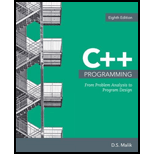
Explanation of Solution
Below, the program execution and the output (if applicable) for relevant blocks of code are explained.
#include <iostream>
using namespace std;
const int NUM = 10;
const double X = 20.5;
int main() {
int firstNum, secondNum;
double z;
char grade;
firstNum = 62;
Explanation:
In the above lines of code, the constants NUM and X are declared and initialized, followed by the declaration of the variables firstNum, secondNum, z, and grade. The variable firstNum is also assigned a value of 62 through an assignment statement.
cout << "firstNum = " << firstNum << endl;
Explanation:
The stream insertion operator << and cout are used to print a string "firstName = " and the value of the firstNum variable which is 62 at this point of execution. The insertion point is finally set to a new line through the use of the manipulator endl. So the output is,
firstNum = 62
cout << "Enter three numbers: ";
Explanation:
The stream insertion operator << and cout are used to print a string "Enter three numbers: ". The insertion point remains in the same line as the string output on the console. So the output is,
Enter three numbers:
cin >> firstNum >> z >> secondNum;
Explanation:
The stream extraction operator >> and cin are used to accept the user inputs of three numbers (35, 10.5 and 27) which are assigned respectively to the variables firstNum, z, and secondNum. The insertion point remains in the same line as the numbers which were typed as input at the console.
cout << endl;
Explanation:
The stream insertion operator << cout is used to move the insertion point to a new line through the use of the manipulator endl.
cout << "The numbers you entered are "
<< firstNum << ", " << z << ", and "
<< secondNum << endl;
Explanation:
The above three lines of code are read as a single line of code by the compiler. The stream insertion operator << and cout are used to print a string "The numbers you entered are " followed by the value of the firstNum variable (which has a value assigned to 35), another string ", " followed by the value of variable z (which has a value assigned to 10.5). This in turn is followed by a string ", and " and the value of the variable secondNum (which has a value assigned to 27) at this point of execution. The insertion point is finally set to a new line through the use of the manipulator endl. So the output is,
The numbers you entered are 35, 10.5, and 27
z = z - X + 2 * firstNum - secondNum;
Explanation:
The assignment statement assigns a new value to the variable z after evaluating the right-hand-side arithmetic expression. The expression is evaluated as follows,
z - X + 2 * firstNum - secondNum
= 10.5 - 20.5 + 2 * 35 - 27 (value substitution)
= 10.5 - 20.5 + (2 * 35) - 27 (* operation has higher precedence than the other operators)
= -10.0 + (2 * 35) - 27 (expression evaluated left to right)
= -10.0 + 70 - 27 (integer multiplication)
= -10.0 + 70.0 - 27 (+ operator with mixed operands - convert to floating point)
= 60.0 - 27 (floating point addition)
= 60.0 - 27.0 (- operator with mixed operands - convert to floating point)
= 33.0 (floating-point subtraction)
cout << "z = " << z << endl;
Explanation:
The stream insertion operator << and cout are used to print a string "z = " followed by the value of z which is 33.0 at this point of execution. However, cout prints it as 33 due to lack of formatting expressions. The insertion point is set to a new line through the use of the manipulator endl. So the output is,
z = 33
cout << "Enter grade: ";
Explanation:
The stream insertion operator << and cout are used to print a string "Enter grade: ". The insertion point remains in the same line as the string output on the console. So the output is,
Enter grade:
cin >> grade;
Explanation:
The stream extraction operator >> and cin are used to accept the user input of a letter ('B') which are assigned respectively to the variable grade. The insertion point remains in the same line as the letter which was typed as input at the console...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
C++ Programming: From Problem Analysis to Program Design
- (Conversion) a. Write a C++ program to convert meters to feet. The program should request the starting meter value, the number of conversions to be made, and the increment between metric values. The display should have appropriate headings and list the meters and the corresponding feet value. If the number of iterations is greater than 10, have your program substitute a default increment of 10. Use the relationship that 1 meter = 3.281 feet. b. Run the program written in Exercise 6a on a computer. Verify that your program begins at the correct starting meter value and contains the exact number of conversions specified in your input data. c. Modify the program written in Exercise 6a to request the starting meter value, the ending meter value, and the increment. Instead of the condition checking for a fixed count, the condition checks for the ending meter value. If the number of iterations is greater than 20, have your program substitute a default increment of (ending value - starting value) / 19.arrow_forward## Q3. Finding the largest number from three numbers. **C++ #include using namespace std; int findLargest3(int a, int b, int c) { int largest; // Put your code here return largest; int main() { int a, b, c; cout > a; cin >> b; cin >> c; cout << "The largest number is " <« fingLargest3(a, b, c) « endl; return 1; *Hint 1: Considering the case that the two or three numbers are equal.*arrow_forwardSORU 3. (QUESTION 3.) 1 #include using namespaces std; intmain() 4日{ flaot a=6, b=8,c=4, d=-11;M=78,x,y,z,t; x=(M-(a+b/4)+c-d); y=(++a *b/8); z=++y*c; t=(c-d)/a*++b-0.2857; 6. 7 8. 9. 10 11 } ---.--- I.- -.? What is the number of errors made in the program? -+n sa SI (Number of Errors) : P. . y Jlur? Bosluklara vazını What will be the final values of the following variables (x,y,z,t) when the program is run? Write it in the blanks. y%3Darrow_forward
- PROGRAMMING LANGUAGE: C++ Add screenshots of outputs as well Execute the following example and write a comment after each line of code. In result print output of code. #include<iostream> #include<string> using namespace std; class Date {public: Date() {month=1; day=1; year=1990; } Date(int m,int d, int y) { month=m; day=d; year=y; } void set() { int d,m,y; cout<<"Enter day: "; cin>>d; cout<<"Enter month: "; cin>>m; cout<<"Enter year: "; cin>>y; month=m; day=d; year=y; } void print() { cout<<day<<"-"<<month<<"-"<<year; } private:…arrow_forward#include // Function to calculate the factorial of a given positive integer int factorial(int n) { // TODO: Implement the factorial function here } int main() { int num; printf("Enter a positive integer: "); scanf("%d", &num); // TODO: Call the factorial function and print the result } return 0; • Q1: Write a C program to calculate the factorial of a given positive integer entered by the user.arrow_forwardint x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forward
- #include using namespace std; int main() int x=1,y=2; for (int i=0; i<3; i++) e{ x=x*y; 8{ } cout<arrow_forwarda) Given the following codes int x = 0; int *y = while(x < 10) { int z = 0; 1: 2: 3: 4: 5: 6: 7: malloc(sizeof(int)); int y = x; while (y } < 10) { z = z + y; y = y + 1; 8: 9: 10: } 11: *y = x; Write the symbol table indicating the type of variables in line 4arrow_forward#include<stdio.h> #include<stdarg.h> void fun1(int num, ...); void fun2(int num, ...); int main() { fun1(1, "Apple", "Boys", "Cats", "Dogs"); fun2(2, 12, 13, 14); return 0; } void fun1(int num, ...) { char *str; va_list ptr; va_start(ptr, num); str = va_arg(ptr, char *); printf("%s ", str); } void fun2(int num, ...) { va_list ptr; va_start(ptr, num); num = va_arg(ptr, int); printf("%d", num); }.arrow_forwardC++ Coding: Arrays Given the following code and program output: #include <iostream>using namespace std;double average(const int g[], const int& CAP);void output(const int *g, const int& GRADES, const double& GPA);int main() { const int NUM_GRADES = 5; int grades[NUM_GRADES] = {10, 15, 20, 25, 30}; //average function is called by output function output(grades, NUM_GRADES, average(grades, NUM_GRADES)); return 0;} Example Output: 10, 15, 20, 25, 30GPA: 20 Code function definitions for average() and output(): average() accepts the array and its size as input, and returns the average grade with two decimal places. output() accepts the original array, the array's size, and GPA (generated from the average function) as function parameters. Display the array and GPA.arrow_forward// // main.c // Assignment1 // // Created by Hassan omer on 15/10/21. // #include <stdio.h> # include <stdlib.h> int input(); int multiples(); int cions(); void display_change(); int main() { int num; num = input(); multiples(num); cions(); display_change(); return 0; } int input(int num) { printf("enter 5-95 number\n"); scanf("%d",&num); return num; } int multiples(int num) { int sum5 =0; if (sum5 %5 != 0 ||sum5<5|| sum5 >95) { printf("invaild input %d",sum5); } cions(sum5); return sum5; } int cions(int sum05){ int cent50 = 0; int cent20 = 0; int cent10 = 0; int cent05 = 0; if (sum05 > 0) { if (sum05 >= 50){ sum05 -= 50; cent50++; } else if (sum05 >=20){ sum05 -= 20; cent20++; } else if (sum05 >= 10){ sum05 -=10;…arrow_forward#include using namespace std; bool Facto(int* pSquared, int* pCubed, int n); int main() { int number, squared, cubed; bool status; cout « "Enter a number (0 - 10): "; cin >> number; /* a. Write the statement to call function Facto() */ if (status) { cout <« "Number: " << number << end1; cout <« "Square: cout <« "Cube: " <« cubed « endl; } " « squared « endl; else cout « "Error encountered!\n"; cout <« endl « endl; bool Facto(int* pSquared, int* pCubed, int n) bool value; /* b. Write the statements in the function body */ return value;arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
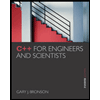