write a program that creates a linked list of points in an x-y coordinate system. Following are the node content of your linked list: head -> P1(0,1) -> P2(1,2) -> P3(10,11) -> P4(0,3) -> P5(17, 16) -> P6(0,0) -> P7(3,4) -> P8(16,1) ->tail Where Pz(x,y) is point z with x and y coordinates in the 2D space. Write a method searchList that recursively searches the linked list of point objects for a specified point w with x1 and y1 cooredinates: Pw(x1, y1). Method searchList should return true if it is found; otherwise, false should be returned. Use your method in a test program that creates the above list. The program should prompt the user for a value to locate in the list. For example: Enter two integer values representing the x and y coordinate of the point you want to look for in the list: 3 4 This point is found Anothet example: Enter two integer values representing the x and y coordinate of the point you want to look for in the list: 0 1 The point does not exist.
write a
Where Pz(x,y) is point z with x and y coordinates in the 2D space.
Write a method searchList that recursively searches the linked list of point objects for a specified point w with x1 and y1 cooredinates: Pw(x1, y1). Method searchList should return true if it is found; otherwise, false should be returned. Use your method in a test program that creates the above list. The program should prompt the user for a value to locate in the list. For example: Enter two integer values representing the x and y coordinate of the point you want to look for in the list:
3 4
This point is found
Anothet example: Enter two integer values representing the x and y coordinate of the point you want to look for in the list:
0 1
The point does not exist.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

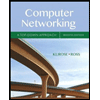
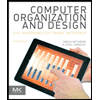
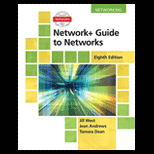
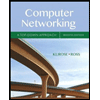
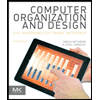
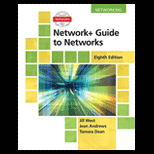
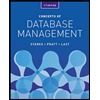
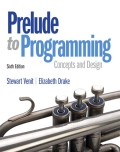
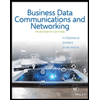