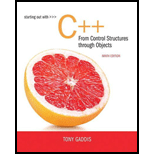
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 7PC
Program Plan Intro
Queue Converter
Program Plan:
DynIntQueue.h:
- Include required header files.
- Declare a class named “DynIntQueue”; inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Declare a variable “value”.
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable “numItems”.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue()”, “dequeue()”, “isEmpty()”, “isFull()”, and “clear()”.
- Inside the “private” access specifier,
DynIntQueue.cpp:
- Include required header files.
- Give definition for the constructor.
- Assign the values.
- Give definition for the destructor.
- Call the function “clear()”.
- Give function definition for “enqueue()”.
- Make the pointer “newNode” as null.
- Assign “num” to “newNode->value”.
- Make “newNode->next” as null.
- Check whether the queue is empty using “isEmpty()” function.
- If the condition is true then, assign “newNode” to “front” and “rear”.
- If the condition is not true then,
- Assign “newNode” to “rear->next”.
- Assign “newNode” to “rear”.
- Increment the variable “numItems”.
- Give function definition for “dequeue()”.
- Assign “temp” pointer as null.
- Check if the queue is empty using “isEmpty()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make “front->next” as “temp”.
- Delete the front value.
- Make temp as front.
- Decrement the variable “numItems”.
- Give function definition for “isEmpty()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Give function definition for “clear()”.
- Declare a variable.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Declare a variable.
IntBinaryTree.h:
- Include required header files.
- Declare a class named “IntBinaryTree”. Inside the class,
- Inside the “private” access specifier,
- Give the structure declaration for the creation of node.
- Declare a variable
- Create two pointers named “left” and “right” to access the value left and right nodes respectively.
- Create a pointer named “root” to access the value of root node.
- Give function declaration for “insert ()”, “destroy_SubTree ()”, “delete_Node ()”, “make_Deletion ()”, “display_InOrder ()”, “display_PreOrder ()”, “display_PostOrder ()”, “copyTree ()”, and “setQueue ()”.
- Give the structure declaration for the creation of node.
- Inside “public” access specifier,
- Give the definition for constructor and destructor.
- Give function declaration for binary tree operations.
- Inside the “private” access specifier,
IntBinaryTree.cpp:
- Include required header files.
- Give definition for copy constructor.
- Give function definition for “insert()”.
- Check if “nodePtr” is null.
- If the condition is true then, insert node.
- Check if value of new node is less than the value of node pointer
- If the condition is true then, Insert node to the left branch by calling the function “insert()” recursively.
- Else,
- Insert node to the right branch by calling the function “insert()” recursively.
- Check if “nodePtr” is null.
- Give function definition for “insert_Node ()”.
- Create a pointer for new node.
- Assign the value to the new node.
- Make left and right node as null.
- Call the function “insert()” by passing parameters “root” and “newNode”.
- Give function definition for “destroy_SubTree()”.
- Check if the node pointer points to left node
- Call the function recursively to delete the left sub tree.
- Check if the node pointer points to the right node
- Call the function recursively to delete the right sub tree.
- Delete the node pointer.
- Check if the node pointer points to left node
- Give function definition for “search_Node()”.
- Assign false to the Boolean variable “status”.
- Assign root pointer to the “nodePtr”.
- Do until “nodePtr” exists.
- Check if the value of node pointer is equal to “num”.
- Assign true to the Boolean variable “status”
- Check if the number is less than the value of node pointer.
- Assign left node pointer to the node pointer.
- Else,
- Assign right node pointer to the node pointer.
- Check if the value of node pointer is equal to “num”.
- Return the Boolean variable.
- Give function definition for “remove()”.
- Call the function “delete_Node()”
- Give function definition for “delete_Node()”
- Check if the number is less than the node pointer value.
- Call the function “delete_Node()” recursively.
- Check if the number is greater than the node pointer value.
- Call the function “delete_Node()” recursively.
- Else,
- Call the function “make_Deletion()”.
- Check if the number is less than the node pointer value.
- Give function definition for “make_Deletion()”
- Create pointer named “tempPtr”.
- Check if the “nodePtr” is null.
- If the condition is true then, print “Cannot delete empty node.”
- Check if right node pointer is null.
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the left node child.
- Delete temporary pointer.
- If the condition is true then,
- Check is left node pointer is null
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the right node child.
- Delete temporary pointer.
- If the condition is true then,
- Else,
- Move right node to temporary pointer
- Reach to the end of left-Node using “while” condition.
- Assign left node pointer to temporary pointer.
- Reattach left node sub tree.
- Make node pointer as the temporary pointer.
- Reattach right node sub tree
- Delete temporary pointer.
- Give function definition for “display_InOrder()”.
- Check if the node pointer exists.
- Call the function “display_InOrder()” recursively.
- Print the value
- Call the function “display_InOrder()” recursively.
- Check if the node pointer exists.
- Give function definition for “display_PreOrder()”.
- Print the value.
- Call the function “display_PreOrder()” recursively.
- Call the function “display_PreOrder()” recursively.
- Give function definition for “display_PostOrder()”.
- Call the function “display_PostOrder()” recursively.
- Call the function “display_PostOrder()” recursively.
- Print value.
- Give function definition for assignment operator.
- Call the function “destroy_SubTree()”
- Call the copy constructor.
- Return the pointer.
- Copy tree function is called by copy constructor and assignment operator function
- Create a pointer named “newNode”.
- Check if “nPtr” is not equal to null
- Allocate memory dynamically.
- Assign pointer value to the new node.
- Call the function “copyTree()” by passing “nPtr” of left.
- Call the function “copyTree()” by passing “nPtr” of right
- Return the new node.
- Function definition for “setQueue()”.
- Check if the pointer “nodePtr” exists.
- Call the function “setQueue()” recursively by passing the left node.
- Call the function “setQueue()” recursively by passing the right node.
- Call the function “enqueue()” recursively by passing the left node.
Main.cpp:
- Include required header files.
- Inside “main()” function,
- Declare a variable “value” and assign it to 0.
- Create an object “intBT” for “IntBinaryTree” class.
- Insert 5 values using “insert_Node()” function.
- Display all the values by using the function “display_InOrder()”.
- Create an object “iqueue” for “DynIntQueue” class.
- Load the address to the pointer “qPtr”.
- Pass this pointer to the function “treeToQueue ()”.
- Do until the queue is not empty.
- Declare a variable.
- Call the function “dequeue()”.
- Display the value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
ListQueue
Node
Node
Node
front=
next
next=
next = nul1
rear =
data "Thome"
data "Abreu"
data - "Jones"
size - 3
The above is a queue of a waiting list. The ListQueue has a node (front)
to record the address of the front element of a queue. It also has another
node (rear) to record the address of the tail element of a queue.
4. How do you push a node with data, "Chu" to the above queue?
front.next = new Node("Chu", front);
a.
b.
front = new Node ("Chu", front)
rear = new Node("Chu", rear)
с.
d.
= new Node ("Chu", rear.next)
rear
Describe the reason of your choice.
Your answer is
(a, b, c, or d)
Will the push action take time in 0(1) or 0(n)?
Next Page
Type here to search
3- Write a program that randomly generates 10 numbers (between 1 and 8), inserts into queue and then finds how many distinct elements exist in the queue.
Example 1:
Example 2:
Queue:
2 40 3 3 2 18 4 18 18 3
Queue:
1 1 4 33 16 16 4 16 4
Output: 5
Output: 4
Notes:
• You must use ONLY queue data structure. Don't use other different data structures like string or normal (pure) array or stack or array list.
• Don't write any other method in the Qeueu class. All methods must be written in the main program.
8-Write a procedure that takes a tree (represented as a list) and returns a list whose elements are all the leaves of the tree arranged in right to left order or left to right. For example, ( leaves '((1 2) ((3 4) 5 )) returns (1 2 3 4 5) or (5 4 3 2 1). Then, Manually trace your procedure with the provided example.
Chapter 21 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- List=(Two,three,four,five,six,seven and eight)Perform the following operations on the given list above utilizing c++ code.a)enqueue() i.e add the list above to the queue.b)dequeue() i.e delete or remove any two elements in the queue in a) above.c)ISfull() i.e Determine if the queue is Full.d)Isempty() i.e Determine if the queue is Empty.e)print() i.e print the elements of the Queue.arrow_forwardListQueue Node Node Node front- next = next next - nul1 data - "Jones" rear data "Thome" data - "Abreu" size = 3 The above is a queue of a waiting list. The ListQueue has a node (front) to record the address of the front element of a queue. It also has another node (rear) to record the address of the tail element of a queue. 3. How do you pop a node from the above queue? a. front.next = front; b. front = front.next с. rear = rear.next d. rear.next = rear Describe the reason of your choice. Your answer is (a, b, c or d) will the pop action take time in 0(1) or 0(n)? Next Page P Type here to searcharrow_forwardPlease don't copy Write a C++ program that uses a linked list implementation The information for each toy product includes the product ID, product name, available quantity and price. Create a linked list node to store the information for each product. Provide a menu to perform the following actions. * Add a new product. Products are sorted according to the product ID in the linked list. * Sell a product. The available quantity of this product is reduced, based on the number of products that have been sold * Check if a product is available * Search if a product is sold by a shop * List the names of productscheaper than 50arrow_forward
- 24If you enqueue 5 elements into an empty queue, and then perform the isEmpty operation 5 times, the queue will be empty again.arrow_forwardMultiple choice in data structures void doo(node<int>*root){ if(root !=0) { node<int>*p=root; while(root->next!=0) root=root->next; p->data=root->data; } What is this code do? a. swap the first item with the last item in the linked list b. set the first item in the linked list as the last item c. doesn't do anything because the root parameter is passed by value d. change the root item in the binary tree with the farthest leaf itemarrow_forwardTOPICS: LIST/STACK/QUEUE Write a complete Java program about Appointment schedule(anything). Your program must implements the linked list The program should have the following basic operations of list, which are: a) Add first, in between and last b) Delete first, in between and last c) Display all data The program should be an interactive program that allow user to choose type of operation.arrow_forward
- C# language Write a program that creates a Queue or Stack (your choice) that represents a list of work orders. This program should use loop, allowing the user to push and pop items on the stack / queue. The program should also allow the user to print all the items in the stack / queue to the console.arrow_forwardLINKED LIST IN PYTHON Create a program using Python for the different operations of a Linked List. Your program will ask the user to choose an operation. 1. Create a List -Ask the user how many nodes he/she wants. -Enter the element/s -Display the list -Back to menu 2. Add at beginning -Ask for the element to be inserted. -Display the list -Back to menu 3. Add after -Ask for the element to be inserted. -Ask for the position AFTER which the element is to be inserted -Display the list -Back to menu 4. Delete -Ask for the element (data) to be deleted -If found, delete the node with that data. -If multiple values, delete only the first element found -If not found, display that the element is not found -back to menu 5. Display -Display the list 6. Count -Display the number of elements 7. Reverse -Reverse the list and display it 8. Search -Ask the user for the element (data) to be searched -Display a message if the element is found or not 9. Quit -Exits the programarrow_forwardCreate a pseudocode for the Queue Operations. The pseudocode should accept N values, checks if the queue is full or empty and display messages indicating if the Queue is empty or full. It should also print the final contents.arrow_forward
- 08. Problem Title: "Add Two Numbers" Problem Description: You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list. You may assume the two numbers do not contain any leading zero, except the number 0 itself.08.arrow_forwardBinary Search Tree Using a binary search tree, you are tasked with building a dictionary program which you can store a word with its definition. Each node of the tree will contain the word and definition. The word is what will be used as the key to sort our data. The dictionary should allow you to search for a word. If the word exist then the definition will display. You can also add words to the dictionary. For testing you should be able to display the current word list. The dictionary will populate from a data file and new words will be saved to the file as well. We are still covering Binary trees in class, but we have covered enough to get you started.arrow_forwardTrue or false: In a queue, elements are both inserted and deleted from one end. True Falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
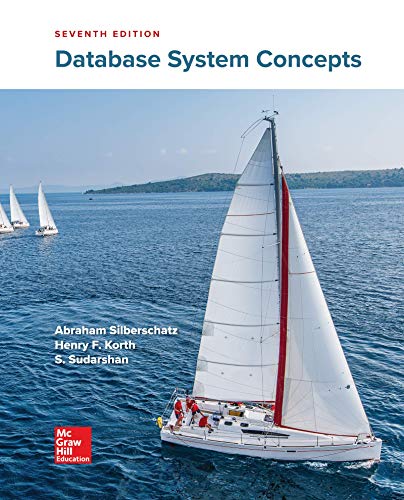
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
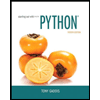
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
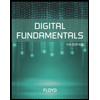
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
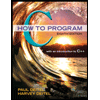
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
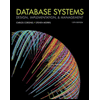
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
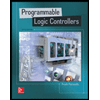
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education