using C++ 1- Creates a class called Member with two integer x and y. a. Add a constructor able to create a Member object with tow integers and the default values 0,0. b. Add the methods setX and setY to modify the attributes x and y; [2 Mark c. Add the method display able to display the attributes. 2- A Stack is a special array where the insertion and deletion will be via a specific index called "head". A Stack is characterized by 3 attributes: a. capacity (int): the maximum number element Member that can be contained into the Stack. b. head: presents the index where we can add/remove element to the Stack. The head value presents also the current number of elements into the Stack. When a Stack is created the initial value of head is 0. c. Member content[]: an array of elements of type Member. Creates the class Stack with the following methods: a. bool empty(): this method returns true if no element exists in the Stack. b. bool full(): this method returns true if there are no place to add a new element to the Stack. c. overload the operator += (Member): able to add an element to the Stack. You need to be sure that there is an available space in the Stack: (use assert (condition); from , the condition must describe the existence of an available space). When a member is added, the head index will be increased. d. delete(): able to delete an element from the Stack. You need to be sure that there is at least one available member in the Stack (use the assert function). When a member is deleted, the head index will be decreased. e. display(): a method able to display all the member elements existing in the Stack; 3- Creates a function main() to test the program: a. Creates a Stack. b. Creates 3 Members and then insert all of them into the Stack. c. Display the Stack. d. Delete an element from the Stack.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
using C++
1- Creates a class called Member with two integer x and y.
a. Add a constructor able to create a Member object with tow integers and the default values 0,0.
b. Add the methods setX and setY to modify the attributes x and y; [2 Mark
c. Add the method display able to display the attributes.
2- A Stack is a special array where the insertion and deletion will be via a specific index called "head". A Stack is characterized by 3 attributes:
a. capacity (int): the maximum number element Member that can be contained into the Stack.
b. head: presents the index where we can add/remove element to the Stack. The head value presents also the current number of elements into the Stack. When a Stack is created the initial value of head is 0.
c. Member content[]: an array of elements of type Member.
Creates the class Stack with the following methods:
a. bool empty(): this method returns true if no element exists in the Stack.
b. bool full(): this method returns true if there are no place to add a new
element to the Stack.
c. overload the operator += (Member): able to add an element to the Stack. You need to be sure that there is an available space in the Stack: (use assert (condition); from <cassert>, the condition must describe the existence of an available space). When a member is added, the head index will be increased.
d. delete(): able to delete an element from the Stack. You need to be sure that there is at least one available member in the Stack (use the assert function). When a member is deleted, the head index will be decreased.
e. display(): a method able to display all the member elements existing in
the Stack;
3- Creates a function main() to test the program:
a. Creates a Stack.
b. Creates 3 Members and then insert all of them into the Stack.
c. Display the Stack.
d. Delete an element from the Stack.
e. Display the Stack for one more time.


Step by step
Solved in 4 steps with 2 images

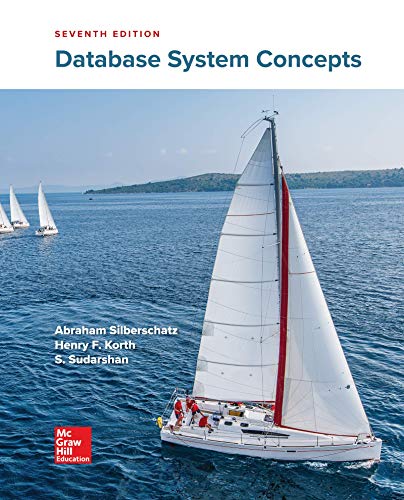
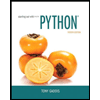
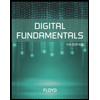
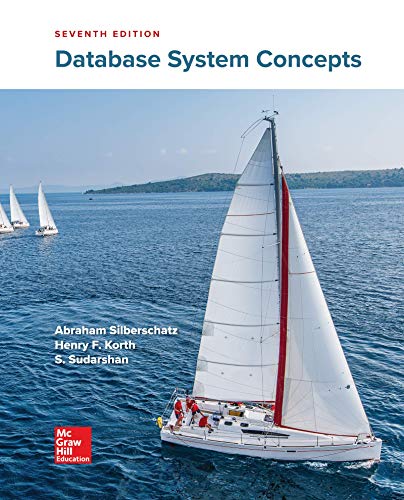
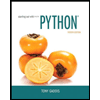
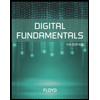
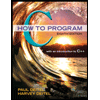
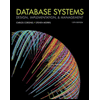
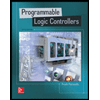