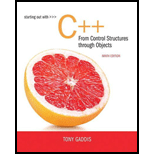
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17.3, Problem 17.19CP
Program Plan Intro
A vector is a container which performs like an extensible array.
- The size of the vector is dynamic.
- The series of elements are stored in the same variable name.
- It uses random-access iterator.
- If an element is added or removed, it adjusts its size automatically, which accommodates the number of elements contained in the vector.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Assignment Instructions:
You are tasked with developing a program to use city data from an online database and generate a
city details report.
1) Create a new Project in Eclipse called "HW7".
2) Create a class "City.java" in the project and implement the UML diagram shown below and add
comments to your program.
3) The logic for the method "getCityCategory" of City Class is below:
a. If the population of a city is greater than 10000000, then the method returns "MEGA"
b. If the population of a city is greater than 1000000 and less than 10000000, then the
method returns "LARGE"
c. If the population of a city is greater than 100000 and less than 1000000, then the method
returns "MEDIUM"
d. If the population of a city is below 100000, then the method returns "SMALL"
4) You should create another new Java program inside the project. Name the program as
"xxxx_program.java”, where xxxx is your Kean username.
3) Implement the following methods inside the xxxx_program program
The main method…
CPS 2231 - Computer Programming – Spring 2025
City Report Application
-
Due Date:
Concepts: Classes and Objects, Reading from a file and generating report
Point value: 40 points.
The purpose of this project is to give students exposure to object-oriented design and programming
using classes in a realistic application that involves arrays of objects and generating reports.
Assignment Instructions:
You are tasked with developing a program to use city data from an online database and generate a
city details report.
1) Create a new Project in Eclipse called "HW7”.
2) Create a class "City.java" in the project and implement the UML diagram shown below and add
comments to your program.
3) The logic for the method "getCityCategory" of City Class is below:
a. If the population of a city is greater than 10000000, then the method returns "MEGA"
b. If the population of a city is greater than 1000000 and less than 10000000, then the
method returns "LARGE"
c. If the population of a city is greater…
Please calculate the average best-case IPC attainable on this code with a 2-wide, in-order, superscalar machine:
ADD X1, X2, X3
SUB X3, X1, 0x100
ORR X9, X10, X11
ADD X11, X3, X2
SUB X9, X1, X3
ADD X1, X2, X3
AND X3, X1, X9
ORR X1, X11, X9
SUB X13, X14, X15
ADD X16, X13, X14
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 17.2 - Prob. 17.1CPCh. 17.2 - Prob. 17.2CPCh. 17.2 - Prob. 17.3CPCh. 17.2 - Suppose you are writing a program that uses the...Ch. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - What does a containers begin() and end() member...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17.3 - Write a statement that defines an empty vector...Ch. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Write a statement that defines a vector object...Ch. 17.3 - What happens when you use an invalid index with...Ch. 17.3 - Prob. 17.16CPCh. 17.3 - If your program will be added a lot of objects to...Ch. 17.3 - Prob. 17.18CPCh. 17.3 - Prob. 17.19CPCh. 17.4 - Prob. 17.20CPCh. 17.4 - Write a statement that defines a nap named myMap....Ch. 17.4 - Prob. 17.22CPCh. 17.4 - Prob. 17.23CPCh. 17.4 - Prob. 17.24CPCh. 17.4 - Prob. 17.25CPCh. 17.4 - Prob. 17.26CPCh. 17.4 - Prob. 17.27CPCh. 17.5 - What are two differences between a set and a...Ch. 17.5 - Write a statement that defines an empty set object...Ch. 17.5 - Prob. 17.30CPCh. 17.5 - Prob. 17.31CPCh. 17.5 - Prob. 17.32CPCh. 17.5 - If you store objects of a class that you have...Ch. 17.5 - Prob. 17.34CPCh. 17.5 - Prob. 17.35CPCh. 17.6 - Prob. 17.36CPCh. 17.6 - What value will be stored in v[0] after the...Ch. 17.6 - Prob. 17.38CPCh. 17.6 - Prob. 17.39CPCh. 17.6 - Prob. 17.40CPCh. 17.6 - Prob. 17.41CPCh. 17.6 - Prob. 17.42CPCh. 17.7 - Prob. 17.43CPCh. 17.7 - Which operator must be overloaded in a class...Ch. 17.7 - Prob. 17.45CPCh. 17.7 - What is a predicate?Ch. 17.7 - Prob. 17.47CPCh. 17.7 - Prob. 17.48CPCh. 17.7 - Prob. 17.49CPCh. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - How does the behavior of the equal_range() member...Ch. 17 - Prob. 12RQECh. 17 - When using one of the STL algorithm function...Ch. 17 - You have written a class, and you plan to store...Ch. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - A(n) ___________ container stores its data in a...Ch. 17 - _____________ are pointer-like objects used to...Ch. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - The _____ class is an associative container that...Ch. 17 - Prob. 27RQECh. 17 - Prob. 28RQECh. 17 - A _______ object is an object that can be called,...Ch. 17 - A _________ is a function or function object that...Ch. 17 - A ____________ is a predicate that takes one...Ch. 17 - A __________ is a predicate that takes two...Ch. 17 - A __________ is a compact way of creating a...Ch. 17 - T F The array class is a fixed-size container.Ch. 17 - T F The vector class is a fixed-size container.Ch. 17 - T F You use the operator to dereference an...Ch. 17 - T F You can use the ++ operator to increment an...Ch. 17 - Prob. 38RQECh. 17 - Prob. 39RQECh. 17 - T F You do not have to declare the size of a...Ch. 17 - T F A vector uses an array internally to store its...Ch. 17 - Prob. 42RQECh. 17 - T F You can store duplicate keys in a map...Ch. 17 - T F The multimap classs erase() member function...Ch. 17 - Prob. 45RQECh. 17 - Prob. 46RQECh. 17 - Prob. 47RQECh. 17 - Prob. 48RQECh. 17 - T F If two iterators denote a range of elements...Ch. 17 - T F You must sort a range of elements before...Ch. 17 - T F Any class that will be used to create function...Ch. 17 - T F Writing a lambda expression usually requires...Ch. 17 - T F You can assign a lambda expression to a...Ch. 17 - Prob. 54RQECh. 17 - Write a statement that defines an iterator that...Ch. 17 - Prob. 56RQECh. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 58RQECh. 17 - Prob. 59RQECh. 17 - The following code defines a vector and an...Ch. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 62RQECh. 17 - Prob. 63RQECh. 17 - Prob. 64RQECh. 17 - Look at the following vector definition: vectorint...Ch. 17 - Write a declaration for a class named Display. The...Ch. 17 - Write code that docs the following: Uses a lambda...Ch. 17 - // This code has an error. arrayint, 5 a; a[5] =...Ch. 17 - // This code has an error. vectorstring strv =...Ch. 17 - // This code has an error. vectorint numbers(10);...Ch. 17 - // This code has an error. vectorint numbers ={1,...Ch. 17 - Prob. 72RQECh. 17 - Prob. 73RQECh. 17 - // This code has an error. vectorint v = {6, 5, 4,...Ch. 17 - // This code has an error. auto sum = ()[int a,...Ch. 17 - Unique Words Write a program that opens a...Ch. 17 - Course Information Write a program that creates a...Ch. 17 - Prob. 3PCCh. 17 - File Encryption and Decryption Write a program...Ch. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Outline the overall steps for configuring and securing Linux servers Consider and describe how a mixed Operating System environment will affect what you have to do to protect the company assets Describe at least three technologies that will help to protect CIA of data on Linux systemsarrow_forwardNode.js, Express, Nunjucks, MongoDB, and Mongoose There are a couple of programs similar to this assignment given in the lecture notes for the week that discusses CRUD operations. Specifically, the Admin example and the CIT301 example both have index.js code and nunjucks code similar to this assignment. You may find some of the other example programs useful as well. It would ultimately save you time if you have already studied these programs before giving this assignment a shot. Either way, hopefully you'll start early and you've kept to the schedule in terms of reading the lecture notes. You will need to create a database named travel using compass, then create a collection named trips. Use these names; your code must work with my database. The trips documents should then be imported unto the trips collection by importing the JSON file containing all the data as linked below. The file itself is named trips.json, and is available on the course website in the same folder as this…arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardusing r languagearrow_forwardWrite a short paper (1 page/about 500 words) summarizing what we as System Admins can do to protect the CIA of our servers. Outline the overall steps for configuring and securing Linux servers Consider and describe how a mixed Operating System environment will affect what you have to do to protect the company assets Describe at least three technologies that will help to protect CIA of data on Linux systems Required Resourcesarrow_forward
- using r language Estimate the MSE of the level k trimmed means for random samples of size 20 generated from a standard Cauchy distribution. (The target parameter θis the center or median; the expected value does not exist.) Summarize the estimates of MSE in a table for k= 1,2,...,9arrow_forwardusing r language Estimate the MSE of the level k trimmed means for random samples of size 20 generated from a standard Cauchy distribution. (The target parameter θis the center or median; the expected value does not exist.) Summarize the estimates of MSE in a table for k= 1,2,...,9arrow_forwardusing r language The data law82 in bootstrap library contains LSAT and GPA for 82 law schools. Compute a 95% bootstrap t confidence interval estimates for the correlation statisticsarrow_forward
- using r language The data law82 in bootstrap library contains LSAT and GPA for 82 law schools. Compute a 95% bootstrap t confidence interval estimates for the correlation statisticsarrow_forwardusing r langauge The data law82 in bootstrap library contains LSAT and GPA for 82 law schools. Compute and compare the three 95% bootstrap confidence interval estimates for the correlation statistics.arrow_forwardWhat is the number of derangements of size k from the set {1,2,...n} to the set {1,2,...n} so that f(x) != x exactly k times with 1 <= k <= narrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
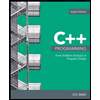
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
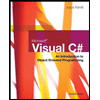
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
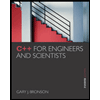
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
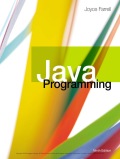
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
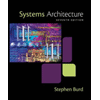
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
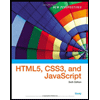
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning