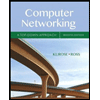
You’ve been given a list of books to read over the summer, but you only want to read the books by a certain author.
Write a method
public List<Book> filterBooks(List<Book> readingList, String author)
That takes a List of Books as a parameter, removes all Books from the readingList that are not written by the given author, then returns the resulting list.
You can access the author of a Book by calling book.getAuthor(). The Book class is provided for reference.
public List<Book> filterBooks(List<Book> readingList, String author)
{
// Remove all Books from the readingList that are not
// written by the given author. Return the resulting List
for(int i = 0; i < readingList.size(); i++)
{
//does current author == author?
String currentBook = readingList.get(i);
if(!currentBook.getAuthor().equals(author))
{
readingList.remove(i);
i--;
}
}
return readingList;
}
Book.java
public class Book
{
private String title;
private String author;
public Book(String theTitle, String theAuthor)
{
title = theTitle;
author = theAuthor;
}
public String getTitle()
{
return title;
}
public String getAuthor()
{
return author;
}
public String toString()
{
return title + " by " + author;
}
public boolean equals(Book other)
{
return title.equals(other.title) && author.equals(other.author);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- In java, Write a method void popMult(int k) for the ArrayBoundedStack class which will pop k items from this. Throw a StackUnderflowException and don't pop anything if there are fewer than k items on this. This is a method of the ArrayBoundedStack class. DO NOT USE ANY OTHER METHODS OF THE ARRAYBOUNDEDSTACK CLASS.arrow_forwardJava this piece of my code is not working .....I am trying to add Warship with a type or warship , but I keep getting an error ? //add another WarShip instance to the ArrayList. //use a foreach loop to process the ArrayList and print the data for each ship. //count the number of ships that are not afloat. Return this count to main for printing. //In the shipShow method: the number of ships that are still a Float public static int shipShow(ArrayList<Ship> fleetList) { //from the ArrayList, remove the CargoShip that was declared as type Ship. fleetList.remove(2); //Removing ElFaro form the list // review Type casting instanceof | up casting Ship < Warship , subclass is a object of its parents //add warship USS John Warner - add cast by (Ship) new WarShip Ship warship2 = (Ship) new WarShip("USS John Warner", 2015, true, "attack submarine", "United States"); fleetList.add(warship2); int count= 0; //use a foreach loop to process the ArrayList and print the data for each ship.…arrow_forwardhow would you do this in a simple way? this is a non graded practice labarrow_forward
- A pet shop wants to give a discount to its clients if they buy one or more pets and at least four other items. The discount is equal to 15 percent of the cost of the other items, but not the pets. Implement a method: public static void discount (double[] prices, boolean[] isPet, int nItems) The method receives information about a particular sale. For the ith item, prices[i] is the price before any discount, and isPet[i] is true if the item is a pet. Wite a program that prompts a cashier to enter each price and then a y for a pet orn for another item. Use a price of -1 as a sentinel. Save the inputs in an array. Call the method that you implemented, and display the discount.arrow_forward4. Say we wanted to get an iterator for an ArrayList and use it to loop over all items and print them to the console. What would the code look like for this? 5. Write a method signature for a method called foo that takes an array as an argument. The return type is void. 6. What is the difference between remove and clear in ArrayLists.arrow_forwardPlease answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…arrow_forward
- In this problem you will fill out three functions to complete the Group ADT and the Diner ADT. The goal is to organize how diners manage the groups that want to eat there and the tables where these groups sit. It is important to take the time to read through the docstrings and the doctests. Additionally, make sure to not violate abstraction barriers for other ADTS, i.e. when implementing functions for the Diner ADT, do not violate abstraction barriers for the Group ADT, and vice versa. # Diner ADT def make_diner (name): """ Diners are represented by their name and the number of free tables they have.""" return [name, 0] def num_free_tables (diner): return diner [1] def name (diner): return diner [0] # You will implement add_table and serve which are part of the Diner ADT # Group ADT def make_group (name): Groups are represented by their name and their status.""" return [name, 'waiting'] |||||| def name (group): return group [0] def status (group): return group [1] def start_eating…arrow_forwardThe play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…arrow_forwardLabProgram.java 1 import java.util.Scanner; 2 3 public class LabProgram { 4 5 /* Define your method here */ public static void main(String[] args) { /* Type your code here. */ } 7 8 9. 10 } 11arrow_forward
- Write a program that reads a list of integers, and outputs whether the list contains all multiples of 10, no multiples of 10, or mixed values. Define a method named isArrayMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing the size of the list. isArrayMult10) returns a boolean that represents whether the list contains all multiples of ten. Define a method named İsArrayNoMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing the size of the list. İsArrayNoMult10() returns a boolean that represents whether the list contains no multiples of ten. Then, write a main program that takes an integer, representing the size of the list, followed by the list values. The first integer is not in the list. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 20 40 60 80 100 the output is: all multiples of 10 Ex: If the input is: 5 11 -32 53 -74 95 the…arrow_forwardIn the existing CircularlyLinkedList class, Write a non-static method named getMax for finding the maximum element in a circularly linked list. Write the testing code in the main method of the class CircularlyLinkedList. For this purpose, you must only use and update the CircularlyLinkedList.java file provided in Lesson3Examples posted in the eCentennialmodule "Lesson Examples (from textbook)" package exercise3; public class CircularlyLinkedList<E> { private static class Node<E> { private E element; private Node<E> next; public Node(E e, Node<E> n) { element = e; next = n; } public E getElement() { return element; } public Node<E> getNext() { return next; } public void setNext(Node<E> n) { next = n; } } private Node<E> tail = null; private int size = 0; public CircularlyLinkedList() { } public int size() { return size; } public boolean isEmpty() { return size == 0; } public E first() { if (isEmpty()) return null; return…arrow_forwardWrite a program that reads a list of integers, and outputs whether the list contains all multiples of 10, no multiples of 10, or mixed values. Define a method named isArrayMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing the size of the list. isArrayMult10) returns a boolean that represents whether the list contains all multiples of ten. Define a method named isArrayNoMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing the size of the list. İsArrayNoMult10() returns a boolean that represents whether the list contains no multiples of ten. Then, write a main program that takes an integer, representing the size of the list, followed by the list values. The first integer is not in the list. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 20 40 60 80 100 the output is: all multiples of 10 Ex: If the input is: 5 11 -32 53 -74 95 the…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
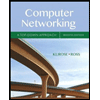
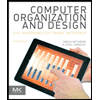
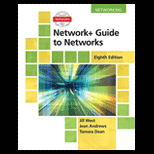
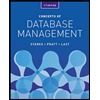
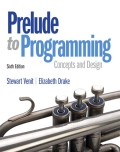
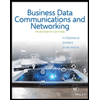