisting of 8 items. Each item in the linked list should be a number. You can use the code given in the lecture slides for your Node and UnorderedList classes. Your node class should contain the following functions: • a constructor • get_data() – returns the data in the node • get_next() – returns the next node in the list set_data() – sets the data in the node to the value given as a parameter set_next() – sets the node that this node links to, to the node given as a parameter Your UnorderedList class should contain the following functions: • a constructor • add() – adds a node to the head of the list, containing the number given in the parameter • is_empty() – returns True if the list is empty, and False otherwise • size() – returns the size of the list • print_list() – displays the contents of all nodes in the list Add code to create an UnorderedList object, and call its add() function to add 8 items…arrow_forwardCreate an implementation of a doubly linked DoubleOrderedList class. You will need to create a DoubleNode class, a DoubleList class, and a DoubleIterator classarrow_forward
- A- Declare a self-referential structure StudentNode for a linked list having one data field called GPA (float), and one pointer to StudentNode called next. B- Write a non-recursive function that prints all the GPAS that are less than or equal to 2 in your linked list starting from the head of the list. Example: If the list is 1.9->2->3.5->4->1.8, the function should print: 1.9->2->1.8. C- Write a recursive function that counts all the GPAS that are higher or equal to 3.5 in your linked list starting from the head of the list. Example: If the list is 1.9->2->3.5->4->1.8, the function should return 2.arrow_forwardWrite a function, to be included in an unsorted linked list class, called replace_item, that will receive two parameters, one called olditem, the other called newitem. The function will replace all occurrences of olditem with newitem ( if olditem exists !! ) and it will return the number of replacements done.arrow_forwardIn c++ , write a program to create a structure of a node, create a class Linked List. Implement all operations of a linked list as member function of this class. • create_node(int); • insert_begin(); • insert_pos(); • insert_last(); • delete_pos(); • sort(); • search(); • update(); • reverse(); • display(); ( Drop coding in words with screenshot of output as well )arrow_forward
- In C++ Plz LAB: Grocery shopping list (linked list: inserting at the end of a list) Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts ItemNode.h Default Code: #include <iostream>#include <string>using namespace std; class ItemNode {private: string item; ItemNode* nextNodeRef; public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; } // Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; } // ConstructorItemNode(string itemInit, ItemNode *nextLoc) {this->item = itemInit;this->nextNodeRef = nextLoc;} // Insert node after this…arrow_forward3.Write a generic class called GenericStack<T> that represents a stack structure. A stack structure follow the strategy last-in-first-out, which means that the last element added to the stack, is the first to be taken out. The GenericStack class has the following attributes and methods: --An attribute ArrayList<T> elements which represents the elements of the stack.(All of you refer collection framework for ArrayList. or you can use an array to hold the elements of Stack.)[Refer the following links to have intro on ArrayList: https://www.w3schools.com/java/java_arraylist.asp, https://www.geeksforgeeks.org/arraylist-in-java/] --A constructor that creates the ArrayList or an Array --A method push(T e) which adds the element to the ArrayList<T> or array. --A method pop() which removes the last element of the ArrayList<T> (last element added), if the list is not already empty and returns it. --A method print() which prints the elements of the stack starting from the…arrow_forwardAdd the following operation to the class orderedLinkedList: void mergeLists(orderedLinkedList<Type> &list1, orderedLinkedList<Type> &list2); //This function creates a new list by merging the //elements of list1 and list2. //Postcondition: first points to the merged list // list1 and list2 are empty Consider the following statements: orderedLinkedList<int> newList; orderedLinkedList<int> list1; orderedLinkedList<int> list2; Suppose list1 points to the list with the elements 2 6 7, and list2 points to the list with the elements 3 5 8. The statement newList.mergeLists(list1, list2); creates a new linked list with the elements in the order 2 3 5 6 7 8, and the object newList points to this list. Also, after the preceding statement executes, list1 and list2 are empty. 2. Write the definition of the function template mergeLists to implement the operation mergeLists.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
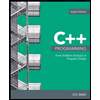
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning