A student implementing a linked list wrote this method: 1 bool LinkedList::Remove(int e) { 2 1: for (Node *ptr = _head; ptr != nullptr; ptr ptr->next) %3D 3 1 if (e ptr->element) { 4. Node *deleteMe; 6. deleteMe ptr->next; ptr->next->element; ptr->next->next; %3D 7 ptr->element ptr->next delete deleteMe; %3D 8. %3D 9. 10 return true; } } return false; 11 12 13 14 } The student tested it with this linked list: head nullptr Strangely he found that it worled perfectly except it crashed with a segmentation fault for only one value. What was the value of e that made it crash? What was the number of the line where it crashed?
A student implementing a linked list wrote this method: 1 bool LinkedList::Remove(int e) { 2 1: for (Node *ptr = _head; ptr != nullptr; ptr ptr->next) %3D 3 1 if (e ptr->element) { 4. Node *deleteMe; 6. deleteMe ptr->next; ptr->next->element; ptr->next->next; %3D 7 ptr->element ptr->next delete deleteMe; %3D 8. %3D 9. 10 return true; } } return false; 11 12 13 14 } The student tested it with this linked list: head nullptr Strangely he found that it worled perfectly except it crashed with a segmentation fault for only one value. What was the value of e that made it crash? What was the number of the line where it crashed?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Linked List Removal Function
A student implementing a linked list wrote this method:
```cpp
bool LinkedList::Remove(int e) {
for (Node *ptr = _head; ptr != nullptr; ptr = ptr->next) {
if (e == ptr->element) {
Node *deleteMe;
deleteMe = ptr->next;
ptr->element = ptr->next->element;
ptr->next = ptr->next->next;
delete deleteMe;
return true;
}
}
return false;
}
```
### Linked List Test Case
The student tested it with this linked list:
- **Linked List Diagram**:
- _head -> 1 -> 2 -> 3 -> nullptr
### Issue Discovered
Strangely, the student found that it worked perfectly except it crashed with a segmentation fault for only one value.
- **Question 1**: What was the value of `e` that made it crash?
- **Question 2**: What was the number of the line where it crashed?
### Analysis of the Crash
The issue likely occurs when trying to access or delete a node reference that doesn't exist, particularly when `ptr->next` or `ptr->next->next` is null, leading to undefined behavior or a segmentation fault.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
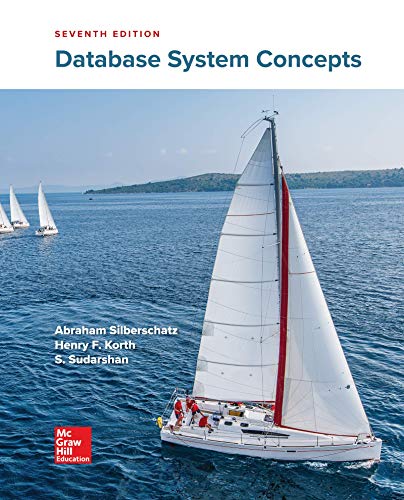
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
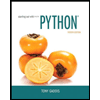
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
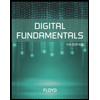
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
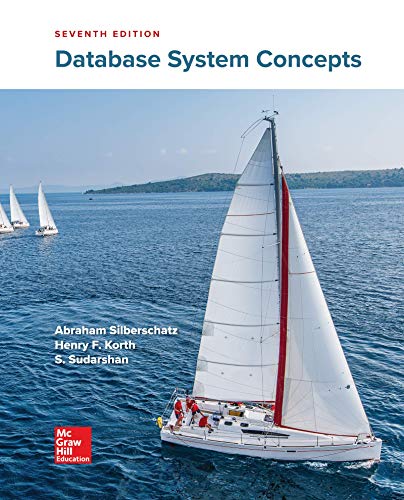
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
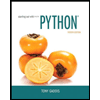
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
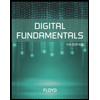
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
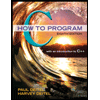
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
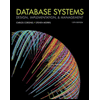
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
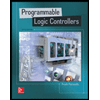
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education