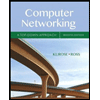
Concept explainers
create using c++
One problem with dynamic arrays is that once the array is created using the new operator the size cannot be changed. For example, you might want to add or delete entries from the array similar to the behavior of a
The class should have the following
A private member variable called dynamicArray that references a dynamic array of type string.
A private member variable called size that holds the number of entries in the array.
A default constructor that sets the dynamic array to NULL and sets size to 0.
A function that returns size .
A function named addEntry that takes a string as input. The function should create a new dynamic array one element larger than dynamicArray , copy all elements from dynamicArray into the new array, add the new string onto the end of the new array, increment size, delete the old dynamicArray , and then set dynamicArray to the new array.
A function named deleteEntry that takes a string as input. The function should search dynamicArray for the string. If not found, it returns false . If found, it creates a new dynamic array one element smaller than dynamicArray .It should copy all elements except the input string into the new array, delete dynamicArray , decrement size, and return true .

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 9 images

- In C++ Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string). Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list. Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list. Write member functions to: remove and return the last line from the list add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array empty the entire list return the number of lines still on the list take two lists and return one combined list (with no duplicates) copy constructor to support deep copying remember the destructor!arrow_forwardSmart Pointer: Write a smart pointer class. A smart pointer is a data type, usually implemented with templates, that simulates a pointer while also providing automatic garbage collection. It automatically counts the number of references to a SmartPointer<?> object and frees the object of type T when the reference count hits zero.arrow_forwardWrite a simple trivia quiz game using c++ Start by creating a Trivia class that contains information about a single trivia question. The class should contain a string for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should be worth more). Add appropriate constructor and accessor functions. In your main function create either an array or a vector of type Trivia and hard-code at least five trivia questions of your choice. Your program should then ask each question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. If so, award the player the dollar amount for that question. If the player enters the wrong answer your program should display the correct answer. When all questions have been asked display the total amount that the player has won.arrow_forward
- IN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forwardPointer and classImplement a class called Team as specified:data members:name - the name of the Team (defined as a dynamic variable)members - a dynamic array of stringsize - number of members in the team.functions:Course(): default constructor set name to “TBD”, and size to 0, members toempty listCourse(string): one argument constructor set name, and size to 0, members toempty listaccessor - an accessor for the name and size variablemutator - an mutator for the name variableUse following main() to test your class.int main() {Team a,b("Mets");cout<<a.getName()<<endl; // print TBDa.setName("Yankee");cout<<a.getName()<<endl; // print Yankeecout<<b.getName()<<endl; // print Metscout<<a.getSize()<<endl; // print 0return 0;}arrow_forwardarray of Payroll ObjectsDesign a PayRoll class that has data members for an employee’shourly pay rate and number of hours worked. Write a program withan array of seven PayRoll objects. The program should read thenumber of hours each employee worked and their hourly pay ratefrom a file and call class functions to store this information in theappropriate objects. It should then call a class function, once foreach object, to return the employee’s gross pay, so this informationcan be displayed.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
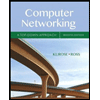
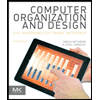
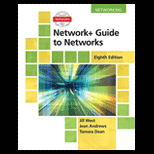
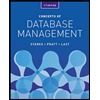
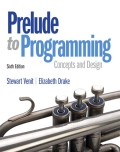
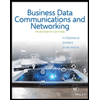