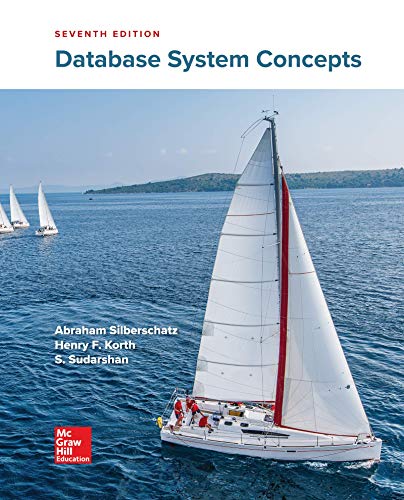
Concept explainers
package com.olympic.cis143.hw1.fizzbuzz;
public class FizzBuzzConverter {
public int[] data;
public FizzBuzzConverter(int[] values) {
if (values == null) {
throw new RuntimeException("Values passed cannot be null");
}
this.data = values;
}
/**
For this method, you will need to iterate through a list of ints and populate a return array with the correct values according to the rules of FizzBuzz. FizzBuzz is a common practice
If the int is a multiples of three print “Fizz�
If the int is a multiples of five print “Buzz�
If the int is a multiple of three and five print "FizzBuzz"
For example, if the array sent into the method contains the following ints [ 0, 1, 2, 3, 5, 10, 15, 7, 16], the returned Object array will be [0, 1, 2, "Fizz", "Buzz", "Buzz", "FizzBuzz", 7, 16]
Note that the method should accept an int array int[] data, and return an object array Object[].
*/
public Object[] convertToFizzBuzz() {
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Add the method below to the parking office.java class. Method getParkingCharges(Customer) : Money Parkingoffice.java public class ParkingOffice {String name;String address;String phone; List<Customer> customers;List<Car> cars;List<ParkingLot> lots;List<ParkingCharge> charges; public ParkingOffice(){customers = new ArrayList<>();cars = new ArrayList<>();lots = new ArrayList<>();charges = new ArrayList<>();}public Customer register() {Customer cust = new Customer(name,address,phone);customers.add(cust);return cust;}public Car register(Customer c,String licence, CarType t) {Car car = new Car(c,licence,t);cars.add(car);return car;}public Customer getCustomer(String name) {for(Customer cust : customers)if(cust.getName().equals(name))return cust;return null;}public double addCharge(ParkingCharge p) {charges.add(p);return p.amount;} public String[] getCustomerIds(){String[] stringArray1 = new String[2];for(int i=0;i<2;i++){stringArray1[i]…arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardin Jave create a method work() with the follow instructions: If the work() method is passed a non-null then it must return a new List that contains some or all of the elements in data. The elements of the new List must be aliases for (not copies of) the objects in the List it is passed. If there are no sign attributes (i.e., the array is null or has 0 elements) then the work() method must return an empty List. If any of the conditions represented by the sign attribute holds for a particular element that that element must be included in the result (i.e., the conditions must be combined using a logical OR). The elements in the returned List must be in the same order they appeared in the original List (though not all elements must be in the result). public List<T> work(List<T> g){arrow_forward
- *JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forwardIn Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forwardmain.py Load default template... 1 from random import randint 3 class RandomNumbers: # Type your code here. 4 5 main ": 6 if low = int(input()) high = int(input()) name 7 8 9 numbers = RandomNumbers() numbers. set_random_values(low, high) numbers.get_random_values() 10 11 12arrow_forward
- import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardWrite all the code within the main method in the TestTruck class below. Implement the following functionality. Construct two Truck objects: one with any make and model you choose and the second object with gas tank capacity 10. If an exception occurs, print the stack trace. Print both Truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main( String[] args ) { // write your code herearrow_forwardpublic class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forward
- Need help with menu loop pleasearrow_forwardpackage lab06;;public class gradereport { public static void main(String[] args) { Scanner in = new Scanner(System.in);double[] Scores = new double[10]; for(int i=0;i<10;i++){ System.out.println("Enter score " + (i+1));scores[i]=in.nextdouble(); } for(int i=0;i<10;i++){ if (scores[i] >=80) System.out.println("Score " + (i+1) + " receives a grade of HD"); else if (scores[i]>=70) System.out.println("Score " + (i+1) + " receives a grade of D"); else if (scores[i] >=60) System.out.println("Score "+ (i+1) + " receives a grade of C"); else if (scores[i] >=50) System.out.println("Score " + (i+1) + " receives a grade of P"); else if (scores[i] >=40) System.out.println("Score " + (i+1) + " receives a grade of MF"); else if (scores[i] >=0) System.out.println("Score " +…arrow_forwardIN JAVA PLEASE, need help finding an element in the list /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ public int indexOf(int value) { //Implement this method return -1; }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
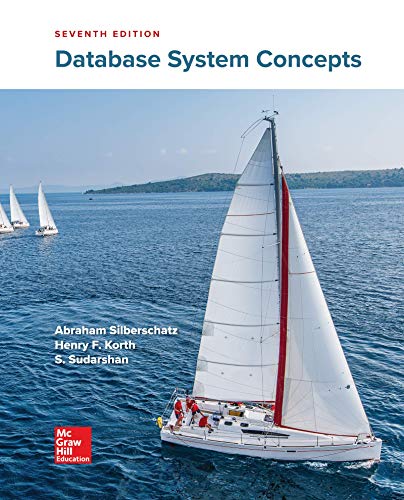
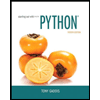
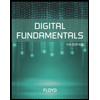
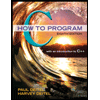
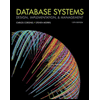
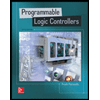