Given a file with data for names and marks you will read them into two arrays You will then display the data, do a linear search and report if found, sort the data, do a binary search. Be sure to test for found and not found in your main program. Read Data Write a function that reads in data from a file using the prototype below. The function can define and open the file, read the names and marks into the arrays and keep track of how many names there are in numElts, and then closes the file. The list of names and marks can be found at the end of the lab and copied into a text file. void getNames(string names[], int marks[], int& numElts); Display Data Write a function to display the contents of names and marks using the prototype given below. void displayData(const string names[], const int marks[], int numElts); Linear Search Write the searchList function given the prototype below so that it searches for a given name. The functions returns and int which is the index of the name found. The main program will decide if -1 is returned then it will say name is not found otherwise it will write out the name and the mark for that name. int linearSearch(const string list[], int numElts, string value); Selection Sort Write the selectionSort given the prototype below so that it sorts by name in ascending order. Be sure to accept both arrays name and mark for sorting purposes. void selectionSort(string name[], int marks[], int numElts); Binary Search Write the binarySearch function given the prototype below so that it searches for a given name.The functions returns and int which is the index of the name found. The main program will decide if -1 is returned then it will say name is not found otherwise it will write out the name and the mark for that name. int binarySearch(const stringt array[], int numElts, string value); Main Function Write a main function that declares the names, marks, number of elements as well as the value to be searched for and the index of the returned function calls. Read and display the contents of names and marks. Ask the user for a name and using the linear search return the index to the user. If -1 is returned then the name is not in the file. Otherwise write out the name and mark for that student. Sort the arrays and write them out. Ask the user for a name to search for. This time use the binarySearch to return -1 or an index. Display the student’s name and mark if found. Test your program for found and not found for each type of search. void getNames(string names[], int marks[], int& numElts); int linearSearch(const string names[], int numElts,string who); int binarySearch(const string names[], int numElts,string who); void selectionSort(string names[], int marks[],int numElts); void displayData(const string names[], const int marks[], int numElts); const int NUM_Names = 20; int main() { string names[NUM_NAMES]; int marks[NUM_NAMES]; int numElts; int index; string searchWho; // Function calls here return 0; } File for names and marks: Collins,Bill 80 Smith,Bart 75 Allen,Jim 82 Griffin,Jim 55 Stamey,Marty 90 Rose,Geri 78 Taylor,Terri 56 Johnson,Jill 77 Allison,Jeff 45 Looney,Joe 89 Wolfe,Bill 63 James,Jean 72 Weaver,Jim 77 Pore,Bob 91 Rutherford,Greg 42 Javens,Renee 74 Harrison,Rose 58 Setzer,Cathy 93 Pike,Gordon 48 Holland,Beth 79 this is one whole question or program not a multipart question please answer all of it, thanks!! answer in beginner C++,
Given a file with data for names and marks you will read them into two arrays You will then display the data, do a linear search and report if found, sort the data, do a binary search. Be sure to test for found and not found in your main program.
- Read Data
Write a function that reads in data from a file using the prototype below. The function can define and open the file, read the names and marks into the arrays and keep track of how many names there are in numElts, and then closes the file. The list of names and marks can be found at the end of the lab and copied into a text file.
void getNames(string names[], int marks[], int& numElts);
- Display Data
Write a function to display the contents of names and marks using the prototype given below.
void displayData(const string names[], const int marks[], int numElts);
- Linear Search
Write the searchList function given the prototype below so that it searches for a given name. The functions returns and int which is the index of the name found.
The main program will decide if -1 is returned then it will say name is not found otherwise it will write out the name and the mark for that name.
int linearSearch(const string list[], int numElts, string value);
- Selection Sort
Write the selectionSort given the prototype below so that it sorts by name in ascending order. Be sure to accept both arrays name and mark for sorting purposes.
void selectionSort(string name[], int marks[], int numElts);
- Binary Search
Write the binarySearch function given the prototype below so that it searches for a given name.The functions returns and int which is the index of the name found.
The main program will decide if -1 is returned then it will say name is not found otherwise it will write out the name and the mark for that name.
int binarySearch(const stringt array[], int numElts, string value);
- Main Function
Write a main function that declares the names, marks, number of elements as well as the value to be searched for and the index of the returned function calls.
- Read and display the contents of names and marks.
- Ask the user for a name and using the linear search return the index to the user. If -1 is returned then the name is not in the file. Otherwise write out the name and mark for that student.
- Sort the arrays and write them out.
- Ask the user for a name to search for. This time use the binarySearch to return -1 or an index. Display the student’s name and mark if found.
- Test your program for found and not found for each type of search.
void getNames(string names[], int marks[], int& numElts);
int linearSearch(const string names[], int numElts,string who);
int binarySearch(const string names[], int numElts,string who);
void selectionSort(string names[], int marks[],int numElts);
void displayData(const string names[], const int marks[], int numElts);
const int NUM_Names = 20;
int main()
{
string names[NUM_NAMES];
int marks[NUM_NAMES];
int numElts;
int index;
string searchWho;
// Function calls here
return 0;
}
File for names and marks:
Collins,Bill 80
Smith,Bart 75
Allen,Jim 82
Griffin,Jim 55
Stamey,Marty 90
Rose,Geri 78
Taylor,Terri 56
Johnson,Jill 77
Allison,Jeff 45
Looney,Joe 89
Wolfe,Bill 63
James,Jean 72
Weaver,Jim 77
Pore,Bob 91
Rutherford,Greg 42
Javens,Renee 74
Harrison,Rose 58
Setzer,Cathy 93
Pike,Gordon 48
Holland,Beth 79
this is one whole question or program not a multipart question please answer all of it, thanks!!
answer in beginner C++,

Step by step
Solved in 2 steps

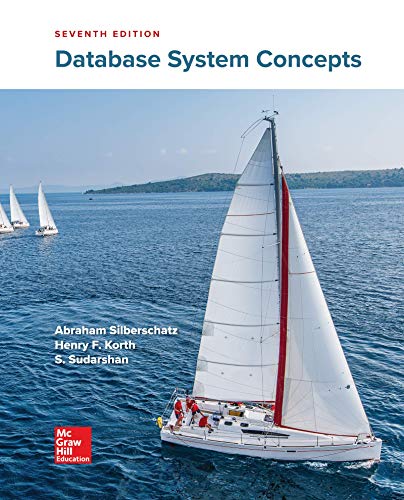
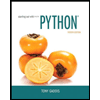
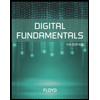
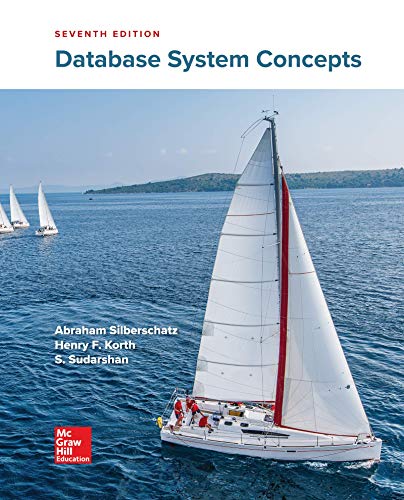
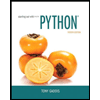
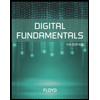
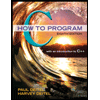
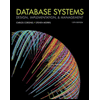
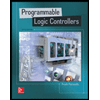