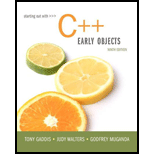
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 11, Problem 15RQE
The class Stuff has both a copy constructor and an overloaded = operator. Assume that blob and clump are both instances of the Stuff class. For each of the statements, indicate whether the copy constructor or the overloaded = operator will be called.
Stuff blob = clump;
clump = blob;
blob.operator=(clump);
showValues(blob); // Blob is passed by value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Problem Statement
You are working as a Devops Administrator.
Y
ou’ve been
t
asked to deploy a multi
-
tier application on Kubernetes Cluster. The application is a NodeJS application
available on Docker Hub with the following name:
d
evopsedu/emp
loyee
This Node
JS application works with a mongo database. MongoDB
image
is available
on
D
ockerHub with the following name:
m
ongo
You are required to deploy this application on Kubernetes:
•
NodeJS is available on port 8888 in the container
and will be reaching out to por
t
27017 for mongo database connection
•
MongoDB will be accepting connections on
port 27017
You must deploy this application using the CL
I
.
Once your application is up and running, ensure you can add an employee from the
NodeJS application and verify by
going to Get Employee page and retrieving your input.
Hint:
Name the Mongo DB Service and deployment, specifically as “mongo”.
I need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergent
I need help in my server client in C language
Chapter 11 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 11.3 - What is the difference between an instance member...Ch. 11.3 - Static member variables are declared inside the...Ch. 11.3 - Does a static member variable come into existence...Ch. 11.3 - What limitation does a static member function...Ch. 11.3 - What action is possible with a static member...Ch. 11.3 - If class X declares function f as a friend, does...Ch. 11.3 - Suppose that class Y is a friend of class X,...Ch. 11.5 - Briefly describe what is meant by memberwise...Ch. 11.5 - Prob. 11.9CPCh. 11.5 - Prob. 11.10CP
Ch. 11.5 - When is a copy constructor called?Ch. 11.5 - How does the compiler know that a member function...Ch. 11.5 - What action is performed by a classs default copy...Ch. 11.6 - Assume there is a class named Pet. Write the...Ch. 11.6 - Assume that dog and cat are instances of the Pet...Ch. 11.6 - What is the disadvantage of an overloaded ...Ch. 11.6 - Prob. 11.17CPCh. 11.6 - Prob. 11.18CPCh. 11.6 - Assume there is a class named Animal, which...Ch. 11.6 - Prob. 11.20CPCh. 11.6 - Describe the values that should he returned from...Ch. 11.6 - Prob. 11.22CPCh. 11.6 - What type of object should an overloaded operator...Ch. 11.6 - Prob. 11.24CPCh. 11.6 - If an overloaded or operator accesses a private...Ch. 11.6 - Prob. 11.26CPCh. 11.6 - When overloading a binary operator such as or as...Ch. 11.6 - Explain why overloaded prefix and postfix and ...Ch. 11.6 - Prob. 11.29CPCh. 11.6 - Overload the function call operator ( ) (int i,...Ch. 11.8 - Prob. 11.31CPCh. 11.8 - How is the type declaration of an r value...Ch. 11.8 - Prob. 11.33CPCh. 11.8 - Prob. 11.34CPCh. 11.8 - Which operator must be overloaded in a class...Ch. 11.8 - Prob. 11.36CPCh. 11.10 - What arc the benefits of having operator functions...Ch. 11.10 - Prob. 11.38CPCh. 11.10 - Assume that there is a class named BlackBox. Write...Ch. 11.10 - Assume there are two classes, Big and Smal1.Write...Ch. 11.13 - What type of relationship between classes is...Ch. 11.13 - Why does it make sense to think of a base class as...Ch. 11.13 - What is a base class access specification?Ch. 11.13 - Think of an example of two classes where one class...Ch. 11.13 - What is the difference between private members and...Ch. 11.13 - What is the difference between member access...Ch. 11.13 - Suppose a program has the following class...Ch. 11.14 - What is the reason that base class constructors...Ch. 11.14 - Why do you think the arguments to a base class...Ch. 11.14 - Passing arguments to base classes constructors...Ch. 11.14 - What will the following program display? #include...Ch. 11.14 - What will the following program display? #include...Ch. 11 - If a member variable is declared _____, all...Ch. 11 - Static member variables are defined _____ the...Ch. 11 - A(n) _____ member function cannot access any...Ch. 11 - A static member function may be called _____ any...Ch. 11 - A(n) _____ function is not a member of a class,...Ch. 11 - A(n) _____ tells the compiler that a specific...Ch. 11 - _____ is the default behavior when an object is...Ch. 11 - A(n) _____ is a special constructor, called...Ch. 11 - _____ is a special built-in pointer that is...Ch. 11 - An operator may be _____ to work with a specific...Ch. 11 - When the _____ operator is overloaded, its...Ch. 11 - Making an instance of one class a member of...Ch. 11 - Object composition is useful for creating a(n)...Ch. 11 - A constructor that takes a single parameter of a...Ch. 11 - The class Stuff has both a copy constructor and an...Ch. 11 - Explain the programming steps necessary to make a...Ch. 11 - Explain the programming steps necessary to make a...Ch. 11 - Consider the following class declaration: class...Ch. 11 - Describe the difference between making a class a...Ch. 11 - What is the purpose of a forward declaration of a...Ch. 11 - Explain why memberwise assignment can cause...Ch. 11 - Explain why a classs copy constructor is called...Ch. 11 - Explain why the parameter of a copy constructor...Ch. 11 - Assume a class named Bird exists. Write the header...Ch. 11 - Assume a class named Dollars exists. Write the...Ch. 11 - Assume a class named Yen exists. Write the header...Ch. 11 - Assume a class named Length exists. Write the...Ch. 11 - Assume a class named Collection exists. Write the...Ch. 11 - Explain why a programmer would want to overload...Ch. 11 - Each of the following class declarations has...Ch. 11 - A derived class inherits the _____ of its base...Ch. 11 - The base class named in the following line of code...Ch. 11 - The derived class named in the following line of...Ch. 11 - In the following line of code, the class access...Ch. 11 - In the following line of code, the class access...Ch. 11 - Protected members of a base class are like _____...Ch. 11 - Complete the following table by filling in...Ch. 11 - Complete the following table by filling in...Ch. 11 - Complete the following table by filling in...Ch. 11 - When both a base class and a derived class have...Ch. 11 - When both a base class and a derived class have...Ch. 11 - An overridden base class function may be called by...Ch. 11 - Each of the following class declarations and/or...Ch. 11 - Soft Skills 44. Your companys software is a market...Ch. 11 - Check Writing Design a class Numbers that can be...Ch. 11 - Day of the Year Assuming that a year has 365 days,...Ch. 11 - Day of the Year Modification Modify the DayOfYear...Ch. 11 - Number of Days Worked Design a class called...Ch. 11 - Palindrome Testing A palindrome is a string that...Ch. 11 - Prob. 6PCCh. 11 - Corporate Sales A corporation has six divisions,...Ch. 11 - Prob. 8PCCh. 11 - Rational Arithmetic II Modify the class Rational...Ch. 11 - HTML Table of Names and Scores Write a class whose...Ch. 11 - Prob. 11PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Determine the internal normal force between lettered points on the cable and rod. Draw all necessary free-body ...
Mechanics of Materials (10th Edition)
What does a subclass inherit from its superclass?
Starting Out with Python (4th Edition)
For the circuit shown, find (a) the voltage υ, (b) the power delivered to the circuit by the current source, an...
Electric Circuits. (11th Edition)
public class MyClass { private int x; private double y; public static void setValues(int a, double b) { x = a; ...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
In Exercises 1 through 52, determine the output produced by the lines of code. DimdtlAsDate=2/1/20162016wasalea...
Introduction To Programming Using Visual Basic (11th Edition)
Why is the study of database technology important?
Database Concepts (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forwardChange the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forward
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
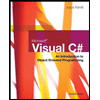
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Call By Value & Call By Reference in C; Author: Neso Academy;https://www.youtube.com/watch?v=HEiPxjVR8CU;License: Standard YouTube License, CC-BY