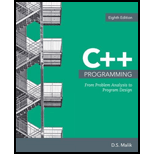
Concept explainers
Explanation of Solution
Below, the program execution and the output (if applicable) for relevant blocks of code are explained:
#include <iostream>
#include <string>
using namespace std;
const double CONVERSION = 3.5;
Explanation:
In the above lines of code, the constant CONVERSION is declared and initialized.
int main() {
const int TEMP = 23;
string name;
int id;
int num;
double decNum;
double mysteryNum;
Explanation:
In the above lines, inside the main function, the declaration and initialization of the constant TEMP is followed by the declaration of the variables name, id, num, decNum and mysteryNum.
cout << "Enter last name: ";
Explanation:
The stream insertion operator << and cout is used to print a string "Enter last name: ". The insertion point remains in the same line as the string output on the console. So the output is:
Enter last name:
cin >> name;
Explanation:
The stream extraction operator >> and cin is used to accept the user input of a string ("Miller") which are assigned to the variable name. The insertion point remains in the same line as the string which was typed as input at the console.
cout << endl;
Explanation:
The stream insertion operator cout is used to move the insertion point to a new line through the use of the manipulator endl.
cout << "Enter a two digit integer: ";
Explanation:
The stream insertion operator << and cout are used to print a string "Enter a two digit integer: ". The insertion point remains in the same line as the string output on the console. So the output is,
Enter a two digit integer:
cin >> id;
Explanation:
The stream extraction operator >> and cin are used to accept the user input of a two-digit number (34) which is assigned to the variable id. The insertion point remains in the same line as the string which was typed as input at the console.
cout << endl;
Explanation:
The stream insertion operator << and cout are used to move the insertion point to a new line through the use of the manipulator endl.
num = (id * TEMP) % (static_cast<int>(CONVERSION));
Explanation:
The assignment statement assigns a new value to the variable num after evaluating the right-hand-side arithmetic expression. The expression is evaluated as follows,
(id * TEMP) % (static_cast<int>(CONVERSION))
= (id * TEMP) % (static_cast<int>(CONVERSION))
= (34 * 23) % (static_cast<int>(3.5)) (value substitution)
= (34 * 23) % (3) (static_cast operator converts the floating point
number into int type discarding the decimal portion)
= 782 % 3 (parentheses has highest precedence, * operator
evaluated)
= 2 (modulus operator - 782 / 3 leaves 2 as remainder)
cout << "Enter a decimal number: ";
Explanation:
The stream insertion operator cout is used to print a string "Enter a decimal number: "...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- PROGRAMMING LANGUAGE: C++ Add screenshots of outputs as well Execute the following example and write a comment after each line of code. In result print output of code. #include<iostream> #include<string> using namespace std; class Date {public: Date() {month=1; day=1; year=1990; } Date(int m,int d, int y) { month=m; day=d; year=y; } void set() { int d,m,y; cout<<"Enter day: "; cin>>d; cout<<"Enter month: "; cin>>m; cout<<"Enter year: "; cin>>y; month=m; day=d; year=y; } void print() { cout<<day<<"-"<<month<<"-"<<year; } private:…arrow_forward#include using namespace std; bool Facto(int* pSquared, int* pCubed, int n); int main() { int number, squared, cubed; bool status; cout « "Enter a number (0 - 10): "; cin >> number; /* a. Write the statement to call function Facto() */ if (status) { cout <« "Number: " << number << end1; cout <« "Square: cout <« "Cube: " <« cubed « endl; } " « squared « endl; else cout « "Error encountered!\n"; cout <« endl « endl; bool Facto(int* pSquared, int* pCubed, int n) bool value; /* b. Write the statements in the function body */ return value;arrow_forward#include using namespace std; void myfunction(int num2, int num1); lint main() { my function (5,2); return 0; } void myfunction(int num1, int num2) {if (num1>3) cout << "A1"; else if (num1<3) cout<<"A2"; else cout<<"A3";} O A2 O A1 O A3 A1 A2 A3arrow_forward
- (a) #include using namespace std; int main() { } for(int i = 0; i <=30; cout << i*2 << endl; } return 0; ){ Output: 0 20 40 60arrow_forward#include using namespace std; int main() int x=1,y=2; for (int i=0; i<3; i++) e{ x=x*y; 8{ } cout<arrow_forwardmain.c pattern2.h 3. 333221(from codeChum, modified) #include #include"pattern2.h" int main(void) { int num = getNum(); display(num); return 0; 2 by Catherine Arellano 3 4 Make a C program that will output the figure below. 5 Implement the following functions: 国 7 int getNum(); *accepts input from the user.*/ void display(int num); /*prints the number pattern*/ Note: 1. Use only while loop. 2. You are not allowed to edit main.c and pattern2.h. Input A single line containing the number of rowsarrow_forwardSORU 3. (QUESTION 3.) 1 #include using namespaces std; intmain() 4日{ flaot a=6, b=8,c=4, d=-11;M=78,x,y,z,t; x=(M-(a+b/4)+c-d); y=(++a *b/8); z=++y*c; t=(c-d)/a*++b-0.2857; 6. 7 8. 9. 10 11 } ---.--- I.- -.? What is the number of errors made in the program? -+n sa SI (Number of Errors) : P. . y Jlur? Bosluklara vazını What will be the final values of the following variables (x,y,z,t) when the program is run? Write it in the blanks. y%3Darrow_forwardC++ Figure 6.9 #include <iostream>#include <cstdlib> // contains prototypes for functions srand and rand#include <ctime> // contains prototype for function timeusing namespace std; unsigned int rollDice(); // rolls dice, calculates and displays sum int main() {// scoped enumeration with constants that represent the game status enum class Status {CONTINUE, WON, LOST}; // all caps in constants // randomize random number generator using current timesrand(static_cast<unsigned int>(time(0))); unsigned int myPoint{0}; // point if no win or loss on first rollStatus gameStatus; // can be CONTINUE, WON or LOSTunsigned int sumOfDice{rollDice()}; // first roll of the dice // determine game status and point (if needed) based on first rollswitch (sumOfDice) {case 7: // win with 7 on first rollcase 11: // win with 11 on first roll gameStatus = Status::WON;break;case 2: // lose with 2 on first rollcase 3: // lose with 3 on first rollcase 12: // lose with 12 on first roll…arrow_forwardint func(int a, int b) { return (aarrow_forwardt) Write a one-liner JAVA function that takes a string s and an integer i as the parameters and removes the character at index i from the string s and returns the string. public static String deleteCharAt_i(String s, int i){ //write your one-line code here }arrow_forward#include using namespace std; int main() { int x,y; or (x=0 ;x 3) break ; cout << y << endl; | }}} O 5 9. O 4 O 10arrow_forward#include using namespace std; bool isPalindrome(int x) { int n=0,val; val = x; while(x > 0) { n = n * 10 + x % 10; x = x / 10; } } int main() { int n; cin >>n; if(isPalindrome(n)) { cout <arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_iosRecommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr