Write an interface and then modify classes to implement the interface. Write an interface, GeometricSolid, which has one method, volume. The volume method takes no arguments and returns a double. You are provided with three classes: Cylinder, Sphere, and RightCircularCone Modify the classes so that they implement the GeometricSolid interface. Supply the appropriate method for each class. Make sure that you use Math.PI in your calculations. Notice in InterfaceRunner that the objects are added to an ArrayList of GeometricSolids. Cylinder.java /** * Models a Cylinder */ public class Cylinder { private double radius; private double height; /** * Constructor for objects of class Cylinder * @param radius the radius of the Cylinder * @param height the height of this Cylinder */ public Cylinder(double radius, double height) { this.radius = radius; this.height = height; } /** * Gets the radius of this Cylinder * @return the radius of the Cylinder */ public double getRadius() { return radius; } /** * Sets the radius of the Cylinder * @param newRadius the value of the new radius */ public void setRadius(int newRadius) { radius = newRadius; } /** * Gets the height of this Cylinder * @return the height of the Cylinder */ public double getHeight() { return height; } /** * Sets the height of the Cylinder * @param newHeight the value of the new height */ public void setHeight(int newHeight) { height = newHeight; } Write Override method here } GeometricSolid.java Write GeometricSolid interface here RightCircularCone.java /** * Models a Right circular cone */ public class RightCircularCone { private double radius; private double height; /** * Constructor for objects of class RightCircularCone * @param radius the radius of the RightCircularCone * @param height the height of this RightCircularCone */ public RightCircularCone(double radius, double height) { this.radius = radius; this.height = height; } /** * Gets the radius of this RightCircularCone * @return the radius of the RightCircularCone */ public double getRadius() { return radius; } /** * Sets the radius of the RightCircularCone * @param newRadius the value of the new radius */ public void setRadius(double newRadius) { radius = newRadius; } /** * Gets the height of this RightCircularCone * @return the height of the RightCircularCone */ public double getHeight() { return height; } /** * Sets the height of the RightCircularCone * @param newHeight the value of the new height */ public void setHeight(double newHeight) { height = newHeight; } Write Override method here } Sphere.java /** * Models a sphere in 3-d space */ public class Sphere { private double radius; /** * Constructor for objects of class Sphere * @param radius the radius of this Sphere */ public Sphere(double radius) { this.radius = radius; } /** * Gets the radius of this Sphere * @return the radius of this Sphere */ public double getRadius() { return radius; } /** * Sets the the radius of this Sphere * @param newRadius the radius of this Sphere */ public void setRadius(double newRadius) { radius = newRadius; } Write Override method here }
Write an interface and then modify classes to implement the interface. Write an interface, GeometricSolid, which has one method, volume. The volume method takes no arguments and returns a double.
You are provided with three classes: Cylinder, Sphere, and RightCircularCone Modify the classes so that they implement the GeometricSolid interface. Supply the appropriate method for each class. Make sure that you use Math.PI in your calculations.
Notice in InterfaceRunner that the objects are added to an ArrayList of GeometricSolids.
Cylinder.java
/**
* Models a Cylinder
*/
public class Cylinder
{
private double radius;
private double height;
/**
* Constructor for objects of class Cylinder
* @param radius the radius of the Cylinder
* @param height the height of this Cylinder
*/
public Cylinder(double radius, double height)
{
this.radius = radius;
this.height = height;
}
/**
* Gets the radius of this Cylinder
* @return the radius of the Cylinder
*/
public double getRadius()
{
return radius;
}
/**
* Sets the radius of the Cylinder
* @param newRadius the value of the new radius
*/
public void setRadius(int newRadius)
{
radius = newRadius;
}
/**
* Gets the height of this Cylinder
* @return the height of the Cylinder
*/
public double getHeight()
{
return height;
}
/**
* Sets the height of the Cylinder
* @param newHeight the value of the new height
*/
public void setHeight(int newHeight)
{
height = newHeight;
}
Write Override method here
}
GeometricSolid.java
Write GeometricSolid interface here
RightCircularCone.java
/**
* Models a Right circular cone
*/
public class RightCircularCone
{
private double radius;
private double height;
/**
* Constructor for objects of class RightCircularCone
* @param radius the radius of the RightCircularCone
* @param height the height of this RightCircularCone
*/
public RightCircularCone(double radius, double height)
{
this.radius = radius;
this.height = height;
}
/**
* Gets the radius of this RightCircularCone
* @return the radius of the RightCircularCone
*/
public double getRadius()
{
return radius;
}
/**
* Sets the radius of the RightCircularCone
* @param newRadius the value of the new radius
*/
public void setRadius(double newRadius)
{
radius = newRadius;
}
/**
* Gets the height of this RightCircularCone
* @return the height of the RightCircularCone
*/
public double getHeight()
{
return height;
}
/**
* Sets the height of the RightCircularCone
* @param newHeight the value of the new height
*/
public void setHeight(double newHeight)
{
height = newHeight;
}
Write Override method here
}
Sphere.java
/**
* Models a sphere in 3-d space
*/
public class Sphere
{
private double radius;
/**
* Constructor for objects of class Sphere
* @param radius the radius of this Sphere
*/
public Sphere(double radius)
{
this.radius = radius;
}
/**
* Gets the radius of this Sphere
* @return the radius of this Sphere
*/
public double getRadius()
{
return radius;
}
/**
* Sets the the radius of this Sphere
* @param newRadius the radius of this Sphere
*/
public void setRadius(double newRadius)
{
radius = newRadius;
}
Write Override method here
}


Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 9 images

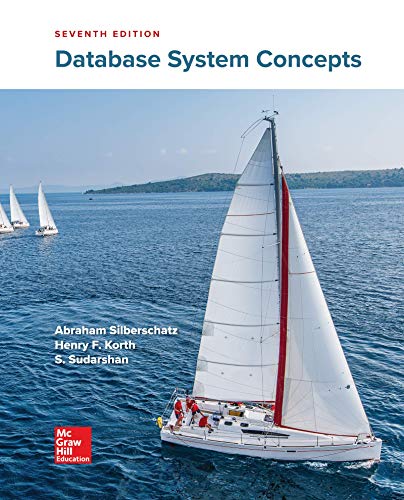
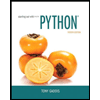
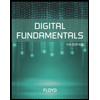
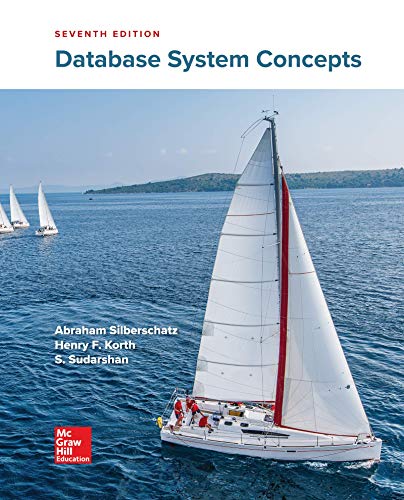
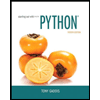
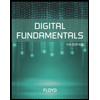
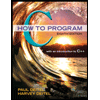
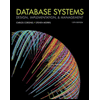
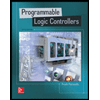