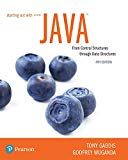
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 6PC
Program Plan Intro
Binary Tree Input and Display
Program plan:
- Import required packages.
- Define the class named “JTreeDemo”.
- Declare required private variables.
- Define the constructor.
- Initialize the values.
- Load the “JTree”.
- Give function definition “readData ()” to read data from the file.
- Create the objects for “FileInputStream”, “InputStreamReader”, and “BufferedReader” in order to read the file
- Declare variables “line”, and “countNode”.
- Inside the “try” block,
- Do until the file becomes empty using “while” condition.
- Check if the line contains the given matches,
-
- Assign the values to “numNodes”.
- Else,
-
- Assign line to “data[countNode]”.
- Increment the value of “countNode”.
- Close the file.
-
-
- Catch the exception using “catch” block and print the exception.
-
-
- Do until the file becomes empty using “while” condition.
- Give function definition to populate the tree.
- Create an object for “DefaultMutableTreeNode”.
- Loop through the number of nodes.
- Split the string.
- Check if the length is greater than 1.
- Add the node to the tree.
- Loop through the length of node.
-
- Add the node to the tree.
- Check if the length is equal to 1.
- Add the node to the tree.
-
-
- Load the nodes to the tree.
-
- Give function definition to create GUI.
- Create a frame and add the tree to the frame.
- Set the close operation and call the function “pack ()”.
- Make the frame visible.
- Give “main ()” function.
- Assign a filename to the variable.
- Create an object for the class.
- Call the function “readData ()”.
- Call the function “populateTree ()”.
- Call the function “createAndShowGUI()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Domino cycledef domino_cycle(tiles):A single domino tile is represented as a two-tuple of its pip values, such as (2,5) or (6,6). This function should determine whether the given list of tiles forms a cycle so that each tile in the list ends with the exact same pip value that its successor tile starts with, the successor of the last tile being the first tile of the list since this is supposed to be a cycle instead of a chain. Return True if the given list of domino tiles form such a cycle, and False otherwise.
tiles
Expected result
[(3, 5), (5, 2), (2, 3)]
True
[(4, 4)]
True
[]
True
[(2, 6)]
False
[(5, 2), (2, 3), (4, 5)]
False
[(4, 3), (3, 1)]
False
struct nodeType {
int infoData;
nodeType * next;
};
nodeType *first;
… and containing the values(see image)
Using a loop to reach the end of the list, write a code segment that deletes all the nodes in the list. Ensure the code performs all memory ‘cleanup’ functions.
Arithmetic progression def arithmetic_progression(items): An arithmetic progression is a numerical sequence so that the stride between each two consecutive elements is constant throughout the sequence. For example, [4, 8, 12, 16, 20] is an arithmetic progression of length 5, starting from the value 4 with a stride of 4. Given a non-empty list items of positive integers in strictly ascending order, find and return the longest arithmetic progression whose all values exist somewhere in that sequence. Return the answer as a tuple (start, stride, n) of the values that define the progression. To ensure unique results to facilitate automated testing, if there exist several progressions of the same length, this function should return the one with the lowest start. If several progressions of equal length emanate from the lowest start, return the progression with the smallest stride.
items
expected results
[42]
(42, 0, 1)
[2, 4, 6, 7, 8, 12, 17]
(2, 2, 4)
[1, 2, 36, 49, 50, 70, 75, 98,…
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- flip_matrix(mat:list)->list You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix. To print the matrix to the console: print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat])) Example: Matrix: W R I T X H D R L G L K F M V G I S T C W N M N F Expected: W N M N F G I S T C L K F M V H D R L G W R I T X Matrix: L C S P Expected: S P L C Matrix: A D J A Q H J C I Expected: J C I A Q H A D Jarrow_forwardData Structurearrow_forwardPython Language - Bad Luck Numbersarrow_forward
- java data structurearrow_forwardWords with given shape def words_with_given_shape(words, shape): The shape of the given word of length n is a list of n - 1 integers, each one either -1, 0 or +1 to indicate whether the next letter following the letter in that position comes later (+1), is the same (0) or comes earlier (-1) in the alphabetical order of English letters. For example, the shape of the word 'hello' is [-1, +1, 0, +1], whereas the shape of 'world' is [-1, +1, -1, -1]. Find and return a list of all words that have that particular shape, listed in alphabetical order. Note that your function, same as all the other functions specified in this document that operate on lists of words, should not itself try to read the wordlist file words_sorted.txt, even when Python makes this possible with just a couple of lines of code. The tester script already reads in the entire wordlist and builds the list of words from there. Your function should use this given list of words without even caring which particular file it…arrow_forwardWords with given shape def words_with_given_shape(words, shape): The shape of the given word of length n is a list of n - 1 integers, each one either -1, 0 or +1 to indicate whether the next letter following the letter in that position comes later (+1), is the same (0) or comes earlier (-1) in the alphabetical order of English letters. For example, the shape of the word 'hello' is [-1, +1, 0, +1], whereas the shape of 'world' is [-1, +1, -1, -1]. Find and return a list of all words that have that particular shape, listed in alphabetical order. Note that your function, same as all the other functions speci0ied in this document that operate on lists of words, should not itself try to read the wordlist 0ile words_sorted.txt, even when Python makes this possible with just a couple of lines of code. The tester script already reads in the entire wordlist and builds the list of words from there. Your function should use this given list of words without even caring which particular 0ile it…arrow_forward
- SKELETON CODE IS PROVIDED ALONG WITH C AND H FILES. #include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdbool.h> #include "node.h" #include "stack_functions.h" #define NUM_VERTICES 10 /** This function takes a pointer to the adjacency matrix of a Graph and the size of this matrix as arguments and prints the matrix */ void print_graph(int * graph, int size); /** This function takes a pointer to the adjacency matrix of a Graph, the size of this matrix, the source and dest node numbers along with the weight or cost of the edge and fills the adjacency matrix accordingly. */ void add_edge(int * graph, int size, int src, int dst, int cost); /** This function takes a pointer to the adjacency matrix of a graph, the size of this matrix, source and destination vertex numbers as inputs and prints out the path from the source vertex to the destination vertex. It also prints the total cost of this…arrow_forwardWord IndexWrite a python program that reads the contents of a text file. The program should create a dictionary inwhich the key-value pairs are described as follows:Key. The keys are the individual words found in the file.576Values. Each value is a list that contains the line numbers in the file where the word (thekey) is found.For example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionarywould contain an element in which the key was the string “robot”, and the value was a listcontaining the numbers 7, 18, 94, and 138.Once the dictionary is built, the program should create another text file, known as a word index,listing the contents of the dictionary. The word index file should contain an alphabetical listingof the words that are stored as keys in the dictionary, along with the line numbers where thewords appear in the original file. Figure 9-1 shows an example of an original text file(Kennedy.txt) and its index file (index.txtarrow_forwardphyton Topics: list and list processing You will write a program to read grade data from a text file, displays the grades as a 5-column table, and then print the statistics (min, max, median, and median). You can assume that the input file only one number per line. You can assume the user always enter a file that exists. The median is the value in the middle of a sorted list. To sort a list, use list.sort() function. It’s computed as below. For a list of odd length, the middle number is just the length divide by 2. For the list, [1,2,3], the median is 2 since 2 is in the middle of the list. The middle index is 3//2, which is 1. Remember that // is the integer division operator. When the length of the list is even, there are two middle numbers. The median is the average of the two middle numbers. For example, [1,2,3,4], the median is (2+3)/2 = 2.5. The two middle indexes for this example are (4//2)-1 = 1 and (4//2) = 2. Functions you need to write: read_grades() Prompts…arrow_forward
- struct node{ int a; struct node * nextptr; }; Write two functions. One for inserting new values to a link list that uses the given node structure. void insert(struct node **head, int value); Second function is called to count the number of even numbers in the link list. It returns an integer that represents the number of even numbers. int countEvenNumbers(struct node *head); Write a C program that reads a number of integers from the user and insert those integers into a link list (use insert function). Later pass the head pointer of this link list to a function called countEvenNumbers. This function counts and returns the number of even numbers in the list. The returned value will be printed on the screen. Note 1: Do not modify the function prototypes. Sample Input1: Sample Output1: 45 23 44 12 37 98 33 35 -1 3 Sample Input2: Sample Output2: 11 33 44 21 22 99 123 122 124 77 -1 4arrow_forwardThe mapped list pattern Our second pattern is the mapped list pattern, described in video 4 3 mapped list pattern. Often we need to write a function that takes a list as a parameter and returns a new list in which each item in the original list is "mapped" to a new item in the result list. For example, the following function takes a list of numbers as a parameter and returns a list of all the numbers squared, e.g. squares ( [1, 3, 7]) returns [1, 9, 49]. def squares (nums): "Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result Although this is just a special case of the accumulator pattern, it is so common that we give it its own name: the mapped list pattern. Consider the following function: def squares(nums): ""Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result If the main program calls print(squares ( [5, -3, 2, 7]) what is the state table for the function…arrow_forwardHAPTER 5: Lists and Dictiona st membership > 51621 O WorkArea Instructions eadline: 03/ 11:59pm EDT Given that k contains an integer and that play_list has been defined to be a list, write a expression that evaluates to True if the value assigned to k is an element of play_list. Additional Notes: play_list and k should not be modifiedarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
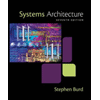
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning