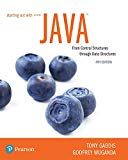
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10, Problem 3FTE
// Superclass
public class Vehicle
{
private double cost;
public Vehicle (double c)
{
cost = c;
}
(Other methods …)
}
// Subclass
public class Car extends Vehicle
{
private int passengers;
public Car (int p)
{
passengers = c;
}
(Other methods …)
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
public class Person {
private String personID; private String firstName; private String lastName; private String birthDate; private String address;
public Person(){
personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = "";
}
public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add);
}
public void setPerson(String id, String first, String last, String birth, String add){
personID = id; firstName = first; lastName = last; birthDate = birth; address = add;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
public String getBirthdate(){
return birthDate;
}
public String getAddress(){ return address;
}
public void print(){
System.out.print("\nPerson ID = " + personID);
System.out.print("\nFirst Name = " +firstName);
System.out.print("\nLast Name = " +lastName);
System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address);
}
public String…
public class Person {
private String personID; private String firstName; private String lastName; private String birthDate; private String address;
public Person(){
personID = ""; firstName = ""; lastName = ""; birthDate = ""; address = "";
}
public Person(String id, String first, String last, String birth, String add){ setPerson(id,first,last,birth,add);
}
public void setPerson(String id, String first, String last, String birth, String add){
personID = id; firstName = first; lastName = last; birthDate = birth; address = add;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
public String getBirthdate(){
return birthDate;
}
public String getAddress(){ return address;
}
public void print(){
System.out.print("\nPerson ID = " + personID);
System.out.print("\nFirst Name = " +firstName);
System.out.print("\nLast Name = " +lastName);
System.out.print("\nBirth Date = " +birthDate); System.out.print("\nAddress = " +address);
}
public String…
C#
List the differences between CommissionEmployee class and BasePlusCommissionEmployee class
public class BasePlusCommissionEmployee
{
public string FirstName { get; }
public string LastName { get; }
public string SocialSecurityNumber { get; }
private decimal grossSales;
private decimal commissionRate;
private decimal baseSalary;
public BasePlusCommissionEmployee(string firstName, string lastName,
string socialSecurityNumber, decimal grossSales,
decimal commissionRate, decimal baseSalary)
{
FirstName = firstName;
LastName = lastName;
SocialSecurityNumber = socialSecurityNumber;
GrossSales = grossSales;
CommissionRate = commissionRate;
BaseSalary = baseSalary;
}
public decimal GrossSales
{
get
{
return grossSales;
}
set
{
if (value < 0) // validation
{
throw new ArgumentOutOfRangeException(nameof(value),…
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 10.1 - Here is the first line of a class declaration....Ch. 10.1 - Look at the following class declarations and...Ch. 10.1 - Class B extends class A. (Class A is the...Ch. 10.2 - Prob. 10.4CPCh. 10.2 - Look at the following classes: public class Ground...Ch. 10.3 - Under what circumstances would a subclass need to...Ch. 10.3 - How can a subclass method call an overridden...Ch. 10.3 - If a method in a subclass has the same signature...Ch. 10.3 - If a method in a subclass has the same name as a...Ch. 10.3 - Prob. 10.10CP
Ch. 10.4 - When a class member is declared as protected, what...Ch. 10.4 - What is the difference between private members and...Ch. 10.4 - Why should you avoid making class members...Ch. 10.4 - Prob. 10.14CPCh. 10.4 - Why is it easy to give package access to a class...Ch. 10.6 - Look at the following class definition: public...Ch. 10.6 - When you create a class, it automatically has a...Ch. 10.7 - Recall the Rectangle and Cube classes discussed...Ch. 10.8 - Prob. 10.19CPCh. 10.8 - If a subclass extends a superclass with an...Ch. 10.8 - What is the purpose of an abstract class?Ch. 10.8 - If a class is defined as abstract, what can you...Ch. 10.9 - Prob. 10.23CPCh. 10.9 - Prob. 10.24CPCh. 10.9 - Prob. 10.25CPCh. 10.9 - Prob. 10.26CPCh. 10.9 - Prob. 10.27CPCh. 10.9 - Prob. 10.28CPCh. 10 - In an inheritance relationship, this is the...Ch. 10 - In an inheritance relationship, this is the...Ch. 10 - This key word indicates that a class inherits from...Ch. 10 - A subclass does not have access to these...Ch. 10 - This key word refers to an objects superclass. a....Ch. 10 - In a subclass constructor, a call to the...Ch. 10 - The following is an explicit call to the...Ch. 10 - A method in a subclass that has the same signature...Ch. 10 - A method in a subclass having the same name as a...Ch. 10 - These superclass members are accessible to...Ch. 10 - Prob. 11MCCh. 10 - With this type of binding, the Java Virtual...Ch. 10 - This operator can be used to determine whether a...Ch. 10 - When a class implements an interface, it must...Ch. 10 - Prob. 15MCCh. 10 - Prob. 16MCCh. 10 - Abstract classes cannot ___________. a. be used as...Ch. 10 - You use the __________ operator to define an...Ch. 10 - Prob. 19MCCh. 10 - Prob. 20MCCh. 10 - You can use a lambda expression to instantiate an...Ch. 10 - True or False: Constructors are not inherited.Ch. 10 - True or False: in a subclass, a call to the...Ch. 10 - True or False: If a subclass constructor does not...Ch. 10 - True or False: An object of a superclass can...Ch. 10 - True or False: The superclass constructor always...Ch. 10 - True or False: When a method is declared with the...Ch. 10 - True or False: A superclass has a member with...Ch. 10 - True or False: A superclass reference variable can...Ch. 10 - True or False: A subclass reference variable can...Ch. 10 - True or False: When a class contains an abstract...Ch. 10 - True or False: A class may only implement one...Ch. 10 - True or False: By default all members of an...Ch. 10 - // Superclass public class Vehicle { (Member...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { public...Ch. 10 - Write the first line of the definition for a...Ch. 10 - Look at the following code, which is the first...Ch. 10 - Write the declaration for class B. The classs...Ch. 10 - Write the statement that calls a superclass...Ch. 10 - A superclass has the following method: public void...Ch. 10 - A superclass has the following abstract method:...Ch. 10 - Prob. 7AWCh. 10 - Prob. 8AWCh. 10 - Look at the following interface: public interface...Ch. 10 - Prob. 1SACh. 10 - A program uses two classes: Animal and Dog. Which...Ch. 10 - What is the superclass and what is the subclass in...Ch. 10 - What is the difference between a protected class...Ch. 10 - Can a subclass ever directly access the private...Ch. 10 - Which constructor is called first, that of the...Ch. 10 - What is the difference between overriding a...Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is an. abstract class?Ch. 10 - Prob. 12SACh. 10 - When you instantiate an anonymous inner class, the...Ch. 10 - Prob. 14SACh. 10 - Prob. 15SACh. 10 - Employee and ProductionWorker Classes Design a...Ch. 10 - ShiftSupervisor Class In a particular factory, a...Ch. 10 - TeamLeader Class In a particular factory, a team...Ch. 10 - Essay Class Design an Essay class that extends the...Ch. 10 - Course Grades In a course, a teacher gives the...Ch. 10 - Analyzable Interface Modify the CourseGrades class...Ch. 10 - Person and Customer Classes Design a class named...Ch. 10 - PreferredCustomer Class A retail store has a...Ch. 10 - BankAccount and SavingsAccount Classes Design an...Ch. 10 - Ship, CruiseShip, and CargoShip Classes Design a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Write a void method called display such that the invocation display (a) will display the contents of the array ...
Java: An Introduction to Problem Solving and Programming (8th Edition)
The decimal number system is a weighted system with ten digits.
Digital Fundamentals (11th Edition)
the variable die1.
Java How To Program (Early Objects)
Indentify and correct the errors in each of the following. [Note: There may be more than one error in each piec...
C How to Program (8th Edition)
Days in a Month Write a class named MonthDays, The classs constructor should accept two arguments: An integer f...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- public class Cylinder { public void smoothen() { } } } public abstract class Vessel implements Steerable { private Engine e; } System.out.println("Smoothening.."); public Engine getEngine() { return e; } public void setEngine (Engine e) { this.e = e; } public abstract void turnOn(); public class Engine { private Cylinder [] y; private int cyls = 1; static final int maxCylinders = 12; public void addCylinder (Cylinder y) { if (cylsarrow_forwardJAVAarrow_forwardQUESTION 15 public class numClass { private int a; private static int y = 10; public numClass(int newX) {a=newx; } public void set(int newx) { a=newx; y+=a; } public void setY(int newy) { y=newY; } public static int getY() { return y; } }// end of class public class output { public static void main(String[] args) { numClass one = new numClass(10); numClass two = new numClass (2); try{ one. sety(30); two.set(4); if(one.getY()==30) throw new Exception ("30"); one.setY(40); } catch (Exception e) { two.sety (50); } System.out.println(two.getY()); }// end of main } // end of classarrow_forwardpublic class LabProgram { public static void main(String args[]) { Course course = new Course(); String first; // first name String last; // last name double gpa; // grade point average first = "Henry"; last = "Cabot"; gpa = 3.5; course.addStudent(new Student(first, last, gpa)); // Add 1st student first = "Brenda"; last = "Stern"; gpa = 2.0; course.addStudent(new Student(first, last, gpa)); // Add 2nd student first = "Jane"; last = "Flynn"; gpa = 3.9; course.addStudent(new Student(first, last, gpa)); // Add 3rd student first = "Lynda"; last = "Robison"; gpa = 3.2; course.addStudent(new Student(first, last, gpa)); // Add 4th student course.printRoster(); } } // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average…arrow_forward49. Consider the following class definition. public class Cylinder } private double baseRadius; private double height; public Cylinder () baseRadius = 0; height = 0; public Cylinder (double 1, double h) } baseRadius - 1; height = h; { public void set (double r, double h) } baseRadius = r; height = h; { public String toString () } return (baseRadius + It n + height); { public double SurfaceArea () return 2 * 3.14 * baseRadius height; public double volume () } return 3.14 baseRadius * baseRadius * height; { Which of the following statements correctly instantiates the Cylinder object myCylinder? (i) Cylinder mycylinder = new Cylinder (2.5, 7.3) : (ii) class Cylinder myCylinder = new Cylinder (2.5, 7.3); (ii) myCylinder new Cylinder (2.5, 7.3); %3D a. Only (i) b. Only (i) c. Only (iüi) d. Both (ii) and (ii)arrow_forwardConver the class To UML diagram class Clint { private String accNumber; private String holderName; private long currBalance; public Clint() { currBalance = 0; } public Clint(String acc, String name, long bal) { accNumber = acc; holderName = name; currBalance = bal; } Scanner sc = new Scanner(System.in); // deposit money into the bank account by given amount amt public void deposit(long amt) { currBalance += amt; } // if amount is not given ask the user for the amount public void deposit() { System.out.print("Enter Amount(to deposit): $"); long amt = sc.nextLong(); currBalance += amt; } // withdraw money from the bank account public void withdraw(long amt) { if (currBalance >= amt) { currBalance -= amt; } else { System.out.println("Insufficient funds!"); } } // if amt is not given ask the user for the amount public…arrow_forwardpublic class Player { private int jerseyNumber; private int rating; public Player(int jerseyNumber, int rating) { this.jerseyNumber = jerseyNumber; this.rating = rating; } public int getJerseyNumber() { return jerseyNumber; } public void setJerseyNumber(int jerseyNumber) { this.jerseyNumber = jerseyNumber; } public int getRating() { return rating; } public void setRating(int rating) { this.rating = rating; } @Override public String toString() { return "Jersey number: " + jerseyNumber + ", Rating: " + rating; }}public class RosterManager { private ArrayList<Player> roster; public RosterManager() { roster = new ArrayList<>(); } public void addPlayer(Player player) { roster.add(player); } public void updatePlayerRating(int jerseyNumber, int newRating) { for (Player player : roster) { if (player.getJerseyNumber() ==…arrow_forwardPublic Class SavingsAccount { float interest; float FixedDeposit; SeniorAccount(float interest, float FixedDeposit) { this. interest = interest; this.fixedDeposit= FixedDeposit; } float calculateInterest(); { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*interest/100); } } Public Class SeniorAccount extends SavingAccount { float seniorInterest; SeniorAccount(float interest, float FixedDeposit) { this.seniorInterest=interest; super(interest, FixedDeposit) } float calculateInterest() { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*seniorinterest/10); } } Public static void main(String args[]) { SavingsAccount saving = new SavingsAccount(6,100000); System.out.println(saving.calculateinterest()); SeniorAccount senior=new seniorAccount(10,100000); System.out.println(Senior.calculateInterest()); } Correct the syntax and logical errorarrow_forwardPublic Class SavingsAccount { float interest; float FixedDeposit; SeniorAccount(float interest, float FixedDeposit) { this. interest = interest; this.fixedDeposit= FixedDeposit; } float calculateInterest(); { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*interest/100); } } Public Class SeniorAccount extends SavingAccount { float seniorInterest; SeniorAccount(float interest, float FixedDeposit) { this.seniorInterest=interest; super(interest, FixedDeposit) } float calculateInterest() { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*seniorinterest/10); } } Public static void main(String args[]) { SavingsAccount saving = new SavingsAccount(6,100000); System.out.println(saving.calculateinterest()); SeniorAccount senior=new seniorAccount(10,100000); System.out.println(Senior.calculateInterest()); } Rubric: Correct the syntax and logical errors Proper working codearrow_forwardPublic classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forwardCode: interface Bicycle{ //interface for bicycle void changeGear(int val); //abstract methods void changeSpeed(int inc); void applyBrakes(int dec); void ringBell(int count); } class NewCycle implements Bicycle{ //class for a new cycle int speed=0; //stores the speed value int gear=0; //stores the gear value @Override public void changeGear(int val) { //method to change the gear gear=val; } @Override public void changeSpeed(int inc) { //method to change the speed speed=speed+inc; } @Override public void applyBrakes(int dec) { //method to apply brakes speed=speed-dec; } @Override public void ringBell(int count) { //method to ring the bell for(int i=0;i<count;i++){ System.out.print("Clang!!"+" "); } System.out.println(""); } public void printState(){ //method to print the states System.out.println("Ring bell, Speed, Gear,…arrow_forwardpublic class Application { public static void main(String[] args) { Employee empl = new Employee(); empl.firstName = "Ali"; empl.lastName = "Omar"; empl.salary = 20000; Employee emp2 = new Employee(); emp2.firstName = "Mohamed"; emp2.lastName = "Nour"; emp2.salary = 30000; System.out.println("- System.out.printin("- empl.employeeSalary(); System.out.println("- empl.checkEmployeeSalary(emp1.salary, emp2.salary); System.out.printin("- System.out.println("- printEmployee(emp2); System.out.printin("- Employee employeeArr[] = new Employee[2]: employeeArr[0] = empl; employeeArr[1] = emp2; Application a = new Åpplication(); a.printArrayofEmployee(employeeArr); Non static (instance) start to call Static function to print ---------"); "); - Non Static function to print - --"); Non static (instance) start to call same class Static function to print --------); -"); Non Static function to print ----); System.out.println("- System.out.printin("- System.out.printin("Salary : " + Employee.salary);…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
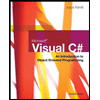
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
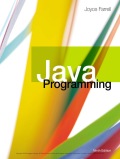
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY