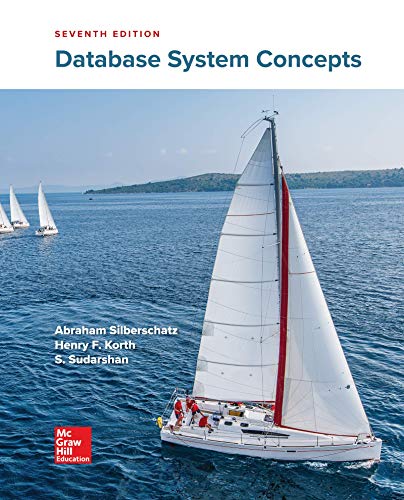
Concept explainers
READY CAREFULLY
Java add COMMENT TO CODE, please TEST-CASE on how you would
test your solution assumptions and hence your code.
Example of cases to be tested for are like : What if the array input which
is expected does not exist - that is , input is a null. How should your code
handle such a situation ? Maybe output some message like "Null input
case, so no output"? What if the length of the array is one ?....so on and
so forth.
In this assignment you are required programs which takes as input two sorted arrays and returns a new array containing the elements found in both the sorted arrays. It is alright if the input arrays have duplicates, but the returned array should be duplicate free!
Below is how the arrays are represented
ARRAY1[] = [1, 5, 6, 6, 9, 9, 9, 11, 11, 21]
Here length of ARRAY1 is m.
ARRAY2[] = [6, 6, 9, 11, 21, 21, 21]
Here length of ARRAY2 is n.
Array to be returned would be:
ARRAY[] = [6, 9, 11, 21]
ANSWER THE QUESTION BELOW.
- Implement the function in such a way that your solution solves the problem with O(nlog(m)) time complexity. This brute-force method suggested has a name called "loop-join" where you basically just traverse through the elements of one array comparing it to the elements of the other array.
2. In the form of sentences, as a comment in your code (at the bottom of your Solution2), you are required to suggest how can Solution2 be improved by leveraging the fact that both the arrays are already sorted. Suggest a solution so that your suggested solution can run linearly with O(m + n) time complexity. 5 sentences or less.

Find the duplicates in two sorted arrays :
program in java:
public class arrayduplicates {
public static void main(String[] args) {
int[] arr1 = {1,5,6,6,9,9,9,11,11,21};
int[] arr2 = {6, 6, 9, 11, 21, 21, 21};
Integer[] duplicates = duplicates(arr1, arr2);
for(Integer d: duplicates) {
System.out.println(d.intValue());
}
}
public static Integer[] duplicates(int[] arr1, int[] arr2) {
List<Integer> duplicates = new ArrayList<Integer>();
for(int count = 0; count < sorted1.length; count ++) {
for(int counter = 0; counter < arr2.length; counter ++) {
if(sorted1[count] == arr22[counter]) {
duplicates.add(arr1[count]);
} else if(arr1[count] < arr2[counter]) {
break;
}
}
}
return duplicates.toArray(new Integer[duplicates.size()]);
}
}
the above program time complexity is O(n log m)
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- I need to have a main method in a Tester class that will: Fill an array of type Vehicle[] of size NUM_VEHICLES with Vehicle objects. You need to generate a random number between 0 and 2. If the number is 0, add a Vehicle to the array. 1, add a Car, 2, add a Boat. The Vehicle/Car/Boat that you add must be initialized with a random efficiency between 0 and 100.0. Do this until you have added NUM_VEHICLES objects to the array How do I write it so that the array generates random numbers 0 through 2 (inclusive) and how would I then correlate those numbers to a different class object?arrow_forwardWrite the Java method averageOfDoubles that is passed an array of double values, and returns the average of the array values.You can assume the array length is a positive integer.arrow_forwardPlease use Java for the solutionarrow_forward
- Write a program in java in which we had an array and you have to find Whether this array is monotonic or notarrow_forwardCan you help me fix my code in Java, please? I am stuck to keep going. Thank youarrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
- Without using any pre-defined method. Don't copy from internet Two array is given and you check whether these array is equal or not in java. Without using any pre-defined methodarrow_forwardWrite a Java method (do not write a class, only the method) with the following signature: Return type: void Parameters: 2D integer array Name: printDiagonal Implement the method code that will print out the values of the 2D array along the Diagonal from left to right. For example, if the array had the following values: 56 78 98 21 33 55 98 44 11 The method should print to the console: 56,33,11 The above 2D array example is only given for you to understand the problem Do not hard code the values in your solution or assume the array is the same as the given data. Your method should work for all 2D integer arrays.arrow_forwardUse java.arrow_forward
- Please complete the task by yourself only in JAVA with explanation. Don't copy. Thank you. Using quicksort to sort an array of car objects by various criteria. Define a class Car as follows: class Car { public String make; public String model; public int mpg; // Miles per gallon } b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg.arrow_forwardAre there obvious improvements that could be made here with respect the software design for Squares and Circles? What programming constructs were you familiar with, and which did you need to look up? Assume we used a separate array for Squares and for Circles rather than one unifying Object array. How would this complicate the task of adding a new Shape (say, a Triangle) to our ObjectList class?arrow_forwardDesign and implement a class called RandomArray, which has an integer array. The constructor receives the size of the array to be allocated, then populates the array with random numbers from the range 0 through the size of the array. Methods are required that return the minimum value, maximum value, average value, and a String representation of the array values. Document your design with a UML Class diagram. Create a separate driver class that prompts the user for the sample size, then instantiates a RandomArray object of that size and outputs its contents and the minimum, maximum, and average values.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
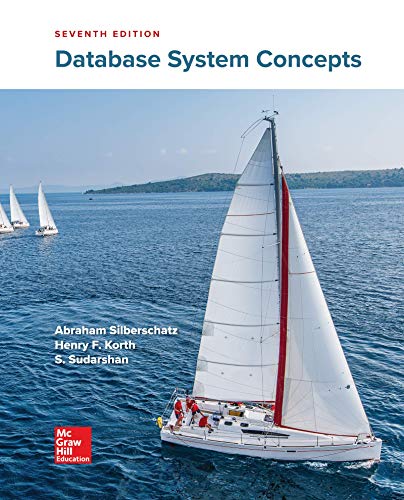
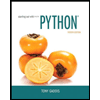
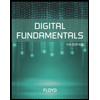
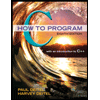
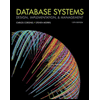
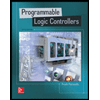