JAVA: Fill in the ParsedRequest method in the CustomParser.java file. The point of it is to parse the data that is found in the BasicRequestTest.java file.
Computer Science
JAVA: Fill in the ParsedRequest method in the CustomParser.java file. The point of it is to parse the data that is found in the BasicRequestTest.java file.
CustomParser.java:
package request;
public class CustomParser {
public static ParsedRequest parse(String request){
//fill in here
}
}
ParsedRequest.java:
package request;
import java.util.HashMap;
import java.util.Map;
public class ParsedRequest {
private String path;
private Map<String,String> queryMap = new HashMap<>();
private String method;
private String body;
public String getQueryParam(String key){
return queryMap.get(key);
}
public void setQueryParam(String key, String value){
this.queryMap.put(key, value);
}
public void setPath(String path) {
this.path = path;
}
public void setMethod(String method) {
this.method = method;
}
public String getPath(){
return path;
}
public String getMethod(){
return method;
}
public String getBody(){
return body;
}
public void setBody(String body) {
this.body = body;
}
}
BasicRequestTest.java:
package request;
import org.testng.Assert;
import org.testng.annotations.Test;
public class BasicRequestTest {
@Test
public void basicTest(){
String test1 = "GET /hello HTTP/1.1\n"
+ "User-Agent: Mozilla/4.0 (compatible; MSIE5.01; Windows NT)\n"
+ "Accept-Language: en-us\n"
+ "Accept-Encoding: gzip, deflate\n"
+ "Connection: Keep-Alive";
ParsedRequest request = CustomParser.parse(test1);
Assert.assertEquals(request.getPath(), "/hello");
Assert.assertEquals(request.getMethod(), "GET");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

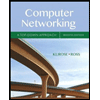
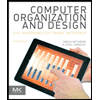
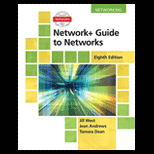
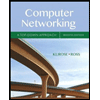
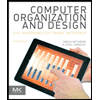
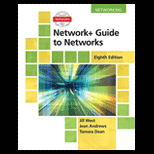
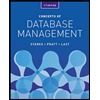
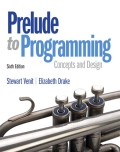
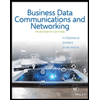