Step 1b: Modify some methods Make the following changes to your DynamicArray code: 1. Add the word const at the end of the at, sum, print, and cap function headers in both the .cpp and the .h files. For example: int sum() const; O O int cap const { return capacity; } 2. Modify print to take an output stream as a parameter instead of assuming cout. Here's the new prototype for the .h file: O void print(std::ostream& s) const; Change the implementation in the .cpp file too. All you have to do is replace "cout" with "s". Recompile your code. If it still compiles, you should be good to go. If it doesn't, the answer might be that you made an unnecessary/incorrect modification to the array within one of these functions! Compile and run the new program, and verify that it shows you still pass the Part 1 tests. Step 2: Copy Constructor The next step is to define a copy constructor for DynamicArray. How do we implement a copy constructor? Technically, it's up to you, since you're the one writing the class. But 90+% of the time, you'll do the following for each member variable: 1. If the member variable is not a pointer: copy it directly from the other object 2. If the member variable IS a pointer to dynamically allocated memory: allocate your own space in memory, and copy the contents from the other object as needed Note that, in the example used in class (Point2D), the copy constructor was very simple because both member variables were not pointers. However, our DynamicArray class has a member variable that is a pointer For declaration: Note the general form of the prototype below. Modify it for this class and add it to the header file. Classname(const Classname& source); For definition: follow the prompts below: // capacity and len are ints, so they're covered by case 1 capacity= len = // arr points to dynamically allocated memory. // Directly copying arr = other.arr doesn't actually // COPY that memory it just means we have two DynamicArray //objects referencing the same underlying storage. // So we have to first allocate new memory for this new object, // and then copy over the values from the other object. arr = // Copy the elements from other array to our new space for (int i = 0; i < len; i++) { "}, This code only copies the actual elements, not the garbage in the empty slots at the end of the array When you are done, un-comment these lines in main.cpp: DynamicArray b(a); cout << "*** Lab 6 (Part 1) tests on array created with copy constructor: " << (RunPart1Tests (b)? "passed" : "failed") << endl; Here's what's happening in your code now, in order: 1. You create an empty DynamicArray, a. 2. You create a DynamicArray, b, as a copy of a (so it's also empty). 3. You run the Part 1 tests on a, which add and remove a bunch of values. They leave the array with only one element: 152. 4. You run the same tests on b, with the same result. Compile this code and verify that you passed the tests.
Step 1b: Modify some methods Make the following changes to your DynamicArray code: 1. Add the word const at the end of the at, sum, print, and cap function headers in both the .cpp and the .h files. For example: int sum() const; O O int cap const { return capacity; } 2. Modify print to take an output stream as a parameter instead of assuming cout. Here's the new prototype for the .h file: O void print(std::ostream& s) const; Change the implementation in the .cpp file too. All you have to do is replace "cout" with "s". Recompile your code. If it still compiles, you should be good to go. If it doesn't, the answer might be that you made an unnecessary/incorrect modification to the array within one of these functions! Compile and run the new program, and verify that it shows you still pass the Part 1 tests. Step 2: Copy Constructor The next step is to define a copy constructor for DynamicArray. How do we implement a copy constructor? Technically, it's up to you, since you're the one writing the class. But 90+% of the time, you'll do the following for each member variable: 1. If the member variable is not a pointer: copy it directly from the other object 2. If the member variable IS a pointer to dynamically allocated memory: allocate your own space in memory, and copy the contents from the other object as needed Note that, in the example used in class (Point2D), the copy constructor was very simple because both member variables were not pointers. However, our DynamicArray class has a member variable that is a pointer For declaration: Note the general form of the prototype below. Modify it for this class and add it to the header file. Classname(const Classname& source); For definition: follow the prompts below: // capacity and len are ints, so they're covered by case 1 capacity= len = // arr points to dynamically allocated memory. // Directly copying arr = other.arr doesn't actually // COPY that memory it just means we have two DynamicArray //objects referencing the same underlying storage. // So we have to first allocate new memory for this new object, // and then copy over the values from the other object. arr = // Copy the elements from other array to our new space for (int i = 0; i < len; i++) { "}, This code only copies the actual elements, not the garbage in the empty slots at the end of the array When you are done, un-comment these lines in main.cpp: DynamicArray b(a); cout << "*** Lab 6 (Part 1) tests on array created with copy constructor: " << (RunPart1Tests (b)? "passed" : "failed") << endl; Here's what's happening in your code now, in order: 1. You create an empty DynamicArray, a. 2. You create a DynamicArray, b, as a copy of a (so it's also empty). 3. You run the Part 1 tests on a, which add and remove a bunch of values. They leave the array with only one element: 152. 4. You run the same tests on b, with the same result. Compile this code and verify that you passed the tests.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++ Code
dynamicarray.h and dynamicarray.cpp

Transcribed Image Text:Step 1b: Modify some methods
Make the following changes to your DynamicArray code:
1. Add the word const at the end of the at, sum, print, and cap function headers in both the .cpp and the .h files. For example:
int sum const;
O
int cap const { return capacity; }
2. Modify print to take an output stream as a parameter instead of assuming cout. Here's the new prototype for the .h file:
0 void print(std::ostream& s) const;
O
Change the implementation in the .cpp file too. All you have to do is replace "cout" with "s".
Recompile your code. If it still compiles, you should be good to go. If it doesn't, the answer might be that you made an unnecessary/incorrect modification to the array within one of these functions!
Compile and run the new program, and verify that it shows you still pass the Part 1 tests.
Step 2: Copy Constructor
The next step is to define a copy constructor for DynamicArray.
How do we implement a copy constructor?
Technically, it's up to you, since you're the one writing the class. But 90+% of the time, you'll do the following for each member variable:
1. If the member variable is not a pointer: copy it directly from the other object
2. If the member variable IS a pointer to dynamically allocated memory: allocate your own space in memory, and copy the contents from the other object as needed
Note that, in the example used in class (Point2D), the copy constructor was very simple because both member variables were not pointers. However, our DynamicArray class has a member variable that is a pointer.
For declaration: Note the general form of the prototype below. Modify it for this class and add it to the header file.
Classname(const Classname& source);
For definition: follow the prompts below:
// capacity and len are ints, so they're covered by case 1
capacity=
len =
// arr points to dynamically allocated memory.
// Directly copying arr = other.arr doesn't actually
// COPY that memory -- it just means we have two DynamicArray
// objects referencing the same underlying storage.
// So we have to first allocate new memory for this new object,
// and then copy over the values from the other object.
arr =
// Copy the elements from other array to our new space
for (int i = 0; i < len; i++) {
// This code only copies the actual elements, not the garbage
// in the empty slots at the end of the array
When you are done, un-comment these lines in main.cpp:
DynamicArray b(a);
cout << "*** Lab 6 (Part 1) tests on array created with copy constructor: "
<< (RunPart1Tests (b) ? "passed" : "failed") <<< endl;
Here's what's happening in your code now, in order:
1. You create an empty DynamicArray, a.
2. You create a DynamicArray, b, as a copy of a (so it's also empty).
3. You run the Part 1 tests on a, which add and remove a bunch of values. They leave the array with only one element: 152.
4. You run the same tests on b, with the same result.
Compile this code and verify that you passed the tests.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
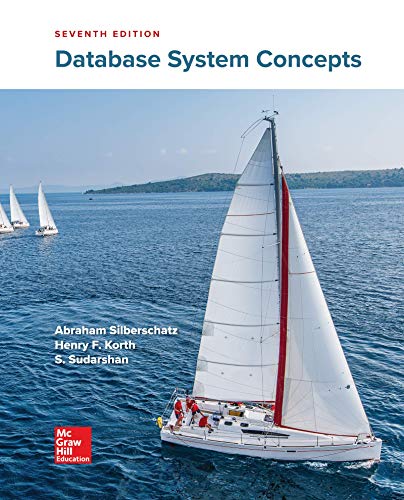
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
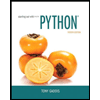
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
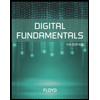
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
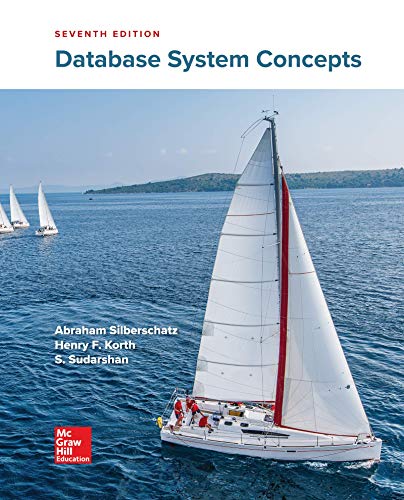
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
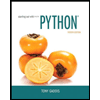
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
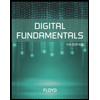
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
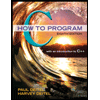
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
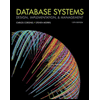
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
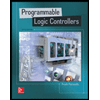
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education