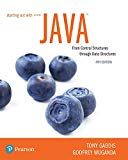
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21, Problem 2AW
Program Plan Intro
Binary tree:
- Binary tree is a hierarchical structure to represent the data. The element of tree is called as a node.
- Here, the branches are used to connect the nodes.
- Each node may have zero, one, or two children.
- A node that does not have a superior node is called the root node.
- The root node is the starting node, and it is the ancestor for all other nodes in the tree.
- A node that does not have any children is called as a leaf node or an end node.
- It is used to implement binary search trees and binary heaps.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
void F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code
Select one:
a.
free the binary tree and returns it empty
b.
Set all data items in the binary tree to 0
c.
remove all items in the binary tree without changing its size
d.
all of them
void F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code
Select one:
a.all of them
b.Set all data items in the binary tree to 0
c.free the binary tree and returns it empty
d.remove all items in the binary tree without changing its size
void F(node<int>&*root){if(root!=0){F(root->left); F(root->right); root->data=0; delete root;}root=0;} this code
Select one:
a.
all of them
b.
Set all data items in the binary tree to 0
c.
free the binary tree and returns it empty
d.
remove all items in the binary tree without changing its size
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21 - Prob. 1MCCh. 21 - Prob. 2MCCh. 21 - Prob. 3MCCh. 21 - Prob. 4MCCh. 21 - Prob. 5MCCh. 21 - Prob. 6MCCh. 21 - Prob. 7MCCh. 21 - Prob. 8MC
Ch. 21 - Prob. 9MCCh. 21 - Prob. 10MCCh. 21 - Prob. 11TFCh. 21 - Prob. 12TFCh. 21 - Prob. 13TFCh. 21 - Prob. 14TFCh. 21 - Prob. 15TFCh. 21 - Prob. 16TFCh. 21 - Prob. 17TFCh. 21 - Prob. 18TFCh. 21 - Prob. 19TFCh. 21 - Prob. 20TFCh. 21 - Prob. 21TFCh. 21 - Prob. 1FTECh. 21 - Prob. 2FTECh. 21 - Prob. 3FTECh. 21 - Prob. 1SACh. 21 - Prob. 2SACh. 21 - Prob. 3SACh. 21 - Prob. 4SACh. 21 - What is a priority queue?Ch. 21 - Prob. 6SACh. 21 - Prob. 7SACh. 21 - Prob. 1AWCh. 21 - Prob. 2AWCh. 21 - Prob. 3AWCh. 21 - Prob. 4AWCh. 21 - Prob. 5AWCh. 21 - Prob. 6AWCh. 21 - Prob. 7AWCh. 21 - Prob. 4PCCh. 21 - Prob. 6PC
Knowledge Booster
Similar questions
- Given the preorder and inorder traversals of a binary tree, you build that binary tree. 5. Preorder: X В G Y E Inorder: Y G В D E Narrow_forward2. Write the code for preorder traversal of a binary tree. struct node{ int data; struct node *left, *right; } void preorder(node *x) { }arrow_forwardTrue or False: The largest element in any non-empty BST always has no right child. True or False: The smallest element in any non-empty BST always has no right child. A tree node that has no children is called a node. The children of a same parent node are called asarrow_forward
- Java - Write pseudocode for the binary tree method isLeaf() that returns true if the node is a leaf and false if not.arrow_forwardWhen inorder traversing a complete binary tree resulted E A C K F H D; the postorder traversal would return Select one: a.E C A F H D K b.E C A F D H K c.E A C F D H K d.E C F A D H Karrow_forwardThe tree pointer is the first node of the binary tree and has both data and two reference links. True Falsearrow_forward
- Implement the binary tree ADT using a linked structure as we developed in class, add the following methods: - def countK(self, num): function that takes in num and counts number of times num appears in the binary tree - def equal(self, other: BinaryTree): function thats takes in another binary tree (other) as parameter and returns true if both binary trees are equal, otherwise function returns False.arrow_forwardIn java: binary tree Write a method called findTotalParents (, this method will find all the nodes that considered to be parents with any dependency and return the total number of parents. The public method shall not take any parameters, but the private method takes a BNode as a parameter, representing the current root.arrow_forwardGiven the preorder and inorder traversals of a binary tree, you build that binary tree. Preorder: X In-order: A Y A E Y EX C C K D D L KLarrow_forward
- The following Program in java implements a BST. The BST node (TNode) contains a data part as well as two links to its right and left children. 1. Draw (using paper and pen) the BST that results from the insertion of the values 60,30, 20, 80, 15, 70, 90, 10, 25, 33 (in this order). These values are used by the program 2. Traverse the tree using preorder, inorder and postorder algorithms (using paper and pen) 3. Write the program in c++, follow its steps, then compile and run. - Compare the results to what you obtained in (2) above. - Try different values class TestBST{public static void main(String []args){int i;int x[] = {60,30, 20, 80, 15, 70, 90, 10, 25, 33};BST t = new BST();for (i=0; i < 10; i++){System.out.print(" Adding: "+x[i]+" to the BST ");t.insert(x[i]);}System.out.println("\nPreorder traversal result:");t.preorder(t.root);System.out.println("\nInorder traversal result:");t.inorder(t.root);System.out.println("\nPostorder traversal result:");t.postorder(t.root);}}class…arrow_forwardint doo(node*root){ if(root !=0 ) { doo(root->left); doo(root->right); return root->data; }} this code used for O a. find the root value of the binary tree if it has items O b. print the items in the binary tree if it has items c. find the farthest left item in the binary tree if it has items O d. find the farthest right item in the binary tree if it has itemsarrow_forwardAdd the following new methods in BST./** Display the nodes in a breadth-first traversal */public void breadthFirstTraversal()/** Return the height of this binary tree */public int height()arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
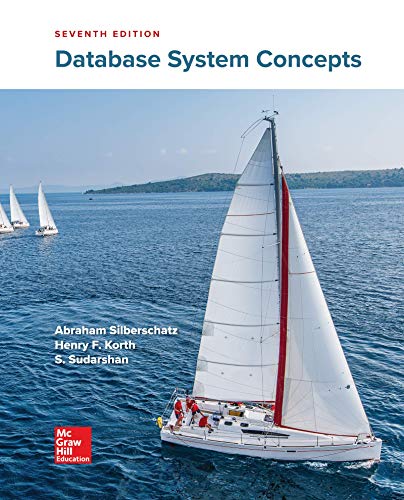
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
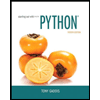
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
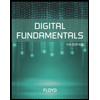
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
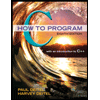
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
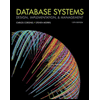
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
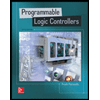
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education