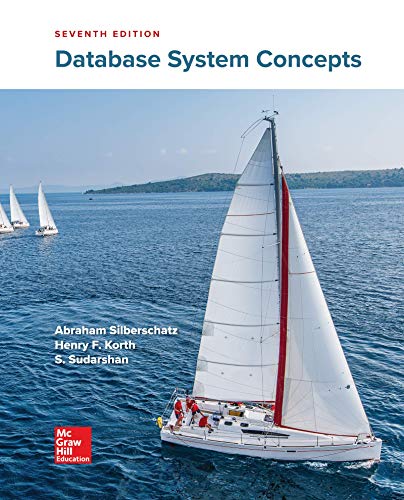
Concept explainers
import java.util.*;
public class RPSgame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Random random = new Random();
boolean playAgain = true;
while (playAgain) {
int computerChoice = random.nextInt(3); // 0 for rock, 1 for paper, 2 for scissors
int userChoice = getUserChoice(scanner);
System.out.println("Your choice: " + getChoiceName(userChoice) + ". Computer choice: " + getChoiceName(computerChoice) + ".");
int result = determineWinner(userChoice, computerChoice);
if (result == 0) {
System.out.println("It's a draw.");
} else if (result == 1) {
System.out.println("Computer wins.");
} else {
System.out.println("You win.");
}
System.out.print("Would you like to play more? (yes/no): ");
String playMore = scanner.next().toLowerCase();
playAgain = playMore.equals("yes") || playMore.equals("y");
}
System.out.println("Thanks for playing!");
}
private static int getUserChoice(Scanner scanner) {
int userChoice;
do {
System.out.print("Enter 1 for rock, 2 for paper, and 3 for scissors: ");
userChoice = scanner.nextInt();
} while (userChoice < 1 || userChoice > 3);
return userChoice - 1; // Convert to 0-based index
}
private static String getChoiceName(int choice) {
switch (choice) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
default:
return "invalid";
}
}
private static int determineWinner(int userChoice, int computerChoice) {
if (userChoice == computerChoice) {
return 0; // Draw
} else if ((userChoice + 1) % 3 == computerChoice) {
return -1; // Computer wins
} else {
return 1; // User wins
}
}
}
Test Case 1
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER
Test Case 2
2ENTER
Your choice: paper. Computer choice: paper.\n
It's a draw.\n
Would you like to play more?\n
nENTER

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Please answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…arrow_forwardPlease write a java code using the photo below. The second photo is the output of my program that I am writingarrow_forwardIn Java: Write a method that multiplies all elements in the array by 3 and returns it to the main method.arrow_forward
- Problem: Write a method that will determine whether a given value can be made given an array of coin values. For example, each value in the array represents a coin with that value. An array with [1, 2, 3] represents 3 coins with the values, 1, 2, and 3. Determine whether or not these values can be used to make a desired value. The coins in the array are infinite and can only be used as many times as needed. Return true if the value can be made and false otherwise. Dynamic Programming would be handy for this problem. Data: An array containing 0 or more coin values and an amount. Output: Return true or false. Sample Data ( 1, 2, 3, 12, 5 ), 3 ( 4, 15, 16, 17, 1 ), 21 ( 1 ), 5 ( 3 ), 7 Sample Output true true true falsearrow_forwardplease complete this method /** * Checks whether an item is inside the set. * @param item to be checked * @return true iff the set contains the item */public boolean contains(T item) {}arrow_forwardThe Delicious class – iterative methods The Delicious class must:• Define a method called setDetails which has the following header: public void setDetails(String name, int price, boolean available) This must find in the ArrayList the Chicken with the given name, change itspricePerKilo to the given price parameter and set whether it is in stock or not. A value of true for the available parameter means the chicken is in stock and a value of false means it is sold out (not in stock). Note that there will only be at most one Chicken object of the given name stored in the ArrayList.• Define a method called removeChicken that takes a single String parameter representing a chicken’s name. This method must remove from the ArrayList the Chicken object (if any) with the given name. The method must return true if a cheese with the given name was found and removed, and false otherwise.The Delicious class – challenge method In the Delicious class complete the findClosestAvailable method: This…arrow_forward
- In Java (Use the isPrime Method) PrimeNumberMethod.java, provides the isPrime(int number) method for testing whether the number is prime. Use this method to find the number of prime numbers less than 10000. Below are some clarifications for the Java program (a) The lab is to COUNT the prime numbers less than 10000. Do NOT list the prime numbers. (b) A pair prime is two prime numbers whose difference is 2. For e.g. 3,5; 11,13; 17, 19; etc. We can use IsPrime function to count how many pair primes are there in a range from 2 to 100 for example. Create a function pairPrime, that takes one argument. pairPrime should return the number of pair primes between 2 and the argument passed. Use the pairPrime function to count the pair primes between 2 and 1000. Make use of the IsPrime function to solve this task. (This feature is worth 8 points). Use the compile/run button to test tour program. Include the output displayed. It will be similar to: command>javac…arrow_forwardFor java. Refer to picture.arrow_forwardPYTHON: This exercise is a variation on "instrumenting" the recursive Fibonacci program to better understand its behavior. Write a supporting method that counts how many times the fib function is called to compute fib (n) where n is a user input. Hint: To solve this problem, you need an accumulator variable whose value "persists" between calls to fib. You can do this by making the count an instance variable of an object. Create a FibCounter class with the following methods: _init_(self) Creates a new FibCounter, setting its count instance variable to 0. getCount(self) Returns the value of count. fib(self, n) Recursive function to compute the nth Fibonacci number. It increments the count each time it is called. resetCount(self) Sets the count back to 0.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
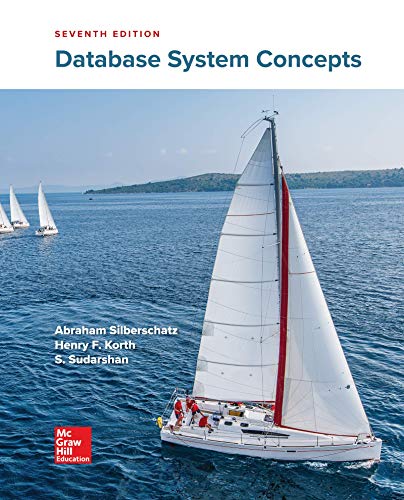
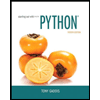
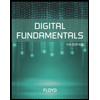
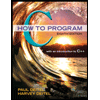
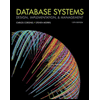
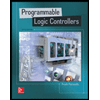