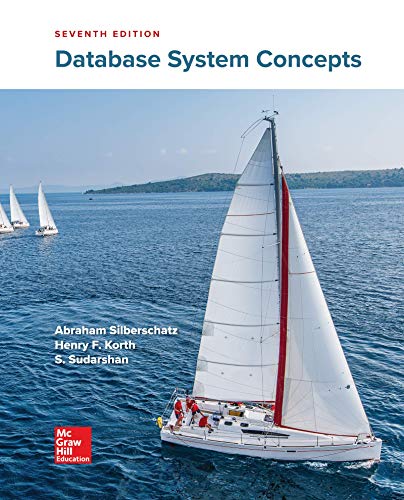
Java:
Please solve without using Hash set.
The class will contain the following static methods:
reverseS – A method that displays a string reversely on the console using the following signature:
public static void reverseS(String s)
printSub1 – print all substrings of a string (duplicated substrings are allowed, but loops are not allowed).
The method signature: public static void printSub1(String s)
printSub2 – print all substrings of a string (duplicated substrings are not allowed, but loops are allowed).
The method signature: public static void printSub2(String s) Note:
All methods should be RECURSIVE.
Any predefined classes that are based on Set are not allowed.
Allow the user to input the string!
In the main method, read a string from the user and output the reversed string and substrings to the screen:
Sample Run:
Please input a string: abcd
The reversed string: dcba
The substrings of the input string: abcd abc ab a b bc b c bcd bc b c cd c d
The substrings of the input string: a ab abc abcd b bc bcd c cd d

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Problem 2: Complete the seperateDuplicateChars () method in Seperate Duplicates.java as follows: The method takes a String str as a parameter and returns a new String. The returned String should be exactly like str, but any identical characters that appear in a consecutive way must be separated by hyphens "-". You may ONLY use the following methods from the String class: charAt(), substring() and length(). Other than uncommenting the code, do not modify the main method in Seperate Duplicates.java. Sample runs provided below. Argument String str "Hello" "Bookkeeper" "Yellowwood door" "Chicago Cubs" Return String "Hel-lo" "Bo-ok-ke-eper" "Yel-low-wo-od do-or" "Chicago Cubs"arrow_forward1. Write a program that takes three numbers (a,b,c) from the user as input and finds the median of the three. The program has a method called median that takes 3 numbers as the parameter and returns the median. Use if-then-else statements to find the median. You can assume the three numbers are not the same. Sample Example: Enter 3 numbers 10 13 The median is 10 public class Median{ public static void main(String[] args){ Scanner input = new int a = int b = int c =arrow_forwardNo written by hand solution Java Please Define stubs for the methods called by the below main(). Each stub should print "FIXME: Finish methodName()" followed by a newline, and should return -1. Example output: FIXME: Finish getUserNum() FIXME: Finish getUserNum() FIXME: Finish computeAvg() Avg: -1 import java.util.Scanner; public class MthdStubsStatistics { //solution here public static void main(String [] args) { int userNum1; int userNum2; int avgResult; userNum1 = getUserNum(); userNum2 = getUserNum(); avgResult = computeAvg(userNum1, userNum2); System.out.println("Avg: " + avgResult); } }arrow_forward
- Rules: Corner cases. By convention, the row and column indices are integers between 0 and n − 1, where (0, 0) is the upper-left site. Throw an IllegalArgumentException if any argument to open(), isOpen(), or isFull() is outside its prescribed range. Throw an IllegalArgumentException in the constructor if n ≤ 0. Unit testing. Your main() method must call each public constructor and method directly and help verify that they work as prescribed (e.g., by printing results to standard output). Performance requirements. The constructor must take Θ(n^2) time; all instance methods must take Θ(1)Θ(1) time plus Θ(1)Θ(1) calls to union() and find().arrow_forwardSimple try-catch Program This lab is a simple program that demonstrates how try-catch works. You will notice the output when you enter incorrect input (for example, enter a string or double instead of an integer). Type up the code, execute and submit the results ONLY. Do at least 2 valid inputs and 1 invalid. NOTE: The program stops executing after it encounters an error! CODE: import java.util.Scanner; public class TryCatchExampleSimple { public static void main(String[] args) { Scanner input = new Scanner(System.in); int num = 0; System.out.println("Even number tester.\n"); System.out.println("Enter your name: "); String name input.nextLine(); } } while (true) { try { System.out.println("Enter an integer : "); num= input.nextInt (); } int mod = num%2; if (mod == 0) " System.out.println(name + else " System.out.println(name + num = 0; } catch (Exception e) { break; > System.out.println("ERROR: The number you entered is illegal!"); System.out.println("Exception error: "+e.toString());…arrow_forwardPlease help me this I need answer typing clear urjent no chatgpt used i will give 5 upvotesarrow_forward
- Complete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardUsing an appropriate package and test class name, write the following tests for GamerProfile's constructor: testNameShouldNotBeNull testNameShouldNotBeEmpty testNameShouldNotBeBlank testShouldCreateValidGamerProfile Hint: use assertTrue or assertFalse for getters involving boolean values Hint: get the game list from the gamer and call the list's isEmpty along with an assertTrue or assertFalse, as appropriate.GamerProfile:public class GamerProfile { private String userName; private boolean pvpEnabled; private boolean online; private ArrayList<GameInfo> gameLibrary; public GamerProfile(String userName) { this.userName = userName; this.pvpEnabled = false; this.online = false; gameLibrary = new ArrayList<GameInfo>(); } // Getter for the getUserName variable public String getUserName() { return userName; } // Getter for the PvpEnabled…arrow_forward2. A pentagonal number is defined as n(3n-1)/2 for n = 1, 2, ..., etc.. Therefore, the first few numbers are 1, 5, 12, 22, ... . Write a method with the following header that returns a pentagonal number: %3D public static int getPentagonalNumber(int n) For example, getPentagonalNumber(1) returns 1 and getPentagonalNumber(2) returns 5. Write a test program that uses this method to display the first 100 pentagonal numbers with 10 numbers on each line. Numbers are separated by exactly one space.arrow_forward
- Integer userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forwardRepeating String Write a method that takes a String and an int and return a String that is the original String repeated n times. For example, repeatString("Famous", 3) should return "FamousFamousFamous" The method signature should be public String repeatString(String str, int n) public String repeatString(String str, int n) { //declare a string variable //set up statements for repeating n number of times //create the appropriate return statement }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
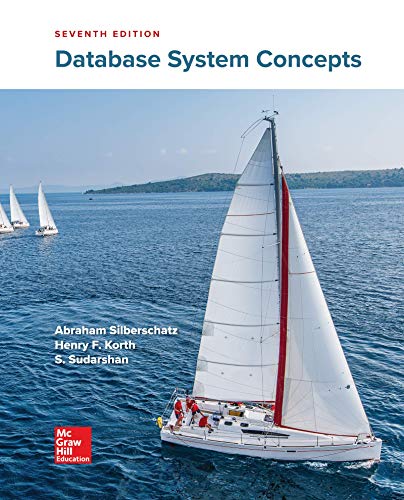
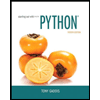
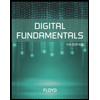
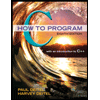
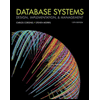
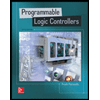