A map has the form Map where: K: specifies the type of keys maintained in this map. V: defines the type of mapped values. Furthermore, the Map interface provides a set of methods that must be implemented. In this section, we will discuss about the most famous methods: clear: Removes all the elements from the map. containsKey: Returns true if the map contains the requested key. containsValue: Returns true if the map contains the requested value. equals: Compares an Object with the map for equality. get: Retrieve the value of the requested key. entrySet: Returns a Set view of the mappings contained in this map. keySet: Returns a Set that contains all keys of the map. put: Adds the requested key-value pair in the map. remove: Removes the requested key and its value from the map, if the key exists. size: Returns the number of key-value pairs currently in the map. Here is an example of TreeMap with a Map: import java.util.Map; import java.util.TreeMap; public class TreeMapExample { public static void main(String[] args) { Map vehicles = new TreeMap<>(); // Add some vehicles. vehicles.put("BMW", 5); vehicles.put("Mercedes", 3); vehicles.put("Audi", 4); vehicles.put("Ford", 10); System.out.println("Total vehicles: " + vehicles.size()); // Iterate over all vehicles, using the keySet method. for (String key : vehicles.keySet()) System.out.println(key + " - " + vehicles.get(key)); System.out.println(); System.out.println("Highest key: " + ((TreeMap) vehicles).lastKey()); System.out.println("Lowest key: " + ((TreeMap) vehicles).firstKey()); System.out.println("\nPrinting all values:"); for (Integer val : vehicles.values()) System.out.println(val); System.out.println(); // Clear all values. vehicles.clear(); // Equals to zero. System.out.println("After clear operation, size: " + vehicles.size()); } } The suspected output looks like this: Total vehicles: 4 Audi - 4 BMW - 5 Ford - 10 Mercedes - 3 Highest key: Mercedes Lowest key: Audi Printing all values: 4 5 10 3 After clear operation, size: 0 Press any key to continue . . .
A map has the form Map <k,v> where:
K: specifies the type of keys maintained in this map.
V: defines the type of mapped values.
Furthermore, the Map interface provides a set of methods that must be implemented. In this section, we will discuss about the most famous methods:
clear: Removes all the elements from the map.
containsKey: Returns true if the map contains the requested key.
containsValue: Returns true if the map contains the requested value.
equals: Compares an Object with the map for equality.
get: Retrieve the value of the requested key.
entrySet: Returns a Set view of the mappings contained in this map.
keySet: Returns a Set that contains all keys of the map.
put: Adds the requested key-value pair in the map.
remove: Removes the requested key and its value from the map, if the key exists.
size: Returns the number of key-value pairs currently in the map.
Here is an example of TreeMap with a Map:
import java.util.Map;
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String[] args) {
Map<String, Integer> vehicles = new TreeMap<>();
// Add some vehicles.
vehicles.put("BMW", 5);
vehicles.put("Mercedes", 3);
vehicles.put("Audi", 4);
vehicles.put("Ford", 10);
System.out.println("Total vehicles: " + vehicles.size());
// Iterate over all vehicles, using the keySet method.
for (String key : vehicles.keySet())
System.out.println(key + " - " + vehicles.get(key));
System.out.println();
System.out.println("Highest key: " + ((TreeMap) vehicles).lastKey());
System.out.println("Lowest key: " + ((TreeMap) vehicles).firstKey());
System.out.println("\nPrinting all values:");
for (Integer val : vehicles.values())
System.out.println(val);
System.out.println();
// Clear all values.
vehicles.clear();
// Equals to zero.
System.out.println("After clear operation, size: " + vehicles.size());
}
}
The suspected output looks like this:
Total vehicles: 4
Audi - 4
BMW - 5
Ford - 10
Mercedes - 3
Highest key: Mercedes
Lowest key: Audi
Printing all values:
4
5
10
3
After clear operation, size: 0
Press any key to continue . . .
Assume that we have an actual FactBook2008.txt which you need to read. We want to create a wordcount map made out of K=String, and V=Integer. The program is to count the frequency of words in the above file. Basically, if the word shows up for the first time we count it as being 1. If we have encountered already we increment the word count by 1.
We would like to print the words in the files and count how many of them we read.Hint: Use the following loop to print the output as follows:
for(String word : wordCount.keySet())
System.out.println(word + " " + wordCount.get(word));

Step by step
Solved in 2 steps

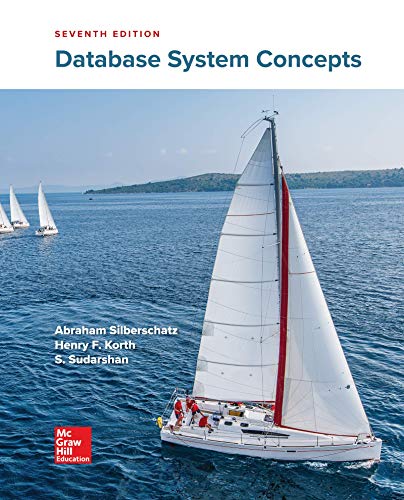
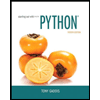
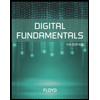
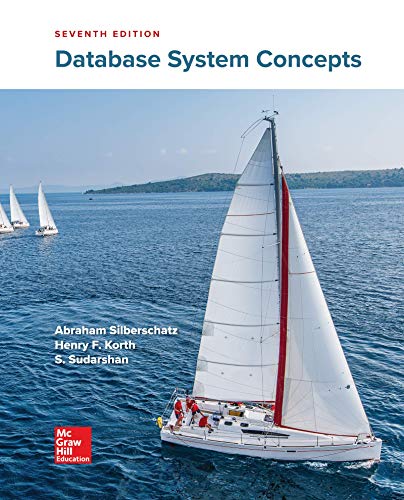
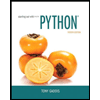
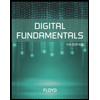
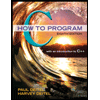
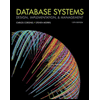
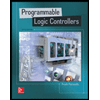