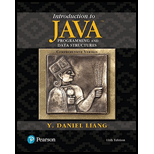
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 10.10, Problem 10.10.3CP
What is the output of the following code?
String s1 = “Welcome to Java”;
String s2 = s1.replace(“o”, “abc”)
System.out.println(s1);
System.out.println(s2);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Loamab keov.
using System;
class Program
{
publicstaticvoid Main(string[] args)
{
int number = 1;
while (number <= 88)
{
Console.WriteLine(number);
number = number + 2;
}
Random r = new Random();
int randNo= r.Next(1,88);
Console.WriteLine("Random Number between 1 and 88 is "+randNo);
}
}
how would this program be modified to choose three random numbers instead of just one number between 1-88?
using System;
class Program
{
publicstaticvoid Main(string[] args)
{
int number = 1;
while (number <= 88)
{
Console.WriteLine(number);
number = number + 2;
}
Random r = new Random();
int randNo= r.Next(1,88);
Console.WriteLine("Random Number between 1 and 88 is "+randNo);
}
}
how can this program be fixed to select three random numbers between 1 and 88 instead of one?
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
If you were writing the definitions for the Canine class member functions and wanted to place these in their ow...
Starting Out with C++: Early Objects (9th Edition)
What is pseudocode?
Starting Out With Visual Basic (8th Edition)
Find the error in the following class: public class TwoValues { private int x, y; public TwoValues() { x = 0; }...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
What is the disadvantage of having too many features in a language?
Concepts Of Programming Languages
Write a program to play the rock-paper-scissor game. Each of two users types in either P, R, or S. The program ...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- JAVAarrow_forwardusing System; class TicTacToe { Console.WriteLine("Do you want be X or O: "); Console.Write("Press 1 for 'X' or Press 2 for 'O'\n"); choice = int.Parse(Console.ReadLine()); if(choice==1) staticint player = 1 staticint choice; { staticvoid Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } } How can this program be fixed to ask the user "It is your turn to place an X. Where would you like to place it?"arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1000000; while (number >= 1) { Console.WriteLine(number); number = number / 2; { Console.WriteLine(number); if(number==3){ Console.WriteLine("I found 3!"); } } } } } This code is written in C# and it starts with 1,000,000 and it divides it in half until the last number is 0. Something went wrong with the program and it prints the same number twice. how can that be fixed so it only prints out the numbers once instead of duplicating them before moving on the next? sorry if that doesn't make much sense!arrow_forward
- - Write a java program that accepts a sentence from the user and prints out the sentence with all uppercase letters changed to lowercase and all lowercase letters changed to uppercase. Repeat the operation until “???” string is entered. For example: Please enter a string …… HelLo hELlO Please enter a string …… ??? Donearrow_forwardusing System; class TicTacToe { Console.WriteLine("Do you want be X or O: "); Console.Write("Press 1 for 'X' or Press 2 for 'O'\n"); choice = int.Parse(Console.ReadLine()); if(choice==1) int player = 1 { staticvoid Print(char[] board) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } } Hello how can this be fixed to compile. I'm trying to get the program to ask the user if they want to be X or O at the beginning of the gamearrow_forwardTRUE or FALSE b. The below code run without any error. class Output { public static void main(String args[]) { Integer i = new Integer(257); byte x = i.byteValue(); System.out.print(x);arrow_forward
- By default, which one of the following types of values will be thrown when the below function is executed?def abstractAdd(a,b,c):print(a+b+c) Choose an answer A None B bool C str D intarrow_forwardint a = 10, b =7; System.out.println(a>b?a:b); Will always print what?arrow_forwardQuestion public class MyProgram { if (h 18) { public static void main(String[] args) { int h=10, t-22; System.out.print ("Good "); } } else } } What is the output displayed by the following lines of code?" System.out.print ("Morning"); t = t + 2; if (t > 30) System.out.println(" Every one!"); System.out.println("The Your answer system.out.print("Evening"); t = t + 5; temperature is " System.out.println(" Brink water?"); Degree.");arrow_forward
- using System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 50) { Console.WriteLine(number); number = number + 1; { number = 100; while (number <= 109) { Console.WriteLine(number); number = number + 1; } } } } } hello, how can this program be fixed to count from 1-50 and then count from 70-200?arrow_forward// Program prompts user to enter a series of integers // separated by spaces // Program converts them to numbers and sums them import java.util.*; public class DebugSeven2 { publicstaticvoidmain(String[] args) { String str; int x; int length; int start; int num; int lastSpace =-1; int sum =0; String partStr; Scanner in =newScanner(System.in); System.out.print("Enter a series of integers separated by spaces >> "); str = in.nextLine; length = str.length(); for(x =0; x <= length; ++x) { if(str.charAt(x) ==' ') { partStr = str.substring(lastSpace + 1, y); num = Integer.parseInt(partStr); System.out.println(" " + num); sum += number; lastSpace = x; } } partStr = str.substring(lastSpace + 1, length); num = Integer.parseInt(partStr); System.out.println(" " + num); sum += num; System.out.println(" -------------------" + "\nThe sum of the integers is "+ sum); } }arrow_forward// DebugFive4.java // Outputs highest of four numbers import java.util.*; public class DebugFive4 { publicstaticvoidmain (Stringargs[]) { Scanner input =newScanner(System.in); int one, two, three, four; String str, output; System.out.println("Enter an integer"); str = input.next(); one = Integer.parseInt(str); System.out.println("Enter an integer"); str = input.next(); two = Integer.parseInt(str); System.out.println("Enter an integer"); str = input.next(); three = Integer.parseInt(str); System.out.println("Enter an integer"); str = input.next(); four = Integer.parseInt(str); if(one > two > one > three > one > four) output = "Highest is " + four; else if(two > one && two > three !! two > four) output = "Highest is " + three; else if(three > one && three > two && three > four) output = "Highest is " + three; else output = "Highest is " + four; System.out.println(output); } }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
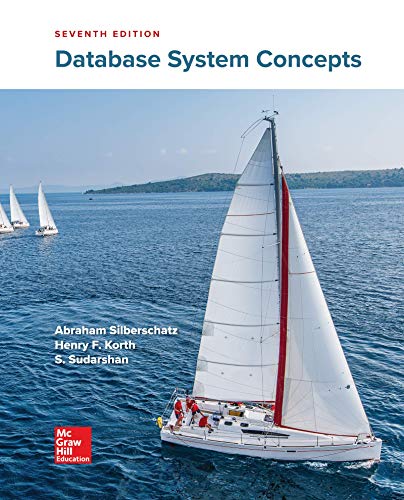
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
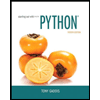
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
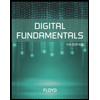
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
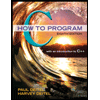
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
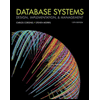
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
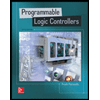
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
The Top Down Approach to Software Development; Author: Christopher Kalodikis;https://www.youtube.com/watch?v=v9M8LA2uM48;License: Standard YouTube License, CC-BY