What numbers can we get with the call random.randrange (0,20,7) We assume that the module was randomly previously imported.
Q: Can you please write the follwing in Java. You will implement this program in a specific way in…
A: Start the program.Declare an array of strings called "fruits" and initialize it with four fruit…
Q: Edit only the class definition. DO NOT CHANGE the code given under 'main' please. Steps:…
A: from math import hypot, pi, cos, sinfrom random import uniformimport turtle as t class Vector2D:…
Q: op = rand.nextInt(2) == 0 ? '+' : '-'; I want to add two more operators to this, but can't get it to…
A: Given, op = rand.nextInt(2)==0 ? "+" : "-"; NOTE: The function rand.nextInt(n) generates random…
Q: the method takes two parameters, the whole array and an element to search. The method returns the…
A: Program.cs : //This is system namespaceusing System;//application namespacenamespace…
Q: How can you prevent accidental method overriding in your code? Are there any best practices?
A: Preventing accidental method overriding in your code is crucial to maintain the integrity and…
Q: I am having trouble with this: Implement the convertString method based on its specification. Here…
A: As per the given question, we need to implement convertString() method. Algorithm for convertString…
Q: Create a Java class Recursive Methods.java and create the following methods inside: ALL THE METHODS…
A: Solution: We have to create a class RecursiveMethods.java having methods oddEvenMatchRec(int arr[],…
Q: 1 def rest_time(miles_driven, brake_count): count = 0 3 count = round (miles_driven/brake_count) 4…
A: code : - from math import floor # method to check your time def rest_time(miles_driven,…
Q: Create a class called IntegerOps, with the following requirements: • Read two arguments from…
A: Here Math.hypot(a,b); will return sqrt(a2 + b2) Math.min(a,b); will return min of a or b…
Q: Create a KeyIndex class with the following properties : Fields: An array of integers.…
A: The
Q: READY CAREFULLY Java add comment to code, please write your test-cases on how you would test your…
A: The answer is given below:-
Q: Develop a program which allows the user to enter numbers into an array. Input will be as follows:The…
A: Answer import java.util.Scanner; public class ArraysSort { /** * @param args */…
Q: Over the first few Long Weekly Homework assignments for this quarter, we have been building a…
A:
Q: The Hole class will: extend Cell class implement HoleInterface • override the receiveRat() - Cell…
A: In this Java programming lesson, we will implement the Hole class, which extends the Cell class and…
Q: Explain encapsulation and packaging in Java. Part II Create a package for int array operations.…
A: Encaptulation is a feature of object oriented feature , its main purpose is to wrap internal…
Q: Create and implement a different solution to the use of semaphores using pseudocode or some…
A: The algorithm is given below for 5 philosopher .
Q: you need to add a method for finding the list of passed modules and one method for total mark…
A: The program is implemented in Java using structured approach. I have declared two function which you…
Q: manner like if we have 1 pair then only 1 possibility. if we have 3 pairs : then possible.
A: This is a Java program to find the number of balanced bracket combinations for a given number of…
Q: Can you please show the getter and setter?
A: getters and setters are shown below as well as explained how to use them
Q: There are 4 types of passengers in the system: Standard: This is the most common type of passenger…
A: Polymorphism in Java is the ability of an object or method to take on various forms based on the…
Q: it is NOT allowed to use any library orpackage function to directly generate random numbers that…
A: To fulfill the requirements of this project, we need to enforce a discrete-event simulator for a…
Q: ); D: ¡ D: ; ); Test Cases main.c:2015: error: else without a previous "14" 201 else if("nXS-8");…
A: #include<stdio.h>void main(){int n;printf("Enter n: ");scanf("%d",&n);for(int…
Q: Create a new project in IntelliJ called Recursion. Add a new Java class called Recursion with a…
A: Recursion is a process of calling the same function itself
Q: The task for this part is to perform testing of the HeapMax class. You need to create a class named…
A: Solution : As the requirements specified in the given problem statement ,here is the java code.…
Q: Write factorial1 function in python 3.8 follow the directions provided below, don't need anything…
A: Given : Function: factorial1 The function implements an iterative factorial. It takes an…
Q: Write a program GuessMyNumber game. When it's finished, it will work like this: I'm thinking of a…
A: java code for the given question :- import java.util.*;import java.lang.*; class GuessMyNumber {…
Q: fizzbuzzy Dat
A: #include <stdio.h> void fizzbuzzy(int max, int step, int divisorOne, int divisorTwo) { for…
Q: Practice these steps again and then solve the set of loop problems. These problems are more…
A: Here, we have used for loop and if-else statements to implement the required functions. For loop is…
Q: For this problem you will accept a single BigInteger value. This number can potentially get VERY…
A: The complete JAVA code is given below with output screenshot
Q: Rewrite the code so break isn't needed for the riddles
A: A break loop is a condition that allows a program to terminate and exit a loop. break command comes…
Q: 1.A) The median of a set of integers is the middle-most integer in size. That is, half the integers…
A: EXPLANATION: - To find the Median, the array needs to be sorted first. If the array is having an…
Q: Provide full C++ Code This assignment is a review of loops. Do not use anything more advanced than a…
A: The objective of the question is to create a simple command line game of blackjack using C++. The…
Q: Lab Goal : This lab was designed to teach you more about recursion. Lab Description : Take a…
A: Answer
Q: omo loriab. Jva
A: Here, In the Fibonacci series, the next number is the sum of the previous two numbers. Example: 0,…
Q: What will you add to 9 to become 6?
A: Answer -3
What numbers can we get with the call random.randrange (0,20,7)
We assume that the module was randomly previously imported.

Answer to the random range() is given in step 2.
Step by step
Solved in 2 steps with 1 images

- (Game: ATM machine) Use the Account class created in our previous Lab Exercise to simulate an ATM machine. Create ten accounts in an array with id 0, 1, . . ., 9, and initial balance $100. The system prompts the user to enter an id. If the id is entered incorrectly, ask the user to enter a correct id. Once an id is accepted, the main menu is displayed as shown in the sample run. You can enter a choice 1 for viewing the current balance, 2 for withdrawing money, 3 for depositing money, and 4 for exiting the main menu. Once you exit, the system will prompt for an id again. Thus, once the system starts, it will not stop.Follow instructions correctly. After completing the methods, use the main method to test them.Course: Algorithm Project: We will use the defintion of of n-Queens Problem from the chapter Backtracking. In this project you need to describe Problem and Algorithm and Indicate input and output clearly. Analyze and prove the time complexity of your algorithm. Implement the algorithm using backtracking(including writing testing case).illustrate key functions with comments indicating: What it does, what each parameter is used for, how it handles errors etc. Indicate the testing scenarios and testing the results in a clear way. Make sure source is commented appropriately and structured well.
- (YOU ARE NOT ALLOWED TO USE ARRAYLIST IN THIS PROJECT)Write a Java program to simulate a blackjack game of cards. The computer will play the role of the dealer. The program will randomly generate the cards dealt to the player and dealer during the game. Cards in this game will be represented by numbers 1 to 13 with Ace being represented by a 1. Remember, that face cards (i.e. Jack, Queen, and King) are worth 10 points to a hand while an Ace can be worth 1 or 11 points depending on the user’s choice. The numbered cards are worth their number value to the hand.Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!Please use Python and don't make the code overly complicated and lengthy. Thanks. Create a KeyIndex class with the following properties : Fields: int [ ] k; Description An array of integers. Note: You can maintain another instance variable if needed (but you can’t use more than one). Constructor: KeyIndex(int [ ]a) Description: This constructor takes an array of integers a and populates array k with the element in a as indices into k. Note: make sure the build-up of your array k supports negative and non-distinct integers. Methods: search (int val) Description: This method searches for the value val within the array and returns true if found or false otherwise. sort () Description: This method will return the sorted form of the array that had been passed into the constructor. NOTE: Create a tester class or write tester statements to check whether the methods in your KeyIndex class work properly
- No plagarism! Correct and detailed answer ( code with comments and output screenshot if necessary)I want more simple solution please and I use replit to implement this code and I want you define class not struct pleaseFinish the TestPlane class that contains a main method that instantiates at least two Planes. Add instructions to instantiate your favorite plane and invoke each of the methods with a variety of parameter values to test each option within each method. To be able to test the functionality of each phase, you will add instructions to the main method in each phase.
- Follow instructions pleaseI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…READY CAREFULLY Java add COMMENT TO CODE, please TEST-CASE on how you would test your solution assumptions and hence your code. Example of cases to be tested for are like : What if the array input which is expected does not exist - that is , input is a null. How should your code handle such a situation ? Maybe output some message like "Null input case, so no output"? What if the length of the array is one ?....so on and so forth. In this assignment you are required programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free! Below is how the arrays are represented ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21] Here length of ARRAY1 is m. ARRAY2[] = [6, 6, 9, 11, 21, 21, 21] Here length of ARRAY2 is n. Array to be returned would be: ARRAY[] = [6, 9, 11, 21] ANSWER THE QUESTION BELOW. Implement the function…
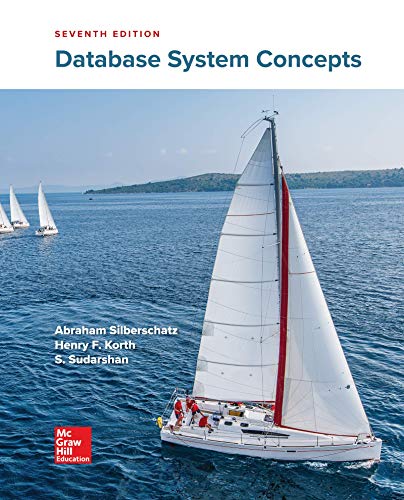
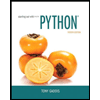
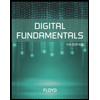
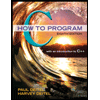
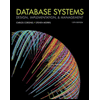
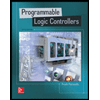
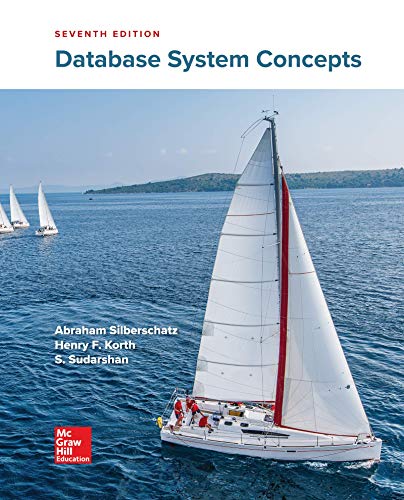
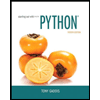
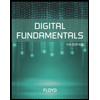
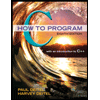
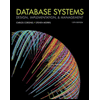
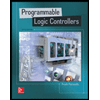