Arithmetic progression def arithmetic_progression(items): An arithmetic progression is a numerical sequence so that the stride between each two consecutive elements is constant throughout the sequence. For example, [4, 8, 12, 16, 20] is an arithmetic progression of length 5, starting from the value 4 with a stride of 4. Given a non-empty list items of positive integers in strictly ascending order, find and return the longest arithmetic progression whose all values exist somewhere in that sequence. Return the answer as a tuple (start, stride, n) of the values that define the progression. To ensure unique results to facilitate automated testing, if there exist several progressions of the same length, this function should return the one with the lowest start. If several progressions of equal length emanate from the lowest start, return the progression with the smallest stride.
Arithmetic progression
def arithmetic_progression(items):
An arithmetic progression is a numerical sequence so that the stride between each two consecutive elements is constant throughout the sequence. For example, [4, 8, 12, 16, 20] is an arithmetic progression of length 5, starting from the value 4 with a stride of 4. Given a non-empty list items of positive integers in strictly ascending order, find and return the
longest arithmetic progression whose all values exist somewhere in that sequence. Return the answer as a tuple (start, stride, n) of the values that define the progression. To ensure unique results to facilitate automated testing, if there exist several progressions of the same length, this function should return the one with the lowest start. If several progressions of equal length emanate from the lowest start, return the progression with the smallest stride.
items | expected results |
[42] | (42, 0, 1) |
[2, 4, 6, 7, 8, 12, 17] | (2, 2, 4) |
[1, 2, 36, 49, 50, 70, 75, 98, 104, 138, 146,148, 172, 206, 221, 240, 274, 294, 367, 440] | (2, 34, 9) |
[2, 3, 7, 20, 25, 26, 28, 30, 32, 34, 36, 41, 53, 57, 73, 89, 94, 103, 105, 121, 137, 181, 186, 268, 278, 355, 370, 442, 462, 529, 554, 616, 646, 703] | (7, 87, 9) |
range(1000000) | (0, 1, 1000000) |

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

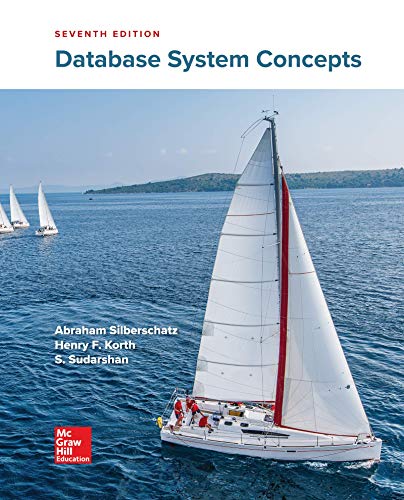
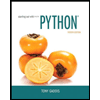
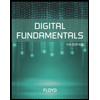
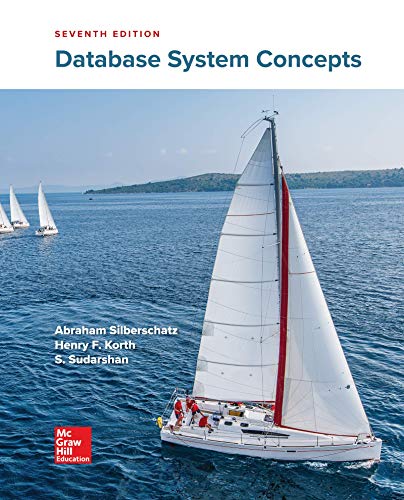
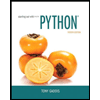
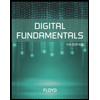
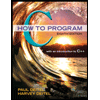
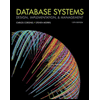
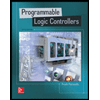