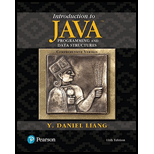
(Geometry: the Triangle2D class) Define the Triangle2D class that contains:
- Three points named p1, p2, and p3 of the type MyPoint with getter and setter methods. MyPoint is defined in
Programming Exercise 10.4. - A no-arg constructor that creates a default triangle with the points (0, 0), (1,1), and (2,5).
- A constructor that creates a triangle with the specified points.
- A method getArea () that returns the area of the triangle.
- A method getPerimeter () that returns the perimeter of the triangle.
- A method contains (MyPoint p) that returns true if the specified point p is inside this triangle (see Figure 10.22a).
- A method contains(Triang1e2D t) that returns true if the specified triangle is inside this triangle (see Figure 10.22b).
- A method overlaps(Triangle2D t) that returns true if the specified triangle overlaps with this triangle (see Figure 10.22c).
FIGURE 10.22 (a) A point is inside the triangle, (b) A triangle is inside another triangle. (c) A triangle overlaps another triangle.
Draw the UML diagram for the class and then implement the class. Write a test program that creates a Triangle2D object t1 using the constructor new Triangle2D(new MyPoint(2.5, 2), new MyPoint(4.2, 3), new MyPoint(5, 3.5)), displays its area and perimeter, and displays the result of t1.contains(3, 3), r1.contains(new Triangle2D(new MyPoint(2.9, 2), new MyPoint(4, 1). MyPoint(1, 3.4))).and t1 .overlaps(new Triangle2D(new MyPoint(2, 5.5), new MyPoint (4. –3), MyPoint(2, 6.5))).
(Hint: For the formula to compute the area of a triangle, sec Programming Exercise 2. 19. To detect whether a point is inside a triangle, draw three dashed lines, as shown in Figure 10.23. If the point is inside a triangle, each dashed line should intersect a side only once. If a dashed line intersects a side twice, then the point must be outside the triangle. For the
FIGURE 10.23 (a) A point is inside the triangle, (b) A point is outside the triangle.

Trending nowThis is a popular solution!

Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
C++ How to Program (10th Edition)
Concepts of Programming Languages (11th Edition)
Starting Out with Python (3rd Edition)
Programming in C
Modern Database Management (12th Edition)
- LocalResource(String date, String sector)- Actions of the constructor include accepting a date in the format “dd/mm/yyyy”, initializing the base class, storing the sector and storing an id as a consecutively increasing integer. Note that the constructor must ensure that the date of birth information is recorded. b. getId():Integer - returns the ID of the current instance of LocalResource c. getSector():String – returns the sector associated with the current instance of localResource d. getTRN():String – returns the trn number of the current instance of LocalResource. The process used to determine the TRN is to add the id to the number 100000000 and then returning the string equivalent. e. Update the NineToFiver class to ensure it properly extends the LocalResource class f. In method getContact() of NineToFiver, remove the comments so that the method returns "Local Employee #"+and the id of the contact. Write a concrete class LocalConsultant that extends LocalResource, and implements…arrow_forward(The Time class) Design a class named Time. The class contains: - The data fields hour, minute, and second that represent a time. - A no-arg constructor that creates a Time object for the current time. (The values of the data fields will represent the current time.) -A constructor that constructs a Time object with a specified elapsed time since midnight, January 1, 1970, in milliseconds. (The values of the data fields will represent this time.) -A constructor that constructs a Time object with the specified hour, minute, and second. - Three getter methods for the data fields hour, minute, and second, respectively. -A method named setTime (long elapseTime) that sets a new time for the object using the elapsed time. For example, if the elapsed time is 555550000 milliseconds, the hour is 10, the minute is 19, and the second is 10. Draw the UML diagram for the class and then implement the class. Write a test program that creates two Time objects (using new Time (), new Time(555550000),…arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward
- This program extends the earlier "Online shopping cart" program. (Consider first saving your earlier program). (1) Extend the ItemToPurchase class to contain a new attribute. item_description (string) - Set to "none" in default constructorImplement the following method for the ItemToPurchase class. print_item_description() - Prints item_description attribute for an ItemToPurchase object. Has an ItemToPurchase parameter. Ex. of print_item_description() output: Bottled Water: Deer Park, 12 oz. (2) Build the ShoppingCart class with the following data attributes and related methods. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Parameterized constructor which takes the customer name and date as parameters Attributescustomer_name (string) - Initialized in default constructor to "none"current_date (string) - Initialized in default constructor to "January 1, 2016"cart_items (list)Methodsadd_item()Adds an item to cart_items list. Has a parameter of…arrow_forwardClasses and Objects) Hand-write a complete Java class that can be used to create a Car object as described below. a. A Vehicle has-a: i. Registration number ii. Owner name iii. Price iv. Year manufactured b. Add all instance variables C. The class must have getters and setters for all instance variables d. The class must have two constructors, a no-args and a constructor that receives input parameters for each instance variable.arrow_forwardThis program extends the earlier "Online shopping cart" program. (Consider first saving your earlier program). (1) Extend the ItemToPurchase class to contain a new attribute. item_description (string) - Set to "none" in default constructorImplement the following method for the ItemToPurchase class. print_item_description() - Prints item_description attribute for an ItemToPurchase object. Has an ItemToPurchase parameter. Ex. of print_item_description() output: Bottled Water: Deer Park, 12 oz. (2) Build the ShoppingCart class with the following data attributes and related methods. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Parameterized constructor which takes the customer name and date as parameters Attributescustomer_name (string) - Initialized in default constructor to "none"current_date (string) - Initialized in default constructor to "January 1, 2016"cart_items (list)Methodsadd_item()Adds an item to cart_items list. Has a parameter of…arrow_forward
- Need help in Java programming. Write the class definitions for all classes. Be sure to include all properties and two getters and setters for each class. One of the getters and setters should be for a string datatype, and one for either a numeric or Boolean data type. At a minimum the following classes need to be defined: Customer (CustID, LastName, FirstName, Street, City, State, Zip, TaxExempt) Inventory (ItemID, Description, Cost, Selling Price, Quantity On Hand) Order Header (OrderID, CustID, OrderDate, Shipto, Amount Due) Order Detail (OrderID, Line Number, ItemID, Quantity Ordered, Selling Price, Total)arrow_forward(The Account class) Account -id: int -balance: double -annualInterestRate : double -dateCreated : Date +Account( ) +Account(someId : int, someBalance : double) +getId() : int +setId(newId : int) : void +getBalance() : double +setBalance(newBalance : double) : void +getAnnualInterestRate( ) : double +setAnnualInterestRate(newRate : double) : void +getDateCreated( ) : Date +getMonthlyInterestRate( ) : double +getMonthlyInterest( ) : double +withdraw(amt : double) : void +deposit(amt : double) : void Design a class named Account that contains: ■ A private int data field named id for the account (default 0). ■ A private double data field named balance for the account (default 0). ■ A private double data field named annualInterestRate that stores the current interest rate (default 0). Assume all accounts have the same interest rate. Make it static. ■ A private Date data field named dateCreated that stores the date when the account was created. (private Date dateCreated)…arrow_forward- Implement the class “cylinder” with member variables radius and height, whichare private of type double. Define the global constant PI=3.1415 and use it incalculating the volume of the cylinder (PI*radius*radius*height).Implement in the class cylinder the following functions:a- A default constructor with default values of one.b- One constructor with two arguments. This constructor should check that thevariable is positive and does not exceed 20, otherwise the variable will beassigned its default value of one.c- A reader for each variable.d- A writer for each variable.e- A reader and a writer for diameter.f- A member function “volume” which calculates the volume of a cylinder.g- A member function “print” which prints the radius, diameter, and height of acylinder.h- A member function display_name() which displays “cylinder”.2- Write a program which declares an array of n cylinders (use n=5). Initialize thearray values by writing a loop which asks the user to input the radius and…arrow_forward
- • Write a Point class with two properties: 1, y. Write required constructor, get & set, and toString methods. Write a Triangle class that has 3 Point properties. Write required constructor, get & set, and toString methods.arrow_forward(TicTacToe Class) Create a class TicTacToe that will enable you to write a complete programto play the game of tic-tac-toe. The class contains as private data a 3-by-3 two-dimensional arrayof integers. The constructor should initialize the empty board to all zeros. Allow two human players.Wherever the first player moves, place a 1 in the specified square. Place a 2 wherever the second player moves. Each move must be to an empty square. After each move, determine whether the gamehas been won or is a draw. If you feel ambitious, modify your program so that the computer makesthe moves for one of the players. Also, allow the player to specify whether he or she wants to go firstor second. If you feel exceptionally ambitious, develop a program that will play three-dimensionaltic-tac-toe on a 4-by-4-by-4 board. [Caution: This is an extremely challenging project that couldtake many weeks of effort!]arrow_forwardQuestion 7 (a) Suppose the class Spaghetti implements the Edible interface and the base class of Spaghetti is Food class. You have given the following variables declaration: Food food; new Spaghetti (); Spaghetti spa Edible e = null; %3D Identify the following statements as valid or invalid. i) e spa; spa = food; = new Food(): ii) spa food (Spaghetti) e: e = new Spaghetti(): CS Scanned with CamScannerarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
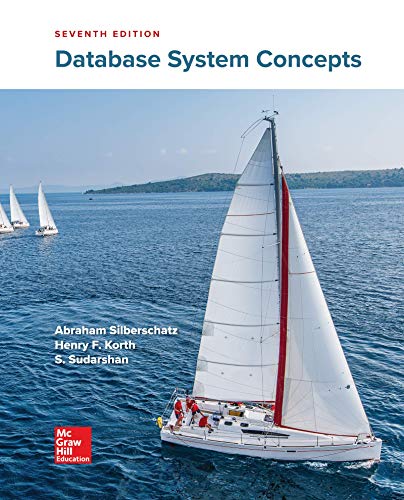
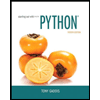
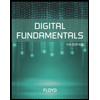
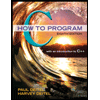
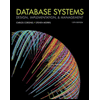
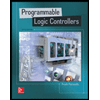