Write a loop that subtracts 1 from each element in lowerScores. If the element was already 0 or negative, assign 0 to the element. Ex: lowerScores = {5, 0, 2, -3} becomes {4, 0, 1, 0}. #include using namespace std; int main() { const int SCORES_SIZE = 4; int lowerScores[SCORES_SIZE]; int i; for (i = 0; i < SCORES_SIZE; ++i) { cin >> lowerScores[i]; } /* Your solution goes here */ for (i = 0; i < SCORES_SIZE; ++i) { cout << lowerScores[i] << " "; } cout << endl; return 0; }
Write a loop that subtracts 1 from each element in lowerScores. If the element was already 0 or negative, assign 0 to the element. Ex: lowerScores = {5, 0, 2, -3} becomes {4, 0, 1, 0}.
#include <iostream>
using namespace std;
int main() {
const int SCORES_SIZE = 4;
int lowerScores[SCORES_SIZE];
int i;
for (i = 0; i < SCORES_SIZE; ++i) {
cin >> lowerScores[i];
}
/* Your solution goes here */
for (i = 0; i < SCORES_SIZE; ++i) {
cout << lowerScores[i] << " ";
}
cout << endl;
return 0;
}

Algorithm:
for (initialExpression; testExpression; updateExpression){
// body of the loop
}
Step 1: The initialExpression initializes and declares variables and executes only once.
Step 2: The condition is evaluated. If the condition is true, the body of the for loop executed.
Step 3: The update expression updates the value of initialExpression.
Step 4: The condition is evaluated again. The process continues until the condition is false.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

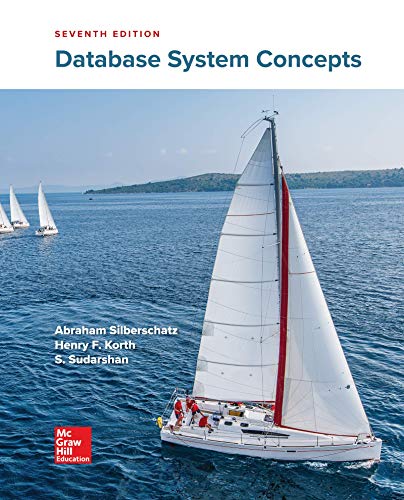
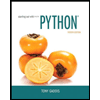
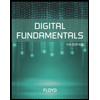
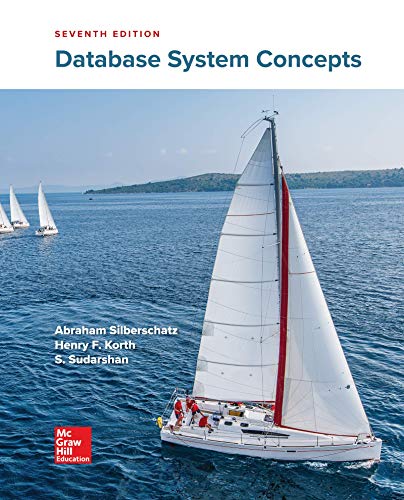
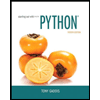
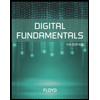
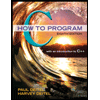
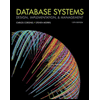
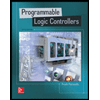