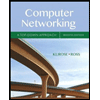
Java
Lab 5 Problem Statement
Write a
What is the capital of Alabama? Montogomery The correct answer is Montgomery. What is the capital of Alaska? juneau That is the correct answer! ... You got 32 out of 50 correct! |
The state capitals will be stored in a 2D String array of size 2 x 50, with the first column the name of the state and the second the name of the capital city, for all 50 states. To create the state capitals array, follow these instructions:
- Copy the String from the attached file into your program (found below these instructions). The String is a pipe delimited list of states and capitals listed as state|capital|state|capital|... The list is alphabetical.
- Read the String into a Scanner object using something like: Scanner scanStr = new Scanner(stateCapitals);
- You must set the delimiter for the scanner before using it, before the loop: scanStr.useDelimiter("\\|");
- Create the necessary 2D array by declaring the array and then using a for loop to scan each element using scanStr.next() .
- You must also close the scanner when you're done with it, after the loop: scanStr.close();
- Text file with stateCapitals String:
- String stateCapitals = "Alabama|Montgomery|Alaska|Juneau|Arizona|" +
"Phoenix|Arkansas|Little Rock|California|Sacramento|Colorado|" +
"Denver|Connecticut|Hartford|Delaware|Dover|Florida|" +
"Tallahassee|Georgia|Atlanta|Hawaii|Honolulu|Idaho|" +
"Boise|Illinois|Springfield|Indiana|Indianapolis|Iowa|Des Moines|"
+
"Kansas|Topeka|Kentucky|Frankfort|Louisiana|Baton Rouge|" +
"Maine|Augusta|Maryland|Annapolis|Massachusetts|Boston|" +
"Michigan|Lansing|Minnesota|St. Paul|Mississippi|Jackson|" +
"Missouri|Jefferson City|Montana|Helena|Nebraska|Lincoln|" +
"Nevada|Carson City|New Hampshire|Concord|New Jersey|" +
"Trenton|New Mexico|Santa Fe|New York|Albany|North Carolina|" +
"Raleigh|North Dakota|Bismarck|Ohio|Columbus|Oklahoma|" +
"Oklahoma City|Oregon|Salem|Pennsylvania|Harrisburg|" +
"Rhode Island|Providence|South Carolina|Columbia|South Dakota|" +
"Pierre|Tennessee|Nashville|Texas|Austin|Utah|Salt Lake City|" +
"Vermont|Montpelier|Virginia|Richmond|Washington|Olympia|" +
"West Virginia|Charleston|Wisconsin|Madison|Wyoming|Cheyenne";
The entire program can be written in the main class, but you must modularize your work into appropriate methods that are called from main. You will need the following:
- String[][] getData() - a method that creates and returns the 2D array of states and capitals as described above.
- int play(String[][] data) - a method that presents each state to the user, reads in their capital city, displays if they are correct or not, as shown above, and keeps count of and returns how many correct answers there are.
- void showResult(int numberCorrect) - a method that displays to the user how many capitals they got correct after they have gone through all 50 states.
Notes:
- Do not use any global variables.
- Be sure to test your code with states that have a multi-word capital cities, like Little Rock or Salt Lake City.
- Remember to make the answers case-insensitive, so that Olympia, olympia, and OLYMPIA are all good answers to the question "What is the capital of Washington?"

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- JAVA PROGRAMMING LAB Write a Java program to takes 2 numbers from the user and print true if one or the other is teen, but not both. "teen" number is that number which lies in the range 13..19 inclusive.arrow_forwardC Language - Write a program that takes in three integers and outputs the largest value. If the input integers are the same, output the integers' value.arrow_forwardChapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a code: please modify this code so it works in Hypergrade when I submit it so it passes all the test casses in Hypergrade. It has to pass all the test casses in Hypergrade becausw when I submit the code in Hypercase it does not run and it says 0 out of 5 casses passed. Please fix this code. I do not need thanks for playing or…arrow_forward
- Student name: CIS 232 Introduction to Programming Homework Assignment 10 Due Date: 11/23/2020 Instructor: Dr. Lomako Problem Statement: Write a Java program with the main method and a multiplication method (must be created, not the library method). Create “HW10_lastname.java” program that: Prints a program title Creates two n x n square matrices A and B and initializes them with random values Displays the matrices A and B Calls the multiplication method that multiplies two square matrices and returns a matrix AB that is the result of the multiplication. Prints AB matrixarrow_forwardWarm-up: Integer Operations Write a program that asks the user to enter two integers and prints the Difference, Product, Area of an Ellipse, and Average of these numbers. Here is an example of the execution of the program:arrow_forwardJava example to check if number is palidrom or not Number will be entered by the userarrow_forward
- in Java languagearrow_forwardProgram Unit Score Calculator Console App Write a Python Console Application program that allows the user to enter the marks for different assessments in a unit, and computes the total mark and grade for the unit. Here is the program logic specification: There are six assessment activities Quiz1, Quiz2, Quiz3, Quiz4, Lab Journal, Major Assignment and Final Exam. The four quizzes are worth 5 marks each, the Lab Journal is worth 10 marks, the Major Assignment is worth 30 marks and the Final Exam is worth 40 marks. The algorithm for computing the total mark for the unit is: Total Mark = Quiz1+Quiz2+Quiz3+Quiz4+Major Assignment+ Lab Journal + Final Exam The following screenshot shows a successful test run:arrow_forwardChapter 5. PC #2. Retail Price Calculator (page 312) Write a program that asks the user to enter an item’s wholesale cost and its markup percentage. It should then display the item’s retail price. For example: • If an item’s wholesale cost is 5.00 and its markup percentage is 100 percent, then the item’s retail price is 10.00. • If an item’s wholesale cost is 5.00 and its markup percentage is 50 percent, then the item’s retail price is 7.50. The program should have a method named calculateRetail that receives the wholesale cost and the markup percentage as arguments, and returns the retail price of the item. Class name: RetailPriceCalculator Here is a working code but please fix it so it will in Hypergrade which as all the test casses. I DO NOT NEED THANK YOU IN THE PROGRAM. IT HAS TO PASS ALL THE TEST CASSES PLEASE. THANK YOU!!!!!: import java.util.*;class RetailPriceCalculator{ // creating a method public static double calculateRetail(double wholesale,double…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
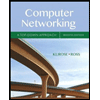
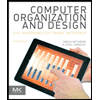
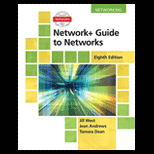
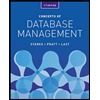
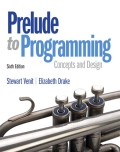
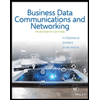