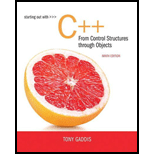
Inventory Bins
Write a
Description of the part kept in the bin
Number of parts in the bin
The program should have an array of 10 bins, initialized with the following data:
Part Description | Number of Parts in the Bin |
Valve | 10 |
Bearing | 5 |
Bushing | 15 |
Coupling | 21 |
Flange | 7 |
Gear | 5 |
Gear Housing | 5 |
Vacuum Gripper | 25 |
Cable | 18 |
Rod | 12 |
The program should have the following functions:
AddParts—increases a specific bin’s part count by a specified number.
RemoveParts—decreases a specific bin’s part count by a specified number.
When the program runs, it should repeat a loop that performs the following steps: The user should see a list of what each bin holds and how many parts are in each bin. The user can choose to either quit the program or select a bin. When a bin is selected, the user can either add parts to it or remove parts from it. The loop then repeats, showing the updated bin data on the screen.
Input Validation: No bin can hold more than 30 parts, so don’t let the user add more than a bin can hold. Also, don’t accept negative values for the number of parts being added or removed.

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Modern Database Management
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Mechanics of Materials (10th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- Considering the TM example of binary sum ( see attached)do the step-by-step of execution for the binary numbers 1101 and 11. Feel free to use the Formal Language Editor Tool to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step.arrow_forwardI need help on inculding additonal code where I can can do the opposite code of MatLab, where the function of t that I enter becomes the result of F(t), in other words, turning the time-domain f(t) into the frequency-domain function F(s):arrow_forwardI need help with the TM computation step-by-step execution for the binary numbers 1101 and 11. Formal Language Editor Tool can be used to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step;arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
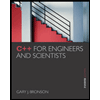
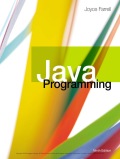
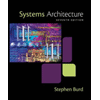
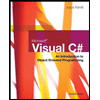
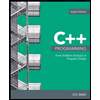