JAVA In order to compute a power of two, you can take the next-lower power and double it. For example, if you want to compute 2^11 and you know that 2^10=1024, then 2^11=2×1024=2048. Write a recursive method public static int pow2(int n) where n is the exponent, that is based on this observation. If the exponent is negative, return -1. import java.util.Scanner; public class PowerTwo { public static int pow2(int n) { /* code goes here */ } public static void main(String[] args) { Scanner in = new Scanner(System.in); while (in.hasNextInt()) { int exponent = in.nextInt(); System.out.println(pow2(exponent)); } } }
JAVA In order to compute a power of two, you can take the next-lower power and double it. For example, if you want to compute 2^11 and you know that 2^10=1024, then 2^11=2×1024=2048. Write a recursive method public static int pow2(int n) where n is the exponent, that is based on this observation. If the exponent is negative, return -1. import java.util.Scanner; public class PowerTwo { public static int pow2(int n) { /* code goes here */ } public static void main(String[] args) { Scanner in = new Scanner(System.in); while (in.hasNextInt()) { int exponent = in.nextInt(); System.out.println(pow2(exponent)); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JAVA
In order to compute a power of two, you can take the next-lower power and double it. For example, if you want to compute 2^11 and you know that 2^10=1024, then 2^11=2×1024=2048. Write a recursive method
public static int pow2(int n)where n is the exponent, that is based on this observation. If the exponent is negative, return -1.
import java.util.Scanner;
public class PowerTwo
{
public static int pow2(int n)
{
/* code goes here */
}
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
while (in.hasNextInt())
{
int exponent = in.nextInt();
System.out.println(pow2(exponent));
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
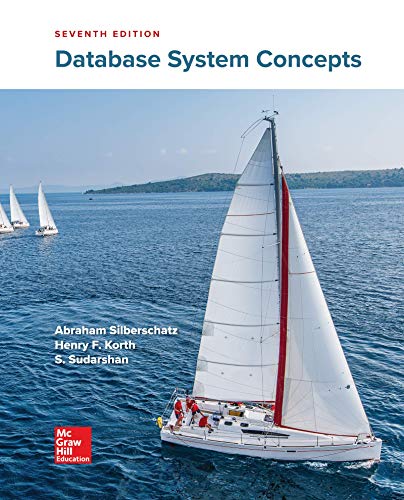
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
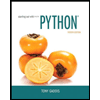
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
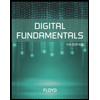
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
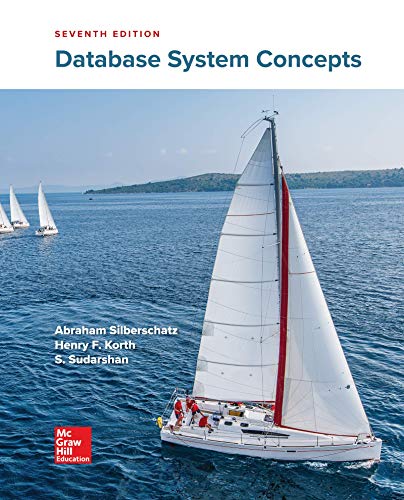
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
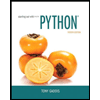
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
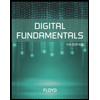
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
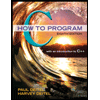
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
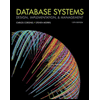
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
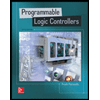
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education