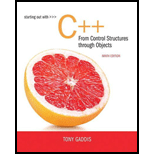
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 2PC
Program Plan Intro
Dynamic Stack Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “dstack” for stack.
- Declare a variable named “popElem”.
- Push 3 elements inside the stack using the function “push ()”.
- Pop 3 elements from the stack using the function “pop ()”.
DynStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack
- Create an object for the template
- Create a stack pointer name “next”.
- Create a stack pointer name “top”
- Give structure declaration for the stack
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty ()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Give function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Give function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top most node and make temp as the top node.
- Give function definition for “isEmpty ()”.
- Assign Boolean value to the variable
- Check if the top node is null
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow
SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS
int main(int argc, char** argv) {
SLLStack* stack = new SLLStack();
int test;
int length;
string str;
char top;
bool flag = true;
cin >> test;
switch (test) {
case 0:
getline(cin, str);
length = str.length();
for(int i = 0; i < length; i++){
if(str[i] == '{' || str[i] == '(' || str[i] == '['){
stack->push(str[i]);
} else if (str[i] == '}' || str[i] == ')' || str[i] == ']'){
if(!stack->isEmpty()){
top = stack->top();
if(top == '{' && str[i] == '}' || top == '(' && str[i] == ')' ||…
Stack Implementation in C++make code for an application that uses the StackX class to create a stack.includes a brief main() code to test this class.
Describe stack segment.
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Multiple choice in data structures Assume the function: void F(stack<T> &S){ } and we send a stack S to the function F, as a result of it Select one: a. Both (copy constructor and destructor) should not be called b. Destructor should be called c. Copy constructor should be called d. Both (copy constructor and destructor) should be calledarrow_forwardC++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow #include "stack.h" #include "linkedlist.h" // SLLStack means Singly Linked List (SLL) Stack class SLLStack : public Stack { LinkedList* list; public: SLLStack() { list = new LinkedList(); } void push(char e) { list->add(e); return; } char pop() { char elem; elem = list->removeTail(); return elem; } char top() { char elem; elem = list->get(size()); return elem; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } };arrow_forwardCode example of stack winding and unwinding in c#.arrow_forward
- Fundamental Data Structures: T1: Stack Implementation In this task, you will implement a stack data structure in Python.Create a Python class named Stack that will have the following methods:__init__(self): Initialize an empty stack.push(self, item): Push an item onto the stack.pop(self): Pop and return the top item from the stack. If the stack is empty, return an appropriate message.peek(self): Return the top item from the stack without removing it. If the stack is empty, return an appropriate message.is_empty(self): Check if the stack is empty and return True or False.size(self): Return the number of elements in the stack.T 2: Using the Stack In this task, you will use the stack you implemented to solve a common problem: checking if a string containing parentheses, brackets, and curly braces is balanced.Write a Python function is_balanced(expression) that takes a string containing parentheses, brackets, and curly braces and returns True if the symbols are balanced and False…arrow_forwardSubject:DATA STRUCTURE Q:Give reason of choosing a list over an array when implementing a stack data structurearrow_forwardc++ data structures ADT Unsorted List The specifications for the Unsorted List ADT states that the item to be deleted is in the list. Rewrite the specifications for DeleteItem so that the list is unchanged if the item to be deleted is not in the list. Rewrite the specifications for DeleteItem so that all copies of the item to be deleted are removed if they existarrow_forward
- 1. a) Write a method in the Stack Class, implemented as an array public String toString() that can be used to display the objects of a Stack in the stack order without changing the stack. (You may assume that an Object.tośtring() method exists.) public class Stack { private Object[] data; int top; b) Write a recursive method in the Stack Class, implemented as a linked structure public String toString() that can be used to display the objects of a Stack in stack order without changing the stack. (You may assume that an Object.toString() method exists.) public class Stack { private node top; } class node{ Object data; node next;}arrow_forwardBriefly explain extended stack pointerarrow_forwardC++ Data Structures Write a program to implement two stacks using linked lists. User will push values on first stack, when anelement is popped from first stack it should automatically push to second stack. From second stack user willpop this element explicitlyarrow_forward
- What exactly is a stack pointer?arrow_forwardAssume an ADT Stack which consists of the following attributes: struct StackNode { int item; StackNode *next; }; StackNode *topPtr; Write the C++ implementation for method displayStack( ) that displays the stack content from top to bottom. Use below method header: void Stack::displayStack ()arrow_forwardBriefly explain stack parameterarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
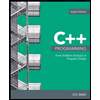
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning