#include using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee* e) { cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12) << e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount << setw(9) << e->major[0]<< setw(7) << e->major[1]<next != nullptr) { current = current->next; } current->next = newNode; } } void displayLinkedList(Employee* head) { Employee* current = head; if(current!=nullptr){ cout << left << setw(10) << "LastName "<< setw(10) << "FirstName " << setw(12)<<"# of Hours"<< setw(12) <<"Hourly Rate "<< setw(10) << "Amount" << setw(9) << "Comp Sci"<< setw(7) << "Comp Sec" << endl; } while (current != nullptr) { printRecord(current); current = current->next; } void updateData(Employee* head) { Employee* current = head; while (current != nullptr) { transform(current->firstName.begin(), current->firstName.end(), current->firstName.begin(), ::toupper); transform(current->lastName.begin(), current->lastName.end(), current->lastName.begin(), ::toupper); if (current->major[0] == 'Y') { current->amount += 1000; } if (current->major[1] == 'Y') { current->amount += 1000; } current = current->next; } } void insertNode(Employee*& head, Employee* newNode) { if (head == nullptr || head->amount > newNode->amount) { newNode->next = head; head = newNode; } else { Employee* current = head; while (current->next != nullptr && current->next->amount < newNode->amount) { current = current->next; } newNode->next = current->next; current->next = newNode; } } int main() { Employee* head = nullptr; Employee* newNode; ifstream infile("LinkedList.txt"); if (!infile) { cerr << "Error: Input file could not be opened." << endl; return 1; } string line; while (getline(infile, line)) { istringstream iss(line); newNode = new Employee; iss >> newNode->firstName >> newNode->lastName >> newNode->numOfHours >> newNode->hourlyRate; string major1, major2; iss >> major1 >> major2; newNode->major[0] = major1[0]; newNode->major[1] = major2[0]; newNode->amount = newNode->numOfHours * newNode->hourlyRate; newNode->next = nullptr; appendNode(head, newNode); } infile.close(); updateData(head); char choice; do { cout << "\nMenu:\n"; cout << "a. Create a linked list\n"; cout << "b. Update data of the linked list\n"; cout << "c. Insert a new node to the linked list\n"; cout << "d. Delete a new node to the linked list\n"; cout << "e. Display linked list\n"; cout << "f. Exit\n"; cout << "Enter your choice: "; cin >> choice; switch (choice) { case 'a': break; case 'b': updateData(head); break; case 'c': { Employee* newNode = new Employee; insertNode(head, newNode); break; } } LinkedList.txt below John Wills 45 18.0 N Y Hank Tom 20 12.0 Y Y Willam Osman 42 21.0 Y N Nick Miller 20 20.0 Y N Jeff Schitte 33 25.0 N N John King 20 32.0 Y N
#include using namespace std; struct Employee { string firstName; string lastName; int numOfHours; float hourlyRate; char major[2]; float amount; Employee* next; }; void printRecord(Employee* e) { cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12) << e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount << setw(9) << e->major[0]<< setw(7) << e->major[1]<next != nullptr) { current = current->next; } current->next = newNode; } } void displayLinkedList(Employee* head) { Employee* current = head; if(current!=nullptr){ cout << left << setw(10) << "LastName "<< setw(10) << "FirstName " << setw(12)<<"# of Hours"<< setw(12) <<"Hourly Rate "<< setw(10) << "Amount" << setw(9) << "Comp Sci"<< setw(7) << "Comp Sec" << endl; } while (current != nullptr) { printRecord(current); current = current->next; } void updateData(Employee* head) { Employee* current = head; while (current != nullptr) { transform(current->firstName.begin(), current->firstName.end(), current->firstName.begin(), ::toupper); transform(current->lastName.begin(), current->lastName.end(), current->lastName.begin(), ::toupper); if (current->major[0] == 'Y') { current->amount += 1000; } if (current->major[1] == 'Y') { current->amount += 1000; } current = current->next; } } void insertNode(Employee*& head, Employee* newNode) { if (head == nullptr || head->amount > newNode->amount) { newNode->next = head; head = newNode; } else { Employee* current = head; while (current->next != nullptr && current->next->amount < newNode->amount) { current = current->next; } newNode->next = current->next; current->next = newNode; } } int main() { Employee* head = nullptr; Employee* newNode; ifstream infile("LinkedList.txt"); if (!infile) { cerr << "Error: Input file could not be opened." << endl; return 1; } string line; while (getline(infile, line)) { istringstream iss(line); newNode = new Employee; iss >> newNode->firstName >> newNode->lastName >> newNode->numOfHours >> newNode->hourlyRate; string major1, major2; iss >> major1 >> major2; newNode->major[0] = major1[0]; newNode->major[1] = major2[0]; newNode->amount = newNode->numOfHours * newNode->hourlyRate; newNode->next = nullptr; appendNode(head, newNode); } infile.close(); updateData(head); char choice; do { cout << "\nMenu:\n"; cout << "a. Create a linked list\n"; cout << "b. Update data of the linked list\n"; cout << "c. Insert a new node to the linked list\n"; cout << "d. Delete a new node to the linked list\n"; cout << "e. Display linked list\n"; cout << "f. Exit\n"; cout << "Enter your choice: "; cin >> choice; switch (choice) { case 'a': break; case 'b': updateData(head); break; case 'c': { Employee* newNode = new Employee; insertNode(head, newNode); break; } } LinkedList.txt below John Wills 45 18.0 N Y Hank Tom 20 12.0 Y Y Willam Osman 42 21.0 Y N Nick Miller 20 20.0 Y N Jeff Schitte 33 25.0 N N John King 20 32.0 Y N
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
#include <bits/stdc++.h>
using namespace std;
struct Employee {
string firstName;
string lastName;
int numOfHours;
float hourlyRate;
char major[2];
float amount;
Employee* next;
};
void printRecord(Employee* e) {
cout << left << setw(10) << e->lastName << setw(10) << e->firstName << setw(12)
<< e->numOfHours << setw(12) << e->hourlyRate << setw(10) << e->amount
<< setw(9) << e->major[0]<< setw(7) << e->major[1]<<endl;
}
void appendNode(Employee*& head, Employee* newNode) {
if (head == nullptr) {
head = newNode;
} else {
Employee* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
void displayLinkedList(Employee* head) {
Employee* current = head;
if(current!=nullptr){
cout << left << setw(10) << "LastName "<< setw(10) << "FirstName "
<< setw(12)<<"# of Hours"<< setw(12) <<"Hourly Rate "<< setw(10)
<< "Amount"
<< setw(9) << "Comp Sci"<< setw(7) << "Comp Sec" << endl;
}
while (current != nullptr) {
printRecord(current);
current = current->next;
}
void updateData(Employee* head) {
Employee* current = head;
while (current != nullptr) {
transform(current->firstName.begin(), current->firstName.end(), current->firstName.begin(), ::toupper);
transform(current->lastName.begin(), current->lastName.end(), current->lastName.begin(), ::toupper);
if (current->major[0] == 'Y') {
current->amount += 1000;
}
if (current->major[1] == 'Y') {
current->amount += 1000;
}
current = current->next;
}
}
void insertNode(Employee*& head, Employee* newNode) {
if (head == nullptr || head->amount > newNode->amount) {
newNode->next = head;
head = newNode;
} else {
Employee* current = head;
while (current->next != nullptr && current->next->amount < newNode->amount) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
int main() {
Employee* head = nullptr;
Employee* newNode;
ifstream infile("LinkedList.txt");
if (!infile) {
cerr << "Error: Input file could not be opened." << endl;
return 1;
}
string line;
while (getline(infile, line)) {
istringstream iss(line);
newNode = new Employee;
iss >> newNode->firstName >> newNode->lastName >> newNode->numOfHours >> newNode->hourlyRate;
string major1, major2;
iss >> major1 >> major2;
newNode->major[0] = major1[0];
newNode->major[1] = major2[0];
newNode->amount = newNode->numOfHours * newNode->hourlyRate;
newNode->next = nullptr;
appendNode(head, newNode);
}
infile.close();
updateData(head);
char choice;
do {
cout << "\nMenu:\n";
cout << "a. Create a linked list\n";
cout << "b. Update data of the linked list\n";
cout << "c. Insert a new node to the linked list\n";
cout << "d. Delete a new node to the linked list\n";
cout << "e. Display linked list\n";
cout << "f. Exit\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 'a':
break;
case 'b':
updateData(head);
break;
case 'c': {
Employee* newNode = new Employee;
insertNode(head, newNode);
break;
}
}
LinkedList.txt below
John Wills 45 18.0 N Y
Hank Tom 20 12.0 Y Y
Willam Osman 42 21.0 Y N
Nick Miller 20 20.0 Y N
Jeff Schitte 33 25.0 N N
John King 20 32.0 Y N
Hank Tom 20 12.0 Y Y
Willam Osman 42 21.0 Y N
Nick Miller 20 20.0 Y N
Jeff Schitte 33 25.0 N N
John King 20 32.0 Y N

Transcribed Image Text:Linked Lists
These are the instructions
Create a meu-driven linked list program, and develop and use the following menu to implement
the linked list, modifying the linked list program from the labs in class.
a. Create a linked list (need to read from the "LinkedList.txt" to create the list)
Create a new node
Append them in ascending order of Lname
b. Update data of the linked list
c. Insert a new node to the linked list
d. Delete a new node to the linked list
e. Display linked list
f. Exit
modify the code it based on the instructions above and modifications below:
-modify case a to what is needed in the intructions
-clicking b which will modify only the last name to uppercase (the first and last name are already
uppercase before I press b which shouldn't be happening, and 'b' is used for another feature not
just changing it to uppercase) maybe use an if statement and ask the user if they want to
change it to uppercase for the first or last name, or
-increase the hourly rate by 10 for comp sci majors, but keep the adding 1000 for comp
sci and cyber security majors (2000 for both)
-clicking b should also add 10 to the hourly rate for computer science majors when
updating data so if you could implement that, please
(for the b selection, make an if statement and ask the user if they would like to either
modify the last names to uppercase or to increase the hourly rate and have it save to so
when the user types display list, then the saved changes are there)
-the last thing is when clicking c, you should be able to insert a new node to the
sequence with the amount calculated and display it, make it so the user can enter in the
new node and have it displayed. Ask the user how many they want to input, then make a
loop to have them insert the new nodes and user input. Display the new employee in the
list with the amount calculated
please do it in a timely manner
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
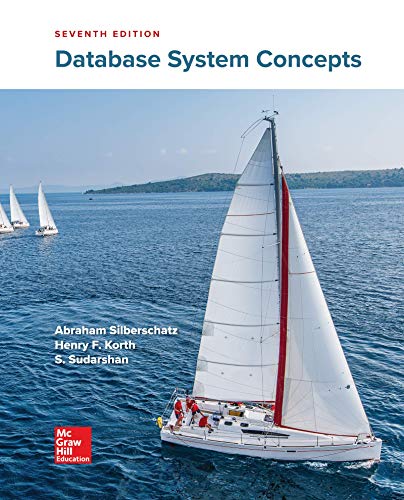
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
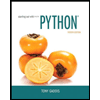
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
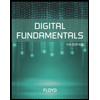
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
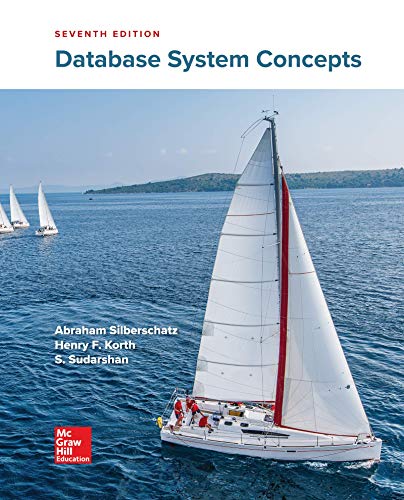
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
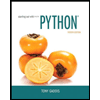
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
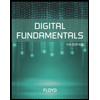
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
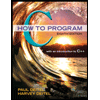
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
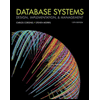
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
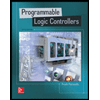
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education