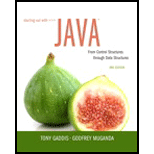
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10, Problem 18MC
You use the __________ operator to define an anonymous inner class.
- a. class
- b. inner
- c. new
- d. anonymous
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A class that is not intended to be instantiated, but used only as a base class, is called a(n) __________. a. dummy class b. subclass c. virtual class d. abstract class
Q#: Write a class Email that contain the following fields, which other classes may not access:
To
From
Subject
Content
Write a function member called SendEmail that initialize all the class fields through information passing as parameters. SendEmail method will print the content of email only.
Write a driver class with any name that must have a main method. Inside main, call SendEmail.
3- Create a class named Player with a default name as "player", a default level as 0, and a default class type of "fighter". Feel free to add any other attributes you wish! Create an instance method in the Player class called attack that takes another player as a parameter. This function should compare the level of the two players, and return whether the player won, lost, or tied. Finally create a loop that allows the user to input a name and a class type for two players; the level of both players should be determined at random (between 1 and 99). Print out the name and attributes of each player, and have the first player attack the second. Print whether the player won, lost, or tied. Ask if the user wants to try again. (Python code)
Chapter 10 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 10.1 - Here is the first line of a class declaration....Ch. 10.1 - Look at the following class declarations and...Ch. 10.1 - Class B extends class A. (Class A is the...Ch. 10.2 - Prob. 10.4CPCh. 10.2 - Look at the following classes: public class Ground...Ch. 10.3 - Under what circumstances would a subclass need to...Ch. 10.3 - How can a subclass method call an overridden...Ch. 10.3 - If a method in a subclass has the same signature...Ch. 10.3 - If a method in a subclass has the same name as a...Ch. 10.3 - Prob. 10.10CP
Ch. 10.4 - When a class member is declared as protected, what...Ch. 10.4 - What is the difference between private members and...Ch. 10.4 - Why should you avoid making class members...Ch. 10.4 - Prob. 10.14CPCh. 10.4 - Why is it easy to give package access to a class...Ch. 10.6 - Look at the following class definition: public...Ch. 10.6 - When you create a class, it automatically has a...Ch. 10.7 - Recall the Rectangle and Cube classes discussed...Ch. 10.8 - Prob. 10.19CPCh. 10.8 - If a subclass extends a superclass with an...Ch. 10.8 - What is the purpose of an abstract class?Ch. 10.8 - If a class is defined as abstract, what can you...Ch. 10.9 - Prob. 10.23CPCh. 10.9 - Prob. 10.24CPCh. 10.9 - Prob. 10.25CPCh. 10.9 - Prob. 10.26CPCh. 10.9 - Prob. 10.27CPCh. 10.9 - Prob. 10.28CPCh. 10 - In an inheritance relationship, this is the...Ch. 10 - In an inheritance relationship, this is the...Ch. 10 - This key word indicates that a class inherits from...Ch. 10 - A subclass does not have access to these...Ch. 10 - This key word refers to an objects superclass. a....Ch. 10 - In a subclass constructor, a call to the...Ch. 10 - The following is an explicit call to the...Ch. 10 - A method in a subclass that has the same signature...Ch. 10 - A method in a subclass having the same name as a...Ch. 10 - These superclass members are accessible to...Ch. 10 - Prob. 11MCCh. 10 - With this type of binding, the Java Virtual...Ch. 10 - This operator can be used to determine whether a...Ch. 10 - When a class implements an interface, it must...Ch. 10 - Prob. 15MCCh. 10 - Prob. 16MCCh. 10 - Abstract classes cannot ___________. a. be used as...Ch. 10 - You use the __________ operator to define an...Ch. 10 - Prob. 19MCCh. 10 - Prob. 20MCCh. 10 - You can use a lambda expression to instantiate an...Ch. 10 - True or False: Constructors are not inherited.Ch. 10 - True or False: in a subclass, a call to the...Ch. 10 - True or False: If a subclass constructor does not...Ch. 10 - True or False: An object of a superclass can...Ch. 10 - True or False: The superclass constructor always...Ch. 10 - True or False: When a method is declared with the...Ch. 10 - True or False: A superclass has a member with...Ch. 10 - True or False: A superclass reference variable can...Ch. 10 - True or False: A subclass reference variable can...Ch. 10 - True or False: When a class contains an abstract...Ch. 10 - True or False: A class may only implement one...Ch. 10 - True or False: By default all members of an...Ch. 10 - // Superclass public class Vehicle { (Member...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { private...Ch. 10 - // Superclass public class Vehicle { public...Ch. 10 - Write the first line of the definition for a...Ch. 10 - Look at the following code, which is the first...Ch. 10 - Write the declaration for class B. The classs...Ch. 10 - Write the statement that calls a superclass...Ch. 10 - A superclass has the following method: public void...Ch. 10 - A superclass has the following abstract method:...Ch. 10 - Prob. 7AWCh. 10 - Prob. 8AWCh. 10 - Look at the following interface: public interface...Ch. 10 - Prob. 1SACh. 10 - A program uses two classes: Animal and Dog. Which...Ch. 10 - What is the superclass and what is the subclass in...Ch. 10 - What is the difference between a protected class...Ch. 10 - Can a subclass ever directly access the private...Ch. 10 - Which constructor is called first, that of the...Ch. 10 - What is the difference between overriding a...Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is an. abstract class?Ch. 10 - Prob. 12SACh. 10 - When you instantiate an anonymous inner class, the...Ch. 10 - Prob. 14SACh. 10 - Prob. 15SACh. 10 - Employee and ProductionWorker Classes Design a...Ch. 10 - ShiftSupervisor Class In a particular factory, a...Ch. 10 - TeamLeader Class In a particular factory, a team...Ch. 10 - Essay Class Design an Essay class that extends the...Ch. 10 - Course Grades In a course, a teacher gives the...Ch. 10 - Analyzable Interface Modify the CourseGrades class...Ch. 10 - Person and Customer Classes Design a class named...Ch. 10 - PreferredCustomer Class A retail store has a...Ch. 10 - BankAccount and SavingsAccount Classes Design an...Ch. 10 - Ship, CruiseShip, and CargoShip Classes Design a...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Write the statement that calls a superclass constructor and passes the arguments x, y, and z.
Starting Out with Java: From Control Structures through Objects (6th Edition)
Code an SQL statement that creates a table with all columns from the parent and child tables in your answer to ...
Database Concepts (7th Edition)
Consider the following function declarations from the definition of the class Money in Display 11.4. void input...
Problem Solving with C++ (10th Edition)
Consider the variable allCents in the method writePositive in Listing 6.14. It holds an amount of money as if i...
Java: An Introduction to Problem Solving and Programming (8th Edition)
The hexadecimal number system has 16 characters, six of which are alphabetic characters.
Digital Fundamentals (11th Edition)
Find the error in the following pseudocode. Display Enter the length of the room. Input length Declare Integer ...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In a class diagram for a class named Computer that has a private double field named price, you want to represent a standard setter. Write the line you would enter to do this. ____ ____ ( ____ : ____ ) : ____arrow_forwardsolve in python Street ClassStep 1:• Create a class to store information about a street:• Instance variables should include:• the street name• the length of the street (in km)• the number of cars that travel the street per day• the condition of the street (“poor”, “fair”, or “good”).• Write a constructor (__init__), that takes four arguments corresponding tothe instance variables and creates one Street instance. Street ClassStep 2:• Add additional methods:• __str__• Should return a string with the Street information neatly-formatted, as in:Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.• compare:• This method should compare one Street to another, with the Street the method is calledon being compared to a second Street passed to a parameter.• Return True or False, indicating whether the first street needs repairs more urgently thanthe second.• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, whichneed repairs more…arrow_forwardDesign a class named StopWatch. The class contains:■ The private data fields startTime and endTime with get methods.■ A constructor that initializes startTime with the current time.■ A method named start() that resets the startTime to the current time.■ A method named stop() that sets the endTime to the current time.■ A method named getElapsedTime() that returns the elapsed time for thestop watch in milliseconds.Draw the UML diagram for the class, and then implement the class. Write a test program that measures the execution time of adding numbers from 1 to 1,000,000.arrow_forward
- Write a program named SalespersonDemo that instantiates objects using classes named Real EstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each objects data fields. First, create an abstract class named Salesperson. Fields include first and last names; the Salesperson constructor requires both these values. Include properties for the fields. Include a method that returns a string that holds the Salespersons full name—the first and last names separated by a space. Then perform the following Create two child classes of Salesperson: Real EstateSalesperson and Girl Scout. The Real EstateSalesperson class contains fields for total value sold in dollars and total commission earned (both of which are initialized to 0), and a commission rate field required by the class constructor. The Girl Scout class includes a field to hold the number of boxes of cookies sold, which is initialized to 0. Include properties for every field. Create an interface named ISell able that contains two methods: SalesSpeech() and MakeSale(). In each Real EstateSalesperson and Girl Scout class, implement SalesSpeech() to display an appropriate one- or two-sentence sales speech that the objects of the class could use. In the Real Estatesalesperson class, implement the MakeSale() method to accept an integer dollar value for a house, add the value to the Real EstateSalespersons total value sold, and compute the total commission earned. In the Girl Scout class, implement the MakeSale() method to accept an integer representing the number of boxes of cookies sold and add it to the total field.arrow_forwardCreate an application for Ninas Cookie Emporium named CookieDemo that declares and demonstrates objects of the CookieOrder class and its descendants. The CookieOrder class includes auto-implemented properties for an order number, recipients name, and cookie type (for example, chocolate chip), and fields for number of dozens ordered and price. When the field value for number of dozens ordered is set, the price field is set as $15 per dozen for the first two dozen and $13 per dozen for each dozen over two. Create a child class named Special Cookieorder, which includes a field with a description as to why the order is special (for example, gluten-free). Override the method that sets a CookieOrders price as described in part a, but also to include special handling, which is SIO for orders up to $40 and $8 for higher-priced orders. Create an application named CookieDem02 that demonstrates using several Special CookieOrder objects.arrow_forwardWrite a class named Car that has the following member variables: yearModel: An int that holds the car’s year model. name: A string that holds the name of the car. company: A string that holds the company of the car. speed: An int that holds the car’s current speed. In addition, the class should have the following member functions. Mutator: Appropriate accessor functions to set the values of object’s yearModel, name, company, and speed member variables. Accessor: Appropriate accessor functions to get the values stored in an object’s yearModel, company, and speed member variables. Accelerate: The accelerate function should add 5 to the speed member variable each time it is called. Brake: The brake function should subtract 5 from the speed member variable each time it is called. Demonstrate the class in a program that creates a Car object, call the setter functions to set the values and then call the accelerate function five times. After each call to the accelerate function, get…arrow_forward
- Create a class called Employee that contains two attributes: name and number. Next, write a class named Production Worker that is a subclass of the Employee class. The Production Worker class should add extra attributes called shift_number (an integer, either 1 or 2) and pay_rate. Write the appropriate accessor and mutator methods for each class. Once you have written the classes, write a program that creates an object of the Employee class and an object of the Production Worker class and prompts the user to enter data for each of the object's data attributes. Store the data in the object, then use the object's accessor methods to retrieve it and display it on the screen.arrow_forwardPlease code in python Create a program with two classes – the person class and the student The person class has the following properties: first name (first_name), last name (last_name) street address (address) city (city) zip code (zip) The class has the following methods: get_full_name, which returns the full name of a person get_full_address, which return greeting, returns a greeting message. The class should provide accessor and mutator for each property The class should override the __str__ method to return the state of the object. Create a child class called student which has a property named graduation year (graduation_year) and major. Provide accessor and mutator for each property of its own It inherits all the properties and methods of the person parent class as well. Create a test program that Create an object of the person class and print the full name of a person. Create an object of the person class and print…arrow_forwardTo declare a class as abstract, you use the __________ in the class header. a. abstract keyword b. base keyword c. void keyword d. virtual keywordarrow_forward
- Question Create a class called Quadratic for performing arithmetic on and solving quadratic equations. A quadratic equation is an equation of the form ax2 + bx + c = 0 Where a !=0. Use double variables to represent the values of a, b, and c and provide a constructor that enables objects of this class to be initialized when they are created. Give default values of a = 1, b = 0, and c = 0. Create a char variable called variable to represent the variable used in the equation and give it a default value of x. The constructor should not allow the value of a to be 0. If 0 is given, assign 1 to a). Provide public member functions that perform the following tasks. add—adds two Quadratic equations by adding the corresponding values of a, b, and c. The function takes another object of type Quadratic as its parameter and adds it to the calling object. subtract—subtracts two Quadratic equations by subtracting corresponding values of a, b, and…arrow_forwardTo hide one of a class’s members, you declare the member using which of the following keywords? a. Hide b. Invisible c. Private d. ReadOnlyarrow_forward1- The private part of Class called ----- and the public part called --. 2- The class that inherits from another class called 3- The objects in OOP can communicate with each others via 4- A constructor that has no parameters called-------.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
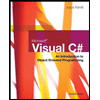
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
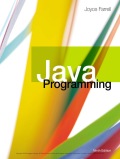
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY