zeroTriples.py: Write a program that reads a list of integers from the user, until they enter -12345 (the -12345 should not be considered part of the list). Then find all triples in the list that sum to zero. You can assume the list won’t contain any duplicates, and a triple should not use the same number more than once. For example: $ python3 zeroTriples.py 1 2 4 -12345 0 triples found $ python3 zeroTriples.py -3 1 4 2 -12345 1 triple found: 1, 2, -3 $ python zeroTriples.py -9 1 -3 2 4 5 -4 -1 -12345 4 triples found: -9, 4, 5 1, -3, 2 -3, 4, -1 5, -4, -1
zeroTriples.py: Write a program that reads a list of integers from the user, until they enter -12345 (the -12345 should not be considered part of the list). Then find all triples in the list that sum to zero. You can assume the list won’t contain any duplicates, and a triple should not use the same number more than once. For example: $ python3 zeroTriples.py 1 2 4 -12345 0 triples found $ python3 zeroTriples.py -3 1 4 2 -12345 1 triple found: 1, 2, -3 $ python zeroTriples.py -9 1 -3 2 4 5 -4 -1 -12345 4 triples found: -9, 4, 5 1, -3, 2 -3, 4, -1 5, -4, -1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
zeroTriples.py: Write a program that reads a list of integers from the user, until they enter -12345 (the -12345 should not be considered part of the list). Then find all triples in the list that sum to zero. You can assume the list won’t contain any duplicates, and a triple should not use the same number more than once.
For example:
$ python3 zeroTriples.py
1
2
4
-12345
0 triples found
$ python3 zeroTriples.py
-3
1
4
2
-12345
1 triple found:
1, 2, -3
$ python zeroTriples.py
-9
1
-3
2
4
5
-4
-1
-12345
4 triples found:
-9, 4, 5
1, -3, 2
-3, 4, -1
5, -4, -1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
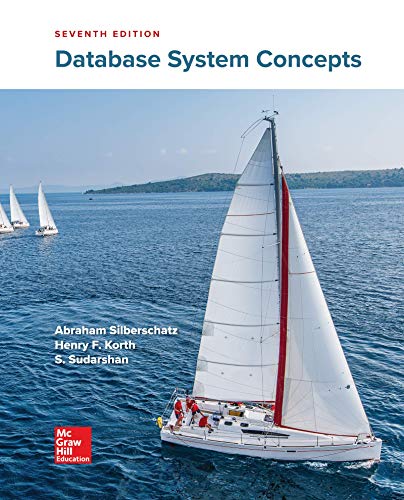
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
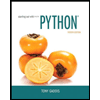
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
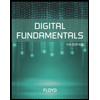
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
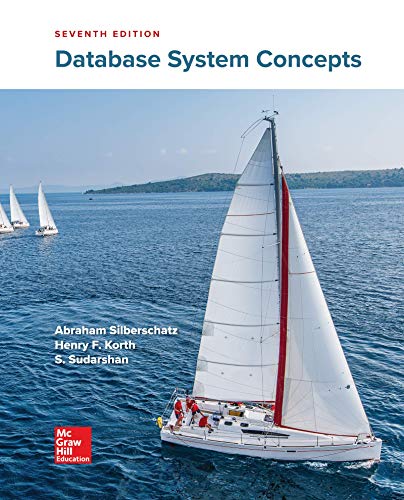
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
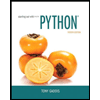
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
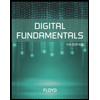
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
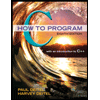
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
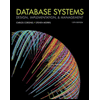
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
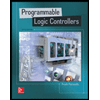
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education