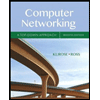
You will write a function that can be used to decrypt Dan’s encrypted text. You will be given an open file that contains an encrypted passage from one of the books, and the name of a file containing a wordlist of English words. You need to discover the shift value to use (0-25) in order to decrypt the text. A correct shift value is one that leads to the maximum number of words being found in the English list of words.
To find the words in the encrypted text, you must call split(). For a given shift value, convert all letters to lowercase, then try to find each word in the English wordlist. Do not remove any punctuation or symbols from the word: for example, if the word is hello!, then that is the exact string, including exclamation mark, that you should try to find in the English wordlist.
Your function should return a string where all letters are in lowercase, and all other characters (newlines, spaces, punctuation, etc.) are retained.
Input
In each test case, we will call your decrypt function with an open file that contains encrypted text, and the name of a file containing an English wordlist.
An encrypted file contains the encrypted text; for example, it might look like this:
Q svme pm nmtb smmvtg bpm ijamvkm wn pqa wev niuqtg.
An English wordlist has one word per line; here is a small example:
abases abash abashed abashes abashing abashment
Return Value
Using the full English wordlist provided in the starter code, your function would return the following string for the above encrypted text:
i knew he felt keenly the absence of his own family.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- I need to modify this code to be able to read from a file and write into a file. The program is working as it should but I need an example of how to change my input and accept a file instead.arrow_forwardcan you do it pythonarrow_forwardUse the functions from the previous three problems to implement a pro-gram that computes the sum of the squares of numbers read from a file. Your program should prompt for a file name and print out the sum of thesquares of the values in the file. Hint: Use readlines ()arrow_forward
- pls code in pythonplagiarism: This Boolean function takes two filenames. If any line occurs in both files, return True.If not, return False. I suggest using nested loops. With nested loops, we call the loop that is in thebody of the other loop, the "inner loop". The loop that contains the inner loop is the "outer loop". Openthe second file inside the outer loop:for line1 in file1:file2 = open(fname2)for line2 in file2:Python only lets you read the file once per open, so you need this order of instructions. Make sure yourreturn False is outside of both loops. Otherwise, you will only compare the first two lines of thefiles. Make sure you close the file that gets read repeatedly after the inner loop but still inside the outerloop.Here is some (more complete) pseudocode to get you started:open file 1for line1 in file 1open file 2for line2 in file 2:if line1 == line2:return Trueclose file 2return Falsearrow_forwardYou are not allowed to use strings, cstrings!!! A C++ Program: We have obtained some files that are encoded with a simple method of writing the text from end to beginning. We therefore need a program that reads a file named codedfile.txt that is 500 chars or less and generates a file call clearfile.txt that has the characters stored in revers order. For example, if codedfile.txt contains the characters dlrow olleh, then after running the program clearfile.txt should contain hello world. Do not use strings or cstrings for reasons explained please!arrow_forwardin the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forward
- Need help with this python problem.arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardIn this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forward
- Counting the character occurrences in a file For this task you are asked to write a program that will open a file called "story.txt" and count the number of occurrences of each letter from the alphabet in this file. At the end your program will output the following report: Number of occurrences for the alphabets: a was used – times. b was used – times. c was used – times.... .and so, on Assume the file contains only lower-case letters and for simplicity just a single paragraph. Your program should keep a counter associated with each letter of the alphabet (26 counters) [Hint: Use array] Your program should also print a histogram of characters count by adding a new function print Histogram (int counters []). This function receives the counters from the previous task and instead of printing the number of times each character was used, prints a histogram of the counters. An example histogram for three letters is shown below) [Hint: Use the extended asci character 254]: A…arrow_forwardIn Python, Create a program that will write 100 integers created randomly in a file. The integers will be separated by a space in the file. Read the data back from the file, and display the sorted data. The program should prompt the user to enter a file name. Utilize the following function headers for this problem: Main() WriteNumbers(filename) ReadNumbers(filename) The main function will first prompt the user to enter the filename. Then the main function calls WriteNumbers-then ReadNumbers. The WriteNumbers function opens an output file and writes 100 random numbers as long a large string text. Do not use lists for this problem- please just write a random number followed by a space 100 times. The ReadNumbers function will then read the text file and display the numbers sorted. In order to sort the numbers, read the big string and then split it into a list. Now convert them into integers by using list comprehension and then sort the list. Loop through the list and print each…arrow_forwardThis is for r Regarding a for loop, which of the following is not true? The for keyword must be followed by parentheses. Following the for statement, the code to be executed is recognized by it being indented. The number of times the loop will be performed is determined by the length of the vector passed to the for keyword. The for loop can contain other for loops.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
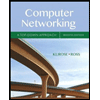
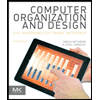
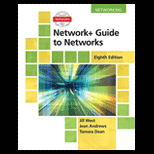
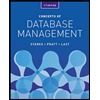
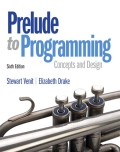
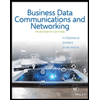