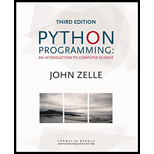
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 3PE
Program Plan Intro
Tracking conference attendees
Program plan:
- Import the package.
- Create a class “Conference_Manager”,
- Define the “_init_()” function,
- Create empty list.
- Open the input file in read mode.
- Read all the characters from the file using “read()”.
- Parse the json string using “json_loads()”.
- Create a “for” loop to iterate all the keys which are obtained from “json_object”.
- Assign initial values by invoking “_init_()”.
- Append the value at the end of the list.
- Close the input file.
- Define the getter method “get_JSON()”,
- Return the key, value pairs get from “json_object”.
- Define the getter method “get_Attendee()”,
- Create “for” loop to iterate over elements of list.
- Check whether the value return from “get_Name()” is equal to the value stored in “name,
- Return the list.
- Check whether the value return from “get_Name()” is equal to the value stored in “name,
- Create “for” loop to iterate over elements of list.
- Define the getter method “find_ByState()”,
- Create a list.
- Create “for” loop to iterate over elements of list.
- Check whether the value return from “get_State()” is equal to the value stored in “state”,
- If it is true, append the value to the end of the list.
- Check whether the value return from “get_State()” is equal to the value stored in “state”,
- Return the list.
- Define the getter method “make_Attendee()”,
- Assign the value to the list return from “_init_ ()” method from Attendee class.
- Append the value to the end of the list.
- Define the method “del_Attendee()”,
- Create for loop,
- Check whether the name is return from “get_name()”,
- If it is true, remove the element using “pop()”.
- Check whether the name is return from “get_name()”,
- Create for loop,
- Define the method “update_Conference()”,
- Create a list.
- Create for loop,
- Check whether the name is return from “get_name()”,
- Append the value to the end of the list.
-
- Open the output file in write mode,
- Serialize the object as a JSON formatted stream using “json_dump()”.
- Open the output file in write mode,
- Check whether the name is return from “get_name()”,
- Define the “_init_()” function,
- Create a class “Attendee”,
- Define the function “_init_()”,
- Assign the initial values for the variables.
- Define the getter method “get_Name()” to return the value stored in “name”.
- Define the getter method “get_Company()” to return the value stored in “company”.
- Define the getter method “get_State()” to return the value stored in “state”.
- Define the getter method “get_Email()” to return the value stored in “email”.
- Define the method “display_Info()” to print the formatted output.
- Define the function “_init_()”,
- Define the “main()” function,
- Call the constructor to initialize the values.
- Call “make_Attendee()” function.
- Assign the value return from “get_Attendee()”.
- Assign the value return from “find_ByState()”.
- Print the value return from “get_Company()”.
- Print the value return from “get_Name()”.
- Print the value return from “get_Sate()”.
- Print the value return from “get_Email()”.
- Call the function “display_Info()”.
- Create for loop,
- Print the value return from “get_Name()”.
- Call the “main()” function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Create a datafile that contains the first name, last name, gender, age, height, smoking preference, eye color and phone number. Add a variety of records to the file. A sample file looks like:

Write a program that opens the file and reads the records one by one. The program will skip any records where the gender preference is not a match. Of those records that match the gender preference, check to see if the age and height are between the maximum and minum preferences. Then check to see if the smoking preference and eye color are also a match. If at least 3 of the remaining fields match, consider the record a partial match, and print it in the report. If all 4 of the remaining fields match, the record is a perfect match and print it in the report with an asterisk next to it. At the end of the program, close the file and report how many total records there were of the specified gender, how many were a partial match, and how many were a perfect match.
A temperature file consists of five records, each containing a temperature in degrees Fahrenheit. A program is to be written that will read the input temperature, convert it from degrees Fahrenheit to degrees Celsius and print both temperatures in two columns on a report. Column headings, which read ‘Degrees F’ and ‘Degrees C’, are to be printed at the top of the page. What ist he best solution algorithm to successfully implement the above question?
Write an application that allows a user to input customer records (ID number, first & last name, and balance owed) and save each record to a file
Chapter 12 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In Chapter 11, you created the most recent version of the MarshallsRevenue program, which prompts the user for customer data for scheduled mural painting. Now, save all the entered data to a file that is closed when data entry is complete and then reopened and read in, allowing the user to view lists of customer orders for mural types.arrow_forwardIn Unix You have a record structured file named mydata that has four columns with default delimiter (white space). Sort the file based on its second column and redirect the file to a new file called sortedData.arrow_forwardYou are given a file called detention.txt with a list of students names and ID numbers. The data will be stored as shown below: Erin Shaw,36425109 Aldo Montes,8736542 Jessica,587963 Logan,22587 Leo Alvera,6544836 etc... Note that not all the students will have their last names in the detention.txt (names will not repeat in the file) Your job is to create a c++ program for teachers to look up a students name and display their student ID. Example output. (Input is highlighted) Welcome Staff! Please enter a student's name: Leo Alvera Searching for Leo Alvera... We found the Student ID: 6544836 for Leo Alvera Please enter a student's name: Jessica Alvera Searching for Jessica Alvera... Jessica Alvera not in detention.txt system Please enter a student's name: Please give proper explanation and typed answer only.arrow_forward
- Write a program that generates student records in a database file. Your program should be able to generate at least 10 records. The format in the database should be: student name, student id The first student id should be 20180001, second id will be 20180001 and so forth.arrow_forwardAdd a command to this chapter’s case study program that allows the user to view the contents of a file in the current working directory. When the command is selected, the program should display a list of filenames and a prompt for the name of the file to be viewed. Be sure to include error recovery in the program. If the user enters a filename that does not exist they should be prompted to enter a filename that does exist.arrow_forwardThis project involves generating a boarding pass ticket and storing it in a file. The application should take the passenger details as input. The details of the boarding pass are to be stored in a file. The details should include valid data such as: name, email, phone number, gender, age, boarding pass number, date, origin, destination, estimated time of arrival (ETA), departure time. The application should generate a boarding pass ticket using the boarding pass details. The generated ticket should contain the following information: Boarding Pass Number, Date, Origin, Destination, Estimated time of arrival (ETA), Departure Time Name, Email, Phone Number, Gender, Age Total Ticket Price The user will be required to enter their Name, Email, Phone Number, Gender, Age, Date, Destination, and Departure Time into the console or GUI. From the input the computer must generate the ETA and Ticket Price. The computer must generate the boarding pass number ensuring the number is unique. All…arrow_forward
- Create a program that simulates a simple registration and login function. Your program should access, write, edit and update data in the credentials.csv file. Note: Create your own credentials.csv file. Add one record having the following value: Juan Dela Cruz, January-1-1995, jdc_000@yahoo.com, Admin, 123456 STEP 1: At the start of the program execution, it should prompt the user of the following options: Register Login STEP 2: If the user chose option #1, the program will prompt the user on the following fields: First Name Middle Name Last Name Date of birth Email address Username Password Confirm Password STEP 2a: Password and Confirm Password should match, thus, incorrect inputs on those 2 fields should not be accepted. STEP 2b: After filling up the fields correctly, the program will then display the following: Display Profile Reset Password Log-out STEP 2c: Options #1 - Display Profile and #2 - Reset Password’s functions are optional. No need to code it in…arrow_forwardIf you remove a file by accident and want to get it back, you must follow the steps below.arrow_forwardUsing any database (one we have created before or a new one), create a program that uses Scanners OR Window Builder to take input from the user and perform: • One 'SELECT' query • One 'INSERT' query • One 'UPDATE' query • One 'DELETE' query *The queries you decide to perform are completely up to you*arrow_forward
- A file has r = 20, 000 STUDENT records of fixed length. Each record has the following fields: NAME (30 bytes), SSN (9 bytes), ADRESS(40 bytes), PHONE(9 bytes), BIRTHDATE (8 bytes), SEX(1 byte), CLASSCODE( 4 bytes, integer) MAJORDEPTCODE(4 bytes), MINORDEPTCODE(4 bytes), and DEGREEPROGRAM( 3 bytes). An additional byte is used as a deletion marker. Block size B = 512 bytes. a) Calculate the blocking factor bfr (=floor(B/R), where R is the record size) and number of file blocks b, assuming unspanned organization (a record can’t be split across blocks). b) Suppose only 80% of the STUDENT records have a value for PHONE, 85% for MAJORDEPTCODE, 15% for MINORDEPTCODE, and 90% for DEGREEPROGRAM. We use a variable-length record file. Each record has a 1-byte field type for each field in the record, plus the 1-byte deletion marker and a 1-byte end-of-record marker. Suppose that we use a spanned record organization, where each block has a 5-byte pointer to the next block (this space is not used…arrow_forwardWrite a program to keep track of conference attendees. For each attendee,your program should keep track of name, company, state, and email address. Your program should allow users to do things such as add a newattendee, display information on an attendee, delete an attendee, list thenames and email addresses of all attendees, and list the names and emailaddresses of all attendees from a given state. The attendee list should bestored in a file and loaded when the program startsarrow_forwardGiven the variable names pathName contains a string describing a directory path, write a statement that will store the names of all files in a List structure named volume. DO NOT traverse the path/directory. You are only interested in the immediate path. You may assume that any neccesay statments already exist.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
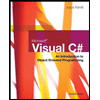
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Files & File Systems: Crash Course Computer Science #20; Author: CrashCourse;https://www.youtube.com/watch?v=KN8YgJnShPM;License: Standard YouTube License, CC-BY
UNIX Programming (Part - 10) The File System (Directories and Files Names); Author: ITUTEES;https://www.youtube.com/watch?v=K35faWBhzrw;License: Standard Youtube License