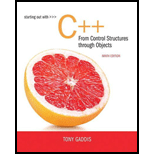
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 5PC
Program Plan Intro
Text File Analysis
Program plan
source.cpp
- Include the required header files to the program.
- Define the main() function,
- Include the required function prototype to the program
- Declare the required variables.
- Create sets to hold each files words.
- Read the first file name from the user.
- Read the second file name from the user.
- Build the sets for two files.
- Display the unique words in both files using “showUnion()” function.
- Make a call to the function “showUnion()”.
- Display only the words that appear in both files using “ShowIntersection()” function.
- Make a call to the function “ShowIntersection ()”.
- Display the words that appear in file #1 but not in file #2 using “showDifference()” function.
- Make a call to the function “showDifference ()”.
- Display the words that appear in only one file, but not in both the files using “showSymDiff()”.
- Function definition for the “SetFile ()”:
- Declare a variable “in” to hold the input.
- Create an object for “ifstream”.
- Open the file using “open()”.
- Loop till “infile” reaches end of the file
- Insert the word to the “setWord”.
- Close the file using “close()”.
- Function definition for the “showUnion()” to display the union of words from two sets:
- Create a
vector to hold the union. - Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Create a
- Function definition for the “showIntersection ()” to display the intersection of words from two sets:
- Create a vector to hold the intersection.
- Get the intersection of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Function definition for the “showDifference ()” to show the difference of two sets:
- Create a vector to hold the difference of two sets.
- Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
- Function definition for the “showSymDiff ()” to show the symmetric difference of two sets:
- Create a vector to hold the symmetric difference of two sets.
- Get the union of the sets using the “begin()” and “end()”.
- Remove the unused words using “resize()” function.
- Display the result.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
JAVA INTRO
The answer last time was incorrect It did not use text files!!!!.
Create a program that generates a report that displays a list of students, classes they are enrolled in and the professor who teaches the class.
There are 3 files that provide the input data:
1. FinalRoster.txt
List of students and professors ( The first value of each row indicates if it is a student or professor; S means a student , P means a professor)
Student and Professor have different data
Student row: "S",Student Name, StudentID
Professor row: "P", Professor Name, Professor ID, Highest Education
2. FinalClassList.txt
List of classes and professor who teach them
Each row contains the following information: ClassID, ClassName, ID of Professor who teach that class
The professor ID in this file matches the Professor ID in FinalRoster.txt.
3. FinalStudentClassList.txt
List of classes the students are enrolled in. (StudentID, ClassID)
Student ID matches Student ID in FinalRoster.txt and ClassID…
GirlNames.txt This file contains a list of the 200 most popular names given to girls born in the United States from the year 2000 through 2009.
BoyNames.txt This file contains a list of the 200 most popular names given to boys born in the United States from the year 2000 through 2009.
Write a program that reads the contents of the two files into two separate lists. The user should be able to enter a boy’s name, a girl’s name, or both, and the application will display messages indicating whether the names were among the most popular.
**programming language is python**
** must use a def main(): function**
*please include some comments*
i aslo have the .txt files but no wya to upload them
C++
Create a program that reads a file containing a list of songs and prints the songs to the screen one at a time. After each song is printed, except for the last song, the program asks the user to press enter for more.After the last song, the program should say that this was the last song and quit.
Chapter 17 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 17.2 - Prob. 17.1CPCh. 17.2 - Prob. 17.2CPCh. 17.2 - Prob. 17.3CPCh. 17.2 - Suppose you are writing a program that uses the...Ch. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - What does a containers begin() and end() member...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17.3 - Write a statement that defines an empty vector...Ch. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Write a statement that defines a vector object...Ch. 17.3 - What happens when you use an invalid index with...Ch. 17.3 - Prob. 17.16CPCh. 17.3 - If your program will be added a lot of objects to...Ch. 17.3 - Prob. 17.18CPCh. 17.3 - Prob. 17.19CPCh. 17.4 - Prob. 17.20CPCh. 17.4 - Write a statement that defines a nap named myMap....Ch. 17.4 - Prob. 17.22CPCh. 17.4 - Prob. 17.23CPCh. 17.4 - Prob. 17.24CPCh. 17.4 - Prob. 17.25CPCh. 17.4 - Prob. 17.26CPCh. 17.4 - Prob. 17.27CPCh. 17.5 - What are two differences between a set and a...Ch. 17.5 - Write a statement that defines an empty set object...Ch. 17.5 - Prob. 17.30CPCh. 17.5 - Prob. 17.31CPCh. 17.5 - Prob. 17.32CPCh. 17.5 - If you store objects of a class that you have...Ch. 17.5 - Prob. 17.34CPCh. 17.5 - Prob. 17.35CPCh. 17.6 - Prob. 17.36CPCh. 17.6 - What value will be stored in v[0] after the...Ch. 17.6 - Prob. 17.38CPCh. 17.6 - Prob. 17.39CPCh. 17.6 - Prob. 17.40CPCh. 17.6 - Prob. 17.41CPCh. 17.6 - Prob. 17.42CPCh. 17.7 - Prob. 17.43CPCh. 17.7 - Which operator must be overloaded in a class...Ch. 17.7 - Prob. 17.45CPCh. 17.7 - What is a predicate?Ch. 17.7 - Prob. 17.47CPCh. 17.7 - Prob. 17.48CPCh. 17.7 - Prob. 17.49CPCh. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - If you want to store objects of a class that you...Ch. 17 - Prob. 9RQECh. 17 - Prob. 10RQECh. 17 - How does the behavior of the equal_range() member...Ch. 17 - Prob. 12RQECh. 17 - When using one of the STL algorithm function...Ch. 17 - You have written a class, and you plan to store...Ch. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 19RQECh. 17 - Prob. 20RQECh. 17 - Prob. 21RQECh. 17 - A(n) ___________ container stores its data in a...Ch. 17 - _____________ are pointer-like objects used to...Ch. 17 - Prob. 24RQECh. 17 - Prob. 25RQECh. 17 - The _____ class is an associative container that...Ch. 17 - Prob. 27RQECh. 17 - Prob. 28RQECh. 17 - A _______ object is an object that can be called,...Ch. 17 - A _________ is a function or function object that...Ch. 17 - A ____________ is a predicate that takes one...Ch. 17 - A __________ is a predicate that takes two...Ch. 17 - A __________ is a compact way of creating a...Ch. 17 - T F The array class is a fixed-size container.Ch. 17 - T F The vector class is a fixed-size container.Ch. 17 - T F You use the operator to dereference an...Ch. 17 - T F You can use the ++ operator to increment an...Ch. 17 - Prob. 38RQECh. 17 - Prob. 39RQECh. 17 - T F You do not have to declare the size of a...Ch. 17 - T F A vector uses an array internally to store its...Ch. 17 - Prob. 42RQECh. 17 - T F You can store duplicate keys in a map...Ch. 17 - T F The multimap classs erase() member function...Ch. 17 - Prob. 45RQECh. 17 - Prob. 46RQECh. 17 - Prob. 47RQECh. 17 - Prob. 48RQECh. 17 - T F If two iterators denote a range of elements...Ch. 17 - T F You must sort a range of elements before...Ch. 17 - T F Any class that will be used to create function...Ch. 17 - T F Writing a lambda expression usually requires...Ch. 17 - T F You can assign a lambda expression to a...Ch. 17 - Prob. 54RQECh. 17 - Write a statement that defines an iterator that...Ch. 17 - Prob. 56RQECh. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 58RQECh. 17 - Prob. 59RQECh. 17 - The following code defines a vector and an...Ch. 17 - The following statement defines a vector of ints...Ch. 17 - Prob. 62RQECh. 17 - Prob. 63RQECh. 17 - Prob. 64RQECh. 17 - Look at the following vector definition: vectorint...Ch. 17 - Write a declaration for a class named Display. The...Ch. 17 - Write code that docs the following: Uses a lambda...Ch. 17 - // This code has an error. arrayint, 5 a; a[5] =...Ch. 17 - // This code has an error. vectorstring strv =...Ch. 17 - // This code has an error. vectorint numbers(10);...Ch. 17 - // This code has an error. vectorint numbers ={1,...Ch. 17 - Prob. 72RQECh. 17 - Prob. 73RQECh. 17 - // This code has an error. vectorint v = {6, 5, 4,...Ch. 17 - // This code has an error. auto sum = ()[int a,...Ch. 17 - Unique Words Write a program that opens a...Ch. 17 - Course Information Write a program that creates a...Ch. 17 - Prob. 3PCCh. 17 - File Encryption and Decryption Write a program...Ch. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 6. File Analysis Write a program that reads the contents of two text files and compares them in the fol- lowing ways: • It should display a list of all the unique words contained in both files. • It should display a list of the words that appear in both files. • It should display a list of the words that appear in the first file but not the second. • It should display a list of the words that appear in the second file but not the first. • It should display a list of the words that appear in either the first or second file, but not both. Hint: Use set operations to perform these analyses.arrow_forwardC++ Programming. Associative and unordered associative containers. Associative containers (set, map, multiset, multimap). • When executing a given task, the input values must be read from a text file. Task : Create a program that deletes a set of elements from a single word of a given string type and creates a second set of elements that consist of a single word, and also displays it on the screen.arrow_forwardJAVA PPROGRAM Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found. While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. The file may contain up to 100 names. You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList. Input validation: a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…arrow_forward
- Program Specification For this assignment you will write a program to help people record the events of their day by supplying prompts and then saving their responses along with the question and the date to a file. Functional Requirements This program must contain the following features: Write a new entry - Show the user a random prompt (from a list that you create), and save their response, the prompt, and the date as an Entry. Display the journal - Iterate through all entries in the journal and display them to the screen. Save the journal to a file - Prompt the user for a filename and then save the current journal (the complete list of entries) to that file location. Load the journal from a file - Prompt the user for a filename and then load the journal (a complete list of entries) from that file. This should replace any entries currently stored the journal. Provide a menu that allows the user choose these options Your list of prompts must contain at least five different prompts.…arrow_forwardProgram Description: The project is a student management system which maintains student records in a simple text file. Your task is to write a program to save a list of students records in a FILE and then perform several operations on this FILE. Your program will ask user to choose the option from a menu. The 3 major function in menu are: ADD STUDENT FIND STUDENT PRINT LIST Exit How it Works The menu is handled by do while and switch statement. Sample output The Methods to be implemented are as follows: 1. ADDSTUDENT This method will add a new student to the file. It takes 3 parameters ( student name, id, and GPA). Your program must ask the user to enter the details of new students: Name, ID and GPA 2. FINDSTUDENT This method will search for a student with his/her id in the file. If the student is found it will print her/his record to the output. If the student is not found it will print “There is no record of this student in this system” 3. PRINTLIST This method will print all the…arrow_forwardWord List File Reader This exercise assumes you have completed the Programming Exercise 7, Word List FileWriter. Write another program that reads the words from the file and displays the following data:• The number of words in the file.• The longest word in the file.• The average length of all of the words in the filearrow_forward
- C-Programming Write a program that tells you how many words in a text file are unique (meaning the word only appears once in the text file). List of words in text file:…arrow_forwardWord List File Writer: Write a program that asks the user how many words they would like to write to a file, andthen asks the user to enter that many words, one at a time. The words should be writtento a file (in python)arrow_forwardProgram Description:in C language The project is a student management system which maintains student records in a simple text file. Your task is to write a program to save a list of students records in a FILE and then perform several operations on this FILE. Your program will ask user to choose the option from a menu. The 3 major function in menu are: ADD STUDENT FIND STUDENT PRINT LIST Exit How it Works The menu is handled by do while and switch statement. Sample output The Methods to be implemented are as follows: 1. ADDSTUDENT This method will add a new student to the file. It takes 3 parameters ( student name, id, and GPA). Your program must ask the user to enter the details of new students: Name, ID and GPA 2. FINDSTUDENT This method will search for a student with his/her id in the file. If the student is found it will print her/his record to the output. If the student is not found it will print “There is no record of this student in this system” 3. PRINTLIST This method will…arrow_forward
- C++ Add a search command that asks the user for a string and finds the first line that contains that string. The search starts at the first line currently displayed and stops at the end of the file. If the string is found, the line that contains it is displayed at the top. In case the user enters a string X that does not occur in the file, the program should print the error message: ERROR: string "X" was not found.arrow_forwardVirus DNA files As a future doctor, Jojo works in a laboratory that analyzes viral DNA. Due to the pandemic During the virus outbreak, Jojo received many requests to analyze whether there was any viral DNA in the patient. This number of requests made Jojo's job even more difficult. Therefore, Jojo ask Lili who is a programmer for help to make a program that can read the file containing the patient's DNA data and viral DNA and then match them. If on the patient's DNA found the exact same string pattern, then write to the index screen the found DNA. Data contained in the file testdata.in Input Format The first line of input is the number of test cases TThe second row and so on as many as T rows are the S1 string of patient DNA and the S2 string of viral DNA separated by spaces Output Format The array index found the same string pattern. Constraints 1 ≤ T ≤ 1003 ≤ |S2| ≤ |S1| ≤ 100|S| is the length of the string.S will only consist of lowercase letters [a-z] Sample Input (testdata.in)…arrow_forwardProblem description Write a program that will read in a file of student academic credit data and create a list of students on academic warning. The list of students on warning will be written to a file. Each line of the input file will contain the student name (a single String with no spaces), the number of semester hours earned (an integer), the total quality points earned (a double). The program should compute the GPA (grade point or quality point average) for each student (the total quality points divided by the number of semester hours) then write the student information to the output file if that student should be put on academic warning. A student will be on warning if he/she has a GPA less than 1.5 for students with fewer than 30 semester hours credit, 1.75 for students with fewer than 60 semester hours credit, and 2.0 for all other students. Do the following:arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
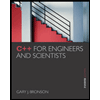
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr