Write a method that accepts two lists of integer values and checks if they are identical or not (i.e. contain the same values). if they are not identical, the method must display the max value of each list.
Q: Write a complete method to create a modified ArrayList. Method Specifications The method takes in an…
A: Here I have defined the function named modifyArrayList(). In this function, I have used a loop to…
Q: Java: Please solve without using Hash set. The class will contain the following static methods:…
A: Introduction: In this problem, we are given a string as input and asked to perform various…
Q: 4- What is the difference between the following list methods: - replace() - pop()
A: Given: 4- What is the difference between the following list methods: - replace() - pop()
Q: Write a method called addBiggest to be considered in a class outside the KWArrayList class. This…
A: //code //KWArrayList class //import necessary packagesimport java.util.Arrays;//create KWArrayList…
Q: URLify: Write a method to replace all spaces in a string with '%20'. You may assume that the string…
A: The JAVA code is given below with code and output screenshot Happy to help you ?
Q: Java Program Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets the…
A: The problem asks to create an automated rock, paper, scissors game The Java code is provided below…
Q: Below is the specs and my main method. (ignore add and remove method for now) Specs: Part 1 -…
A: Here's a possible implementation of the TweetBot class: CODE in JAVA: import…
Q: Write a method called addSmallest to be considered in a class outside the KWArrayList class. This…
A: import java.util.*;/** This class implements some methods of the Java ArrayList class */public class…
Q: Create the Median class that has the method calculateMedian that calculates the median of the…
A: The above given problem statement is studied and the solution implementation by implementing method…
Q: URLify: Write a method to replace all spaces in a string with '%20'. You may assume that the string…
A: The question is to write the JAVA code for the given problem.
Q: sing a Listlterator do the following: Count total number of odd numbers and print the result.
A: Java code: import java.util.*;public class Main{ public static void main(String[] args) { int…
Q: get_nth_word_from_string(s, n): This function takes a string s and a non-negative integer n as…
A: def get_nth_word_from_string(s, n): # function to get the nth word from string index = 0…
Q: Task 2: Write a static method which takes an ArrayList of Strings and an integer and changes the…
A: Program import java.util.*;import java.util.stream.*; class Test { public static void…
Q: Write a method and test it to insert an array of elements at index in a single linked list and then…
A: //Java Source Code :- import java.util.Arrays; import java.util.LinkedList; import…
Q: Complete the method void add(T item). It adds an item into the set if it is not already inside. It…
A: We can make use of contains() method to check whether element is present or not Then we can add item…
Q: Create a method that checks whether two sets of integers are equivalent by writing a function to…
A: As the programming language is not mentioned here, we are using Python
Q: Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets the user play the…
A: 1. Initialize variables: - Set playAgain to true - Create a Random object for generating…
Q: 3. Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to…
A: The solution to the given question is: import java.util.ArrayList;import java.util.Iterator; public…
Q: URLify: Write a method to replace all spaces in a string with '%20'. You may assume that the string…
A: Introduction : In string manipulation problems, a common approach is to edit the string from the end…
Q: JAVA PROGRAM Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets…
A: The algorithm for the code:The user executes the code for the command line argumentThe message asks…
Q: Write a java program that calls a method called removeZeroes that takes as a parameter an ArrayList…
A: Actually, Java is a high level programming language. And also object oriented .It. Is a platform…
Q: 3.9 LAB: Smallest and largest numbers in a list using min and max built-in functions NOTE1: Please…
A: Step 1 : STARTStep 2 : read numbers as long as greater than 0Step 3 : using for, if find the…
Q: Write a method has Twoconsecutive that returns whether or not a list of integers has two adjacent…
A: Given You.are given a solution to the following problem: Write a method hasTwoConsecutive that…
Q: Create a function called find_big_words . The function should accept a list as a parameter. Parts…
A: A list is a type of collection in Python along with tuples and dictionaries. A list can have…
Q: 2. Write a class ArravClass, where the main method asks the user to enter the f num bers. Create a…
A: Java code: import java.util.Scanner;import java.util.Arrays; public class ArrayClass{ public…
Q: Write an application that accepts up to 20 Strings, or fewer if the user enters the terminating…
A: Find the program and output for the given program i.e, the program reads 20 strings until the user…
Q: Using Java: Write the following method that returns the maximum value in an ArrayList of integers.…
A: Write the following method that returns the maximum value in an ArrayList of integers. The method…
Q: Write a java program that calls a method called removeZeroes that takes as a parameter an ArrayList…
A: Step 1 : StartStep 2 : declare all variablesStep 3 : implement removeZero methodStep 4 : enter the…
Q: What is the syntax to call the static method, animalExists? Choose one of the following from the…
A: The problem is based on the basics of classes and objects in java programming language.
Q: QUESTION 2 public static double buyGroceries (String list) a. Identify the return type: b. Identify…
A: The answer of the question 2 is shown below. Answers: a. double b. String c. value-receiving and…
Q: Python help please! Thank you! Add the following methods to your Boat Race class: Write a method…
A: Given, if __name__ == '__main__': the_race = BoatRace('the_big_one.csv')…
Q: mions Add Item. Vien Items. Delete Items and Exit From the program our program and display a list of…
A: Java code: import java.util.*;class items{class node{int data;node link;}node root;void…
Q: 4. This question involves the process of taking a list of words, called wordList, and producing a…
A: In this question we have to write a Java program for the given problem statement of format strings.…
Q: Complete this code You can write a recursive helper method that takes any number of arguments and…
A: Algorithm: Start Implement sum() method that takes a list as an argument and returns sum of all the…
Q: Write this program in Java using a custom method. Implementation details You will implement this…
A: This program uses a do-while loop to run the game engine until the user decides to quit or runs out…
Q: Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets the user play the…
A: Algorithm for Rock, Paper, Scissors Game1. Parse the command line argument to get the seed for the…
Q: abc is an array of integers with N elements. This code fragment finds the index of the first…
A: 1. abc is an array of integers with N elements. This code fragment finds the index of the first…
Q: The two-dimensional arrays m1 and m2 are identicalif they have the same contents. Write a method…
A: Actually, array is a collection of elements. There are three types of arrays 1) one dimensional…
Q: Write a method removeRange that takes two integer parameters, min and max, 7-) and removes all…
A: C program to remove all element from the list in the given range and return the total number of…
Q: zeroTriples.py: Write a program that reads a list of integers from the user, until they enter -12345…
A: Steps =========== Ask user to enter numbers until and put them in list numbers, until user enter…
Q: Task 2: Write a static method whi and changes the ArrayList than the integer argument. tomato cheese…
A: import java.util.*; import java.util.stream.*; class Test { public static void…
Q: Write a void method named MinMaxRange that includes a loop that asks a user to enter a number until…
A: java.util.Scanner is imported for taking input from the user. sc is an object of the Scanner class.…
Write a method that accepts two lists of integer values and checks if they
are identical or not (i.e. contain the same values). if they are not identical, the method
must display the max value of each list.

Step by step
Solved in 2 steps

- Please answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…Chapter 5. PC #17. Rock, Paper, Scissors Game (page 317) Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows. 1. Add the following two lines to the beginning of your main method. This will allow your computer choices match with the test cases here in HyperGrade. long seed = Long.parseLong(args[0]); Random random = new Random(seed); 2. When the program begins, a random number in the range of 0 through 2 is generated. If the number is 0, then the computer has chosen rock. If the number is 1, then the computer has chosen paper. If the number is 2, then the computer has chosen scissors. (Do not display the computer choice yet.) 3. The user enters his or her choice of "rock", "paper", or "scissors" at the keyboard. You should use 1 for rock, 2 for paper, and 3 for scissors. Internally, you can store 0, 1, and 2 as the user choice, to match with the above schema. 4. Both user and computer choices…downvote if wrong
- The play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…Project Description: In this project you implement an ArrayStack ADT and use the stack for implementing the following methods: a) Reverse an array of Words: Accept an array of words as input parameter and return an array of words in reverse order. Use the method signature: public static String[] reverseWords (String[] wordList) Example Input: Bird Cat Dog Elephant Output: Elephant Dog Cat BirdPlease answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…
- 1. Write a method countCommon that accepts two lists of integers as parameters and returns the number of unique integers that occur in both lists. Use one or more sets as storage to help you solve this problem. For example, if one list contains the values [3, 7, 3, –1, 2, 3, 7, 2, 15, 15] and the other list contains the values [–5, 15, 2, –1, 7, 15, 36], your method should return 4 because the elements –1, 2, 7, and 15 occur in both lists. Your method should return correct result with different lists as well. 2. Write a method maxLength that accepts a set of strings as a parameter and that returns the length of the longest string in the list. If your method is passed an empty set, it should return 0.In your project COSC241<Lab4>_<your username>, create Lab4_yourusername.java and declare proper lists and sets to call the two methods countCommon and maxLength. And your output should show the two methods work as expected.Make sure you have comments through your program.For this project you will create a program that populates a list of 8 car manufacturers. You can have theuser populate the list or you can have it predefined.(your choice)If you used a predefined list, then ask the user to add a car manufacturer to the list,Example:cars = ['Ford', 'BMW', 'Volvo']print(‘Enter a new Car Manufacturer: ’)cars.append(input())Use the len() function to acquire the length of the list.(this number is used at the end)Example:cars = ['Ford', 'BMW', 'Volvo']length = cars.len()Use the sort() function to sort the list, (Sort before it is displayed at the end)Example:cars = ['Ford', 'BMW', 'Volvo']cars.sort()and displays the contents of the list using a for loop in the following format:The 9 car manufacturers are:BMWFordVolvoInstructions There are four clubs in Tesset University South, North, West, and East. A Tesset student can choose to join any number of clubs. Your task is to determine how many students are enrolled in exactly two clubs. Write a function/method named dualClubCounter that will accept a string containing the student number and his desired club. Return a set containing the name of students are enrolled exactly in two clubs. Use SET in your solution Example #1 INPUT: "12300:East,12301:West, 12302:South, 12300:West, 123 Expected Content of Set = [12300] Example #2 INPUT: "12300:East,12301:West, 12302:South, 12300:West,123 Expected Content of Set = [12300, 12301]
- Please do not use string methods except to find methodAssume the following method is in class TestSLList (a method outside the SingleLinked List class). public static void test5Question(SingleLinkedList list, int item) { while( list.contains(item) ) list.remove((Integer) item); } a. The method removes the last occurrence of item from "list". b. The method removes all occurrences of item from "1ist". O. The method removes the element at location item from "list". Od. The method removes the first occurrence of item from "list".Which of the above choices (A, B, C or D) prints the name of each animal in the animalList object created from a previous question?// Assume that these accessor and mutator methods exist in the Animal class: // public void setAnimalName(String newName) { ... } // public String getAnimalName() { ... } // A for(int i = 0; i < animalList.length(); i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.getAnimalName()); } // B for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.getAnimalName()); } // C for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println( tempAnimal.setAnimalName()); } // D for(int i = 0; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; System.out.println(tempAnimal.animalName); }
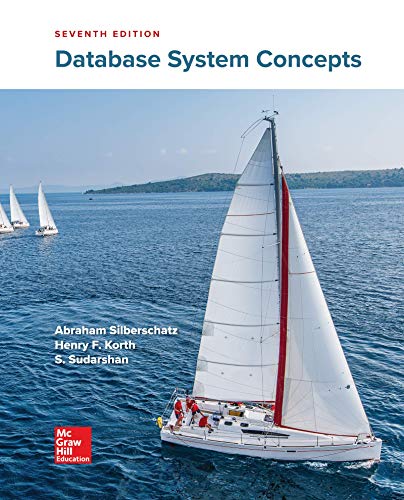
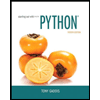
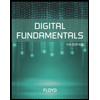
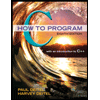
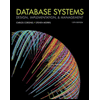
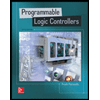
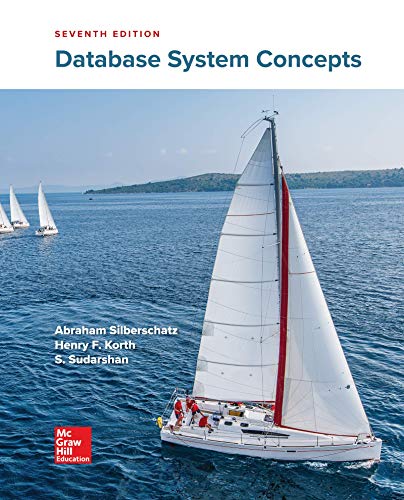
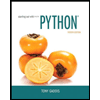
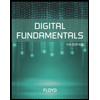
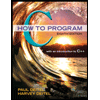
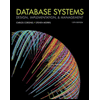
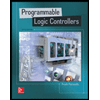