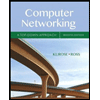
Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons.
We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list.
Your implementation should be O(n) for add, and O(1) for the remaining methods.
Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons.
We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list.
Your implementation should be O(n) for add, and O(1) for the remaining methods.
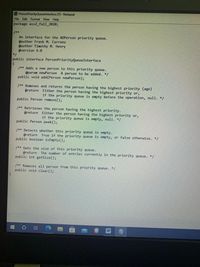
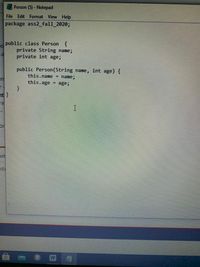

Step by stepSolved in 2 steps

- Write a generic class Students.java which has a constructor that takes three parameters – id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString() method in this class will display these details for any student. A generic method score() will be part of this class and it will be implemented by inherited classes. Write accessors and mutators for all data points. Write two classes RemoteStudents.java and InPersonStudents.java that inherits from Student class. Show the use of constructor from parent class (mind you, RemoteStudents have one additional parameter – discussion). Implement the abstract method score() of the parent class to calculate the weighted score for both types of students. Write a driver class JavaProgramming.java which has the main method. Create one remote student object and one in-person student object. The output should show prompts to enter individual scores – midterm, finals, ...... etc. and the program will…arrow_forwardDefine a constructor on the simpy class that takes a parameter of type list[float].Its purpose is to initialize the values attribute of the newly constructed simpy object to the argument passed in. Once your constructor is properly implemented, you expect to see [1.0, 1.0, 1.0, 1.0, 1.0] printed when evaluating the cell below. ones = Simpy([1., 1., 1., 1., 1.]) print(ones.values) 0.1s Pythonarrow_forwardMovie Recommendations via Item-Item Collaborative Filtering. You are providedwith real-data (Movie-Lens dataset) of user ratings for different movies. There is a readmefile that describes the data format. In this project, you will implement the item-item collab-orative filtering algorithm that we discussed in the class. The high-level steps are as follows: a) Construct the profile of each item (i.e., movie). At the minimum, you should use theratings given by each user for a given item (i.e., movie). Optionally, you can use other in-formation (e.g., genre information for each movie and tag information given by user for eachmovie) creatively. If you use this additional information, you should explain your method-ology in the submitted report. b) Compute similarity score for all item-item (i.e., movie-movie) pairs. You will employ thecentered cosine similarity metric that we discussed in class. c) Compute the neighborhood set Nfor each item (i.e. movie). You will select the moviesthat…arrow_forward
- We have a parking office class for an object-oriented parking management system using java Add(implement) a method to the Parking Office class to return the collection of permit ids for a specific customer (getPermitIds(Customer)) using java I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getPermitIds(Customer) method as mentioned above Explain your code in a few words Important- We have a method to return a collection of permit ids (getPermitIds), what we need is a method to return the collection of permit ids for a specific customer (getPermitIds(Customer))arrow_forwardWrite a class that tests and demonstrates that your Candidate and Electionclasses implement the specification given above. Note that this does not need to be a Junit test class.Your test class must illustrate all of the features that you have implemented. This will best be done by having at least one separate test method for each feature. For instance:• A method that creates a Candidate object and prints out the result of calling each of its accessor (get) methods; • A method that adds a vote to an existing Candidate object.• A method that creates an Election object, creates a few Candidateobjects and adds them to it, and then calls the printAll method;• A method that finds a matching Candidate;• A method that does not find a matching Candidate;• etc.Note that the list above is not a complete list of the test class’s methods and you will need to define more than those for this part. It will be worth writing this test class alongside writing the other classes. For instance, develop the…arrow_forwardWhich of the following cases is most like the strategy pattern? A. If you have an interface called Duck, and have many ducks that implement the duck interface. All of these ducks must have the behaviour “quack” but for some ducks, like a Mallard, this must result in a quack, where for a rubber duck it must result in a squeak. We decide to delegate the quack method to another interface, which can be implemented either in a concrete class that returns a quack or a concrete class that returns a squeak. B. If we have an interface called Duck. We need to give an object to a user that can quack like a duck. All we have is Turkeys. We create a special class that implements the duck interface, and to implement the quack method we simply call the turkey’s gobble method. C. None of these are like the strategy pattern. D. We have a large collection of Ducks. We want to look at them one by one and hear them quack. We write a piece of code that iterates through them all, and as we encounter…arrow_forward
- We have a parking office class for an object-oriented parking management system using java Add (implement )a function to the Parking Office class to return a collection of customer ids (getCustomerIds) using java I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getcustomerID function as mentioned above Explain the code you wrote with a few wordsarrow_forwardFor this assignment, your implementation plan must include at least one class with public and private sections. For assistance with classes, see sections 6.2, "Using a Class," and 6.3, "Defining a Class," in zyBooks. For this assignment, we recommend using Maps (section 11.4 in zyBooks) for implementation. Maps do require precise coding syntax. However, you can choose a different implementation option. Apply industry standard best practices such as in-line comments and appropriate naming conventions to enhance readability and maintainability. Remember that you must demonstrate industry standard best practices in all your code to ensure clarity, consistency, and efficiency. This includes the following: Inserting in-line comments to denote your changes and briefly describe the functionality of the code Using appropriate variable, parameter, and other naming conventions throughout your code Optional Challenge: User Input ValidationValidating user input is a coding best…arrow_forwardSally and Harry are working on an implementation of Coin. Sally declares the sole parameter to her compareTo method as type Object. Harry knows the compareTo method will always be called on objects of type Coin and declares the parameter to be of that type. Which method is suitable for storing Coin objects in a OrderedVector?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
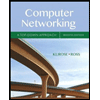
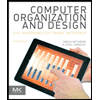
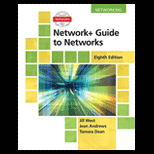
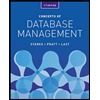
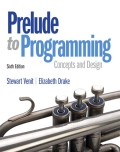
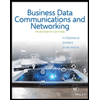