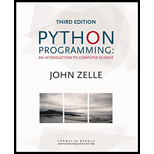
Tracking conference attendees
Program plan:
- In a file “atmmanager.py”,
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- Define the function “_init_()” to create a list of users and manage the account information.
- Define the “run()” function to execute the transaction according to user input.
- Define the function “screen_1()” to return the user input.
- Define the function “screen_2()” to process the transaction according to the user input.
- Define the function “get_User()” to return the user with the given user id.
- Define the function “send_Money()” to move the amount from one user to another. There are two types of accounts are used; “Checking” and “Savings”.
- Define the function “_updateAccounts()” to update the amount in output file whenever the transaction has occurred.
- Define the function “verify()” to check whether the userid and pin given by the user is matched with the saved userid and pin.
- Create a class “User”,
- Define the function “_init_()” method to assign the initial values.
- Define the function “check_Balances()” to return the amount of balance in checking and savings account.
- Define the function “exchange_Cash()” to deposit or withdraw amount fro checking or savings account.
- Define the function “transfer_Money()” to transfer the amount between checking and savings account.
- Define the function “getID()” to return the user id.
- Define the function “getPIN()” to return the pin.
- Create a class “ATMAp” that calls account information from JSON file and modifies it according to the commands given through an interface class.
- In the file “textatm.py”,
- Create a class “TextInterface”,
- Define “_init_()” method to assign initial values.
- Define the function “get_UserInput()” to return the user inputs.
- Define the function “choose_Transaction()” to select the type of transaction.
- Define the function “display_Balanace()” to print the amount in savings and checking accounts.
- Define the function “withdraw_Cash()” to return the withdraw amount and account type.
- Define the function “getTransferInfo()” to return the information of amount transfer between checking and savings account.
- Define the function “getSendInfo()” to return the information of receiver amount transfer.
- Define the function “close()” to exit the transaction.
- Create a class “TextInterface”,
- In a file “atm.py”,
- Import necessary packages.
- Define the “main()” function,
- All the interface class “TextInterface”.
- Call the “ATMAp” class functions with the argument of file and interface.
- Call the “main()” function.

This Python program is to keep track of conference attendees.
Explanation of Solution
Program:
File name: “atmmanager.py”
#Import package
import json
#Create a class
class ATMAp:
#Define the function
def __init__(self, account_file, interface):
#Open the file
with open(account_file) as fi:
#Serialize the object as a json stream
data = json.load(fi)
#Create a list of users
self.users = []
'''Create for loop to iterate key, value pairs till the list of tuples'''
for key,value in data['users'].items():
#Assign the value
usr = User(value["userid"], value["pin"],value["checkin"],value["saving"], value["name"])
#Append to the list
self.users.append(usr)
#Assign the value
self.interface = interface
#Call the run()
self.run()
#Define the run() function
def run(self):
#Execute while loop
while not self.interface.close():
#Call the functions
self.screen_1()
self.screen_2()
#Define the function
def screen_1(self):
#Assign the values
user_id = None
pin = None
#Execute while loop
while not self.verify(user_id, pin):
#Make simultaneous assignment
user_id, pin = self.interface.get_UserInput()
#Assign the value return from get_USer()
self.user = self.get_User(user_id)
#Define the function
def screen_2(self):
#Assign the value
terminate = self.interface.close()
#Execute while loop
while not terminate:
#Make simultaneous assignment
checkin, saving = self.user.check_Balances()
#Assign the value
tran_type = self.interface.choose_Transaction()
#Call the function
self.__updateAccounts()
#Execute the condition
if tran_type[0] == "C":
#Call the function
self.interface.display_Balance(checkin, saving)
#Execute the condition
elif tran_type[0] == "W":
#Make simultaneous assignment
value, account_type = self.interface.withdraw_Cash()
#Call the function
self.user.exchange_Cash(value, account_type)
#Execute the condition
elif tran_type[0] == "T":
#Make simultaneous assignment
inaccount, outaccount, value = self.interface.getTransferInfo()
#Call the function
self.user.transfer_Money(inaccount, outaccount, value)
#Execute the condition
elif tran_type[0] == "S":
#Make the assignment
recipient, type_sender, type_recipient, value = self.interface.getSendInfo()
#Assign the value return from get_User()
recipient = self.get_User(recipient)
#Call the function
self.send_Money(self.user, recipient, value, type_sender, type_recipient)
#Execute the condition
elif tran_type[0] == "Q":
#Call the function
self.user.exchangeCash(-100, "Checking")
#Execute the condition
elif tran_type[0] == "E":
#Call the unction
self.__updateAccounts()
#Assign the boolean value
terminate = True
#Define the function
def get_User(self, userid):
#Create for loop
for user in self.users:
#Check the condition
if userid == user.getID():
#Return the user object for the given userid
return user
#Define the function
def send_Money(self, sender, recipient, value, type_sender, type_recipient):
#Assign the values return from check_Balances()
checking, savings = sender.check_Balances()
checking, savings = recipient.check_Balances()
#Call the functions
sender.exchange_Cash(-value, type_sender)
recipient.exchange_Cash(value, type_recipient)
#Define the function
def __updateAccounts(self):
#Create dictionary
data = {}
#Assign empty dictionary
data['users'] = {}
#Create for loop
for usr in self.users:
#Assign the values
json_str = (vars(usr))
user_id = usr.getID()
#Assign the value
data['users'][user_id] = json_str
#Open the output file in write mode
with open('new_atmusers.json', 'w') as f:
#Serialize the objecct as a json stream
json.dump(data, f, indent=4)
#Define the function
def verify(self, user_id, pin):
#Create for loop
for usr in self.users:
#Execute the condition
if (usr.getID() == user_id) and (usr.getPIN() == pin):
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
#Create a class
class User:
#Define _init_() method
def __init__(self, userid, pin, checkin, saving, name):
#Assign initial values
self.userid = userid
self.pin = pin
self.checkin = float(checkin)
self.saving = float(saving)
self.name = name
#Define the function
def check_Balances(self):
#Return two values
return self.checkin, self.saving
#Define the function
def exchange_Cash(self, value, inaccount):
#Check the condition
if inaccount[0] == "C":
#Assign the value
self.checkin = round(self.checkin + value, 2)
else:
#Assign the value
self.saving = round(self.saving + value, 2)
#Define the function
def transfer_Money(self, inaccount, outaccount, value):
#Check the condition
if inaccount[0] == "C":
#Assign the values
self.checkin = self.checkin + value
self.saving = self.saving - value
#Otherwise
else:
#Assign the values
self.checkin = self.checkin - value
self.saving = self.saving + value
#Define the function
def getID(self):
"""Returns userid"""
#Return the value
return self.userid
#Define the function
def getPIN(self):
#Return the value
return self.pin
File name: “textatm.py”
#Create a class
class TextInterface:
#Define the function
def __init__(self):
#Assign boolean value
self.active = True
#Print the string
print("Welcome to the ATM")
#Define the function
def get_UserInput(self):
#Get user inputs
a = input("Username >> ")
b = input("Pin >> ")
#Return the values
return a, b
#Define the function
def choose_Transaction(self):
#Display user balance
#Assign the value
tran = "xxx"
#Execute the loop
while tran[0] != ("C" or "W" or "T" or "S" or "E" or "Q"):
#Get the choice
tran = input('Please select a transaction from the approved list. "Check Balance", \n "Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> ')
#Check the condition
if tran[0] != "E":
#Return the choice
return tran
else:
#Assign boolean value
self.active = False
#Return the choice
return tran
#Define the function
def display_Balance(self, checking, savings):
#Print savings and checking
print("Checking: {0} Savings: {1}".format(checking, savings))
#Define the function
def withdraw_Cash(self):
#Print the string
print("This function will withdraw or deposit cash.")
#Get the values
value = eval(input("Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> "))
account = input("Checking or Savings account? >> ")
#Print the string
print("Transaction is complete.\n")
#Retuen the values
return value, account
#Define the function
def getTransferInfo(self):
#Get the inputs
outaccount = input("Select account to withdraw money from: >> ")
inaccount = input("Select account to deposit money in: >> ")
value = eval(input("How much would you like to move?"))
#Print the string
print("Transaction is complete.\n")
#Return the values
return inaccount, outaccount, value
#Define the function
def getSendInfo(self):
#Get the inputs
recipient = input("Recipient userid")
type_sender = input("Sender: Checking or Savings? >> ")
type_recipient = input("Recipient: Checking or Savings? >> ")
value = eval(input("How much to send? >> "))
#Print the string
print("Transaction is complete.\n")
#Return the values
return recipient, type_sender, type_recipient, value
#Define the function
def close(self):
#Execute the condition
if self.active == False:
#Print the string
print("\nPlease come again.")
#Return boolean value
return True
#Otherwise
else:
#Return boolean value
return False
File name: “atm.py”
#Import packages
from atmmanager import ATMAp, User
from textatm import TextInterface
#Define the function
def main():
#Assign the value return from TextInterface()
interface = TextInterface()
#Assign the value return from ATMApp class
app = ATMAp("new_atmusers.json", interface)
#Call the function
main()
Content of “new_atmusers.json”:
{
"users": {
"xxxx": {
"userid": "xxxx",
"pin": "1111",
"checkin": 2000.0,
"saving": 11000.0,
"name": "Christina"
},
"ricanontherun": {
"userid": "ricanontherun",
"pin": "2112",
"checkin": 8755.0,
"saving": 7030.0,
"name": "Christian"
},
"theintimidator": {
"userid": "theintimidator",
"pin": "3113",
"checkin": 1845.0,
"saving": 112.0,
"name": "Sean"
},
"jazzplusjazzequalsjazz": {
"userid": "jazzplusjazzequalsjazz",
"pin": "4114",
"checkin": 4489.0,
"saving": 24000.0,
"name": "Dan"
}
}
}
Output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Check Balance
Checking: 2000.0 Savings: 11000.0
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Additional output:
Welcome to the ATM
Username >> xxxx
Pin >> 1111
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Withdraw/Deposit Cash
This function will withdraw or deposit cash.
Enter the amount to deposit/withdraw? Enter negative value to withdraw. >> 100
Checking or Savings account? >> Checking
Transaction is complete.
Please select a transaction from the approved list. "Check Balance",
"Withdraw/Deposit Cash", "Transfer Money", "Send Money", "Exit", "Quick Cash $100 >> Exit
Please come again.
>>>
Want to see more full solutions like this?
Chapter 12 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Write a program that lets the user play the slot machine (https://en.wikipedia.org/wiki/Slot_machine).A slot machine features a screen displaying three or more reels. To play, the user inserts money and activates the machine by means of a lever or button (either physical or on a touchscreen). The reels then spin and stop to rearrange the symbols. If the rearranged symbols match a winning combination, the player earns points based on a pay table. Symbols vary depending on the theme of the machine. Classic symbols include objects such as fruits, bells, and stylized lucky sevens.In this assignment, we slightly modify the operating mode of the slot machine as follows. Instead of the user manually activating the machine every time they want to play, the program will automatically play on behalf of the user multiple times. The user can choose from the following menu:1-> Play n times. The machine will repeatedly play n times.2-> Play until the credit gets below a minimum value. The…arrow_forwardWrite a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 5.1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. Don’t display the computer’s choice yet. 5.2. The user enters his or her choice of “rock”, “paper”, or “scissors” at the keyboard. Gather this input using JOptionPane.showInputDialog(). 5.3. Display the computer’s choice. 5.4. A winner is selected according to the following rules: If one player chooses rock and the other player chooses scissors, then rock wins.Display something to the effect of: “Rock smashes the scissors.” If one player chooses scissors and the other player chooses paper, then scissors wins.Display something like: “Scissors cuts paper.” If one player chooses paper and the other player…arrow_forwardWrite a program to design a graphical user Interface for Your coffee shop or restaurant or any business from your own choice. Your program should allow the customer to make an order using a button called Make an order. The customer can select the order from a drop down list (at least must have 4 products in the menu). For example, Green Tea, Late, Cappuccino, Tea. The customer can customise their order such as (no sugar, with sugar, with cream, with milk, ...etc). The customer can select the size of the order such as (regular, large). The customer can see the details of his order with the price before clicking the button Make Payment. The customer should insert the total amount of the order in the input box then click on Make Payment. The program should check if the amount of the total price is equal to the input number the customer entered, then the program can show a message "payment successfully done and your order is under process", otherwise the program should ask the user to…arrow_forward
- Write a program that calculates how much money you’ll end up with if you invest an amount of money at a fixed interest rate, compounded yearly. Have the user furnish the initial amount, the number of years, and the yearly interest rate in percent. Some interaction with the program might look like this: Enter initial amount: 3000 Enter number of years: 10 Enter interest rate (percent per year): 5.5 At the end of 10 years, you will have 5124.43 dollars.arrow_forwardWrite a program that calculates how much money you’ll end up with if you invest an amount of money at a fixed interest rate, compounded yearly. Have the user furnish the initial amount, the number of years, and the yearly interest rate in percent. Some interaction with the program might look like this: Enter initial amount: 3000 Enter number of years: 10 Enter interest rate (percent per year): 5.5 At the end of 10 years, you will have 5124.43 dollars. Java programming languagearrow_forwardWrite a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’tdisplay the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Therock smashes the scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins.(Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paperwraps rock.)• If both players make the same choice, the game must be…arrow_forward
- Write a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forwardWrite a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forwardHelp with JavaFX barchart: I have a program that allows a user to deposit and withdraw money while updating their balance based on the month the transaction occured, but I am having trouble with the programs class and am trying to make a bar chart that will have the total balance of that month, so as an example in May the bar would show $70, while the bar for June would show $100, even if the deposits were $20 and $50, then $30, $40 and $30.arrow_forward
- Write a program that asks the user for his name and salutes him using it. The prompt must be "What is your name?" and the response "Hello, !". For example if the name is John, the response must be "Hello, John!". Your last line of text must end with a new line character.arrow_forwardWrite a program that simulates a traffic light. The program lets the user select one of three lights: red, yellow, or green. When a radio button is selected, the light is turned on. Only one light can be on at a time (see Figure (b)). No light is on when the program starts. Using java programmingarrow_forwardWrite a program that simulates a snail trying to crawl up a building of height 6steps. The snail starts on the ground, at height 0. In each iteration, the snaileither crawls up one step, or slips off one step and falls all the way back to theground. In each iteration you would take a number as input from user and if the number is less 50 than the snail will slip otherwise it will crawl up. The program should keep going until the snail gets to the top of the building. It should then print out the number of falls that the snail took before it finally reached the top.Here is a sample execution:Iteration 1: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 40 Snail Moved: DownNumber of falls: 8arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
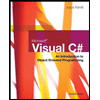