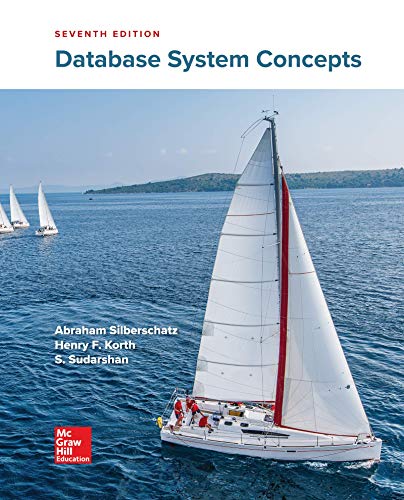
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
What is output?
#include <iostream>
#include <set>
#include <string>
using namespace std;
int main () {
set<int>number;
int i;
int myVar;
for (i = 0; i <=3; i++) {
number.insert(i*i);
}
myVar = number.count(9);
cout << myVar << endl;
return 0;
}
3
0
9
1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- #include #include using namespace std; //function prototypesint countLetters(char*); int countDigits(char*); int countWhiteSpace(char*); int main() { int numLetters, numDigits, numWhiteSpace; char inputString[51]; cout <<"Enter a string of no more than 50 characters: " << endl << endl; cin.getline(inputString,51); numLetters = countLetters(inputString); numDigits = countDigits(inputString); numWhiteSpace = countWhiteSpace(inputString); cout << "The number of letters in the entered string is " << numLetters << endl; cout << "The number of digits in the entered string is " << numDigits << endl; cout << "The number of white spaces in the entered string is " << numWhiteSpace << endl; return 0; } int countLetters(char *strPtr) { int occurs = 0; while(*strPtr != '\0') // loop is executed as long as// the pointer strPtr does not point // to the null character which// marks the end of the string // isalpha determines…arrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward#include <iostream>#include <list>#include <string>#include <cstdlib>using namespace std;int main(){int myints[] = {};list<int> l, l1 (myints, myints + sizeof(myints) / sizeof(int));list<int>::iterator it;int choice, item;while (1){cout<<"\n---------------------"<<endl;cout<<"***List Implementation***"<<endl;cout<<"\n---------------------"<<endl;cout<<"1.Insert Element at the Front"<<endl;cout<<"2.Insert Element at the End"<<endl;cout<<"3.Front Element of List"<<endl;cout<<"4.Last Element of the List"<<endl;cout<<"5.Size of the List"<<endl;cout<<"6.Display Forward List"<<endl;cout<<"7.Exit"<<endl;cout<<"Enter your Choice: ";cin>>choice;switch(choice){case 1:cout<<"Enter value to be inserted at the front: ";cin>>item;l.push_front(item);break;case 2:cout<<"Enter value to be inserted at the end:…arrow_forward
- #include <bits/stdc++.h> using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee* e) { cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12) << e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount << setw(9) << e->major[0]<< setw(7) << e->major[1]<<endl; } void appendNode(Employee*& head, Employee* newNode) { if (head == nullptr) { head = newNode; } else { Employee* current = head; while (current->next != nullptr) { current = current->next; } current->next = newNode; } } void displayLinkedList(Employee* head) { Employee* current = head; if(current!=nullptr){ cout…arrow_forwardC++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forward#include <iostream>using namespace std; struct Person{ int weight ; int height;}; int main(){ Person fred; Person* ptr = &fred; fred.weight=190; fred.height=70; return 0;} What is a correct way to subtract 5 from fred's weight using the pointer variable 'ptr'?arrow_forward
- demo.cpp #include "Poly.h" #include<iostream> using namespace std; int main() { Poly p1; couble d[3]={1,2,3}: Poly p2(3, d); Poly p3(p2); cout << "Polynomial p1 is: "; p1.display(); cout << "Polynomial p2 is: " << p2 << endl; cout << "Polynomial p3 is: " << p3 << endl; if (p2 == p3) { cout << "p2 and p3 are the same."; } else { cout << "p2 and p3 are different."; } cout << endl; cout << "The coefficient of power 1 of p3 is: " << p2.getCoef(1) << endl; p3.setCoef(1, 99); cout << "p3 after setup is: " << p3 << endl; if (p2 == p3) { cout << "p2 and p3 are the same."; } else { cout << "p2 and p3 are different."; } cout << endl; Poly p4; cout << "Please enter the power and coefficients of Polynomial p4: " << endl; cin >> p4; cout <<…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
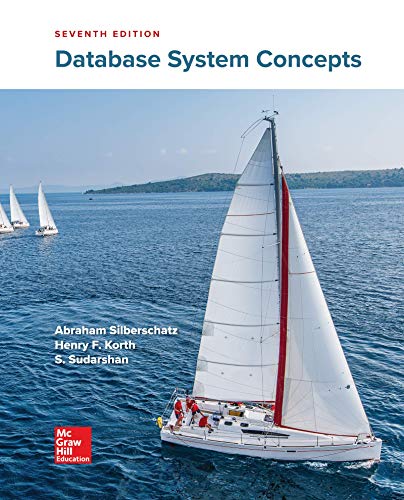
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
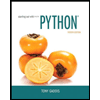
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
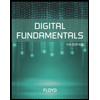
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
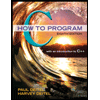
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
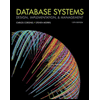
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
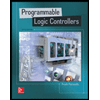
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education