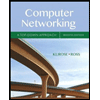
It is python language
Write the code that creates a new Node class. It will store data and next attributes. You only need to create the __init__ method.
data and next variables will have default values, both set to None.
Assume you are using the Node class from the previous connection to create a LinkedList. You have the code below, create a method that removes the first node from the LinkedList.
class LinkedList:
def __init__(self):
self.head = None
Based on the code from the last two questions, create a new LinkedList. Add 2 values to the LinkedList (there is an add method that accepts data as an argument, called add). Then call the removeFront method created in the previous question.
Based on the previous questions, create a Queue class that uses the LinkedList for its data storage. Create the __init__, isEmpty, insert, remove, and size methods. Assume that LinkedList class has the add, removeFront and size methods defined.
Based on the LinkedList code already created previous, write a function that will insert a new value into the middle of a Linked List.
INPUT: The head of the Linked List, the value to insert
OUTPUT: Nothing is output
RETURNED: Nothing is returned

Step by stepSolved in 2 steps

- Given main.py and a Node class in Node.py, complete the LinkedList class (a linked list of nodes) in LinkedList.py by writing the insert_in_ascending_order() method that inserts a new Node into the LinkedList in ascending order. Ex: If the input is: 8 3 6 2 5 9 4 1 7 the output is: 1 2 3 4 5 6 7 8 9 Code givenarrow_forwardThis is a python programming question Python Code: class Node: def __init__(self, initial_data): self.data = initial_data self.next = None class LinkedList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node self.tail = new_node def prepend(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: new_node.next = self.head self.head = new_node def insert_after(self, current_node, new_node): if self.head == None: self.head = new_node self.tail = new_node elif current_node is self.tail: self.tail.next = new_node self.tail = new_node else: new_node.next = current_node.next…arrow_forwardQUESTION 11 You need to store a list of messages so that the most recently-added message is always first. Should you use an ArrayList or a LinkedList? It doesn't matter in this case. Linked List ArrayList QUESTION 12 You need to store a collection of employees in a big company so that you can look them up by name. Should you use an List, a Map, or or a Set? O It doesn't matter in this case. List Set Maparrow_forward
- Based on the previous questions, create a Queue class that uses the LinkedList for its data storage. Create the __init__, isEmpty, insert, remove, and size methods. Assume that LinkedList class has the add, removeFront and size methods defined.arrow_forwardlinked_list_stack_shopping_list_manager.py This is a file that includes the class of linked list based shopping list manager. This class contains such methods as init, insert_item, is_list_empty, print_item_recursive_from_top, print_items_from_top, print_item_recursive_from_bottom, print_items_from_bottom, getLastItem, removeLastItem. In addition, this class requires the inner class to hold onto data as a linked list based on Stack. DO NOT use standard python library, such as deque from the collections module. Please keep in mind the following notes for each method during implementation: Init(): initializes linked list based on Stack object to be used throughout object life. insert_item(item): inserts item at the front of the linked list based on Stack. Parameters: item name. is_list_empty (): checks if the current Stack object is empty (ex. check if head is None). print_item_recursive_from_top(currentNode): a helper method to print linked list based on Stack item recursively. Note:…arrow_forwardA map has the form Map <k,v> where: K: specifies the type of keys maintained in this map.V: defines the type of mapped values.Furthermore, the Map interface provides a set of methods that must be implemented. In this section, we will discuss about the most famous methods: clear: Removes all the elements from the map.containsKey: Returns true if the map contains the requested key.containsValue: Returns true if the map contains the requested value.equals: Compares an Object with the map for equality.get: Retrieve the value of the requested key.entrySet: Returns a Set view of the mappings contained in this map.keySet: Returns a Set that contains all keys of the map.put: Adds the requested key-value pair in the map.remove: Removes the requested key and its value from the map, if the key exists.size: Returns the number of key-value pairs currently in the map. Here is an example of TreeMap with a Map: import java.util.Map; import java.util.TreeMap; public class TreeMapExample {…arrow_forward
- class Node: def __init__(self, data): self.data = data self.next = None self.prev = None # Empty Doubly Linked Listclass DoublyLinkedList: def __init__(self): self.head = None def append(self, new_data): if self.head == None: self.head = new_data self.tail = new_data else: self.tail.next = new_data new_data.prev = self.tail self.tail = new_data def printList(self): if(self.head == None): print('Empty!') else: node = self.head while(node is not None): print(node.data), node = node.next def remove(self, current_node): successor_node = current_node.next predecessor_node = current_node.prev if successor_node is not None: successor_node.prev = predecessor_node if predecessor_node is not None: predecessor_node.next = successor_node if…arrow_forwardData Structures/Algorithms in Javaarrow_forwardcreating tree containers: one that uses a vector to hold your trees (class VectorContainer). Each of these container classes should be able to hold any amount of different expressions each of which can be of any size. see example. Please create vectorContainer.hpp all I need sort.hpp #ifndef __SORT_HPP__#define __SORT_HPP__ #include "container.hpp" class Container; class Sort {public:/* Constructors */Sort(); /* Pure Virtual Functions */virtual void sort(Container* container) = 0;}; #endif //__SORT_HPP__ base.hpp #ifndef __BASE_HPP__#define __BASE_HPP__ #include <string> class Base {public:/* Constructors */Base() { }; /* Pure Virtual Functions */virtual double evaluate() = 0;virtual std::string stringify() = 0;}; #endif //__BASE_HPP__ container.hpp #ifndef __CONTAINER_HPP__#define __CONTAINER_HPP__ #include "sort.hpp"#include "base.hpp" class Sort;class Base; class Container {protected:Sort* sort_function; public:/* Constructors */Container() : sort_function(nullptr) {…arrow_forward
- The numbers on the left margin denote line numbers. line#01 class LL_node {02 int val;03 LL_node next;04 public LL_node (int n) {05 val = n;06 next = null;07 }08 public void set_next (LL_node nextNode) {09 next = nextNode;10 }11 public LL_node get_next () {12 return next;13 }14 public void set_value (int input) {15 val = input;16 }17 public int get_value () {18 return val;19 }20 }21 public class LL {22 protected LL_node head;23 protected LL_node tail;24 public LL () {25 head = null;26 tail = null;27 }28 public int append (int n) {29 if (head == null) {30 head = new LL_node(n);31 tail = head;32 } else {33 LL_node new_node;34 new_node = new LL_node(n);35 tail.set_next (new_node);36 tail = new_node;37 }38 return n;39 }40 } which the following statement…arrow_forward1. Goals and Overview The goal of this assignment is to practice creating a hash table. This assignment also has an alternative, described in Section 5. A hash table stores values into a table (meaning an array) at a position determined by a hash function. 2. Hash Function and Values Every Java object has a method int hashCode() which returns an int suitable for use in a hash table. Most classes use the hashCode method they inherit from object, but classes such as string define their own hashCode method, overriding the one in object. For this assignments, your hash table stores values of type public class HashKeyValue { K key; V value; } 3. Hash Table using Chained Hashing Create a hash table class (called HashTable211.java) satisfying the following interface: public interface HashInterface { void add(K key, V value); // always succeeds as long as there is enough memory V find (K key); // returns null if there is no such key } Internally, your hash table is defined as a private…arrow_forwardcreating tree containers: one that uses a vector to hold your trees (class VectorContainer). Each of these container classes should be able to hold any amount of different expressions each of which can be of any size. see example. Please create vectorContainer.hpp all I need sort.hpp #ifndef __SORT_HPP__#define __SORT_HPP__ #include "container.hpp" class Container; class Sort {public:/* Constructors */Sort(); /* Pure Virtual Functions */virtual void sort(Container* container) = 0;}; #endif //__SORT_HPP__ base.hpp #ifndef __BASE_HPP__#define __BASE_HPP__ #include <string> class Base {public:/* Constructors */Base() { }; /* Pure Virtual Functions */virtual double evaluate() = 0;virtual std::string stringify() = 0;}; #endif //__BASE_HPP__ container.hpp #ifndef __CONTAINER_HPP__#define __CONTAINER_HPP__ #include "sort.hpp"#include "base.hpp" class Sort;class Base; class Container {protected:Sort* sort_function; public:/* Constructors */Container() : sort_function(nullptr) {…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
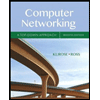
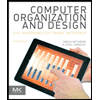
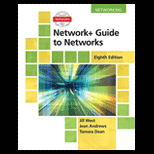
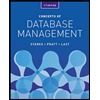
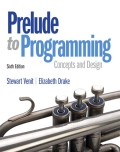
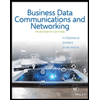