Please explain the questions related to the code below: //1. Why is 20 printed 3 times and why does it start with 1, but end with 2? //2. What is the base case for this recursive method and why? //3. How would you implement this using a loop? //4. What is each if statement checking? //5. Would a solution to this using loops require more or less code and would it be more or less effecient? //6. Why is recursion a good choice to solve this problem? //7. How can recursion become infinite? //8. When is recursion appropriate? /* Load the code below into a cloud compiler and run each method one at a time by removing the comments for the method. Walk through the code as a group and explore how the code works. Submit the answers to the numbered questions for credit. */ public class DemoRecur { //Simple statement designed to show control flow in recursion public static void printDemo(int x, int max) { System.out.print(x + "\n"); if (x != max) printDemo(++x,max); System.out.print(x + "\n"); } //1. Why is 20 printed 3 times and why does it start with 1, but end with 2? //Factorial is the multiplication of all numbers up to a specified number usually //written !num //Example: !3 -> 1*2*3 //0! = 1 and 1! = 1 public static int factorial(int num) { if (num <= 1) return 1; else return num * factorial(num-1); } //2. What is the base case for this recursive method and why? //3. How would you implement this using a loop? //Fibonacci Series //Except for 0 and 1 every number is the sum of the fibonacci of the previous 2 //fib(0) = 0; fib(1) = 1; fib(num) = fib(n-1) + fib(n-2) //0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, //What are your base cases? public static int fib(int num) { if (num == 0 || num == 1)//base case return num; else return fib(num-1) + fib(num-2);//recursive step } //Check to see if a provided string is a palindrome public static boolean palindromeCheck(String data) { if(data.length()==0 || data.length() ==1) return true; if(data.charAt(0) == data.charAt(data.length()-1)) return palindromeCheck(data.substring(1, data.length()-1)); return false; } //4. What is each if statement checking? //5. Would a solution to this using loops require more or less code and would it be more or less effecient? //Tower of Hanoi (Don't spend too much time on this one. See if you can grasp it conceptually.) //Watch Tower of Hanoi youtube video //https://www.youtube.com/watch?v=YstLjLCGmgg public static void solveTowers(int disks, int sourcePeg, int destPeg, int tempPeg) { if (disks ==1) { System.out.println(sourcePeg + "---->" + destPeg); return; } solveTowers(disks - 1, sourcePeg, tempPeg, destPeg); System.out.println(sourcePeg + "---->" + destPeg); solveTowers(disks -1, tempPeg, destPeg, sourcePeg); } //6. Why is recursion a good choice to solve this problem? public static void main(String [] args) { //printDemo(1,20); //System.out.println(factorial(7)); //System.out.println(fib(9)); /* System.out.println(palindromeCheck("racecar")); System.out.println(palindromeCheck("tacocat")); System.out.println(palindromeCheck("bobbob")); System.out.println(palindromeCheck("rock")); */ //solveTowers(4,1,3,2); } //7. How can recursion become infinite? //8. When is recursion appropriate? }
Please explain the questions related to the code below:
//1. Why is 20 printed 3 times and why does it start with 1, but end with 2?
//2. What is the base case for this recursive method and why?
//3. How would you implement this using a loop?
//4. What is each if statement checking?
//5. Would a solution to this using loops require more or less code and would it be more or less effecient?
//6. Why is recursion a good choice to solve this problem?
//7. How can recursion become infinite?
//8. When is recursion appropriate?
/*
Load the code below into a cloud compiler and run each method one at a time by removing the comments for the method.
Walk through the code as a group and explore how the code works.
Submit the answers to the numbered questions for credit.
*/
public class DemoRecur
{
//Simple statement designed to show control flow in recursion
public static void printDemo(int x, int max)
{
System.out.print(x + "\n");
if (x != max)
printDemo(++x,max);
System.out.print(x + "\n");
}
//1. Why is 20 printed 3 times and why does it start with 1, but end with 2?
//Factorial is the multiplication of all numbers up to a specified number usually
//written !num
//Example: !3 -> 1*2*3
//0! = 1 and 1! = 1
public static int factorial(int num)
{
if (num <= 1)
return 1;
else
return num * factorial(num-1);
}
//2. What is the base case for this recursive method and why?
//3. How would you implement this using a loop?
//Fibonacci Series
//Except for 0 and 1 every number is the sum of the fibonacci of the previous 2
//fib(0) = 0; fib(1) = 1; fib(num) = fib(n-1) + fib(n-2)
//0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144,
//What are your base cases?
public static int fib(int num)
{
if (num == 0 || num == 1)//base case
return num;
else
return fib(num-1) + fib(num-2);//recursive step
}
//Check to see if a provided string is a palindrome
public static boolean palindromeCheck(String data)
{
if(data.length()==0 || data.length() ==1)
return true;
if(data.charAt(0) == data.charAt(data.length()-1))
return palindromeCheck(data.substring(1, data.length()-1));
return false;
}
//4. What is each if statement checking?
//5. Would a solution to this using loops require more or less code and would it be more or less effecient?
//Tower of Hanoi (Don't spend too much time on this one. See if you can grasp it conceptually.)
//Watch Tower of Hanoi youtube video
//https://www.youtube.com/watch?v=YstLjLCGmgg
public static void solveTowers(int disks, int sourcePeg, int destPeg, int tempPeg)
{
if (disks ==1)
{
System.out.println(sourcePeg + "---->" + destPeg);
return;
}
solveTowers(disks - 1, sourcePeg, tempPeg, destPeg);
System.out.println(sourcePeg + "---->" + destPeg);
solveTowers(disks -1, tempPeg, destPeg, sourcePeg);
}
//6. Why is recursion a good choice to solve this problem?
public static void main(String [] args)
{
//printDemo(1,20);
//System.out.println(factorial(7));
//System.out.println(fib(9));
/*
System.out.println(palindromeCheck("racecar"));
System.out.println(palindromeCheck("tacocat"));
System.out.println(palindromeCheck("bobbob"));
System.out.println(palindromeCheck("rock"));
*/
//solveTowers(4,1,3,2);
}
//7. How can recursion become infinite?
//8. When is recursion appropriate?
}

Step by step
Solved in 2 steps

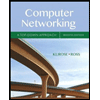
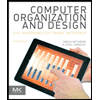
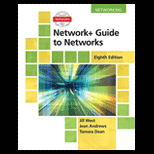
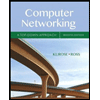
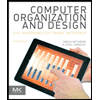
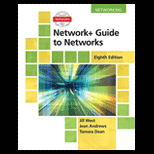
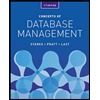
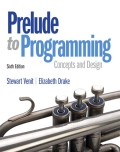
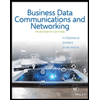