True/ False 1- Class objects can be defined prior to the class declaration. 2- You must use the private access specification for all data members of a class. 3- If an array is partially initialized, the uninitialized elements will be set to zero. 4- A vector object automatically expands in size to accommodate the items stored in it. 5- With pointer variables, you can access but not modify data in other variables. 6- Assuming myValues is an array of int values and index is an int variable, both of the following statements do the same thing. 1. cout << myValues [index] << endl; 2. cout << * (myValues + index) << endl; 7- An alternative to using the open member function is to use the file stream object declaration itself to open the file. For example: fstream DataFile ("names.dat", ios::in | ios::out); 8- When passing a file stream object to a function, you should always pass it by reference. 9- The try/catch/throw construct is able to handle only one type of exception in a try block. 10- The try block of a try/catch construct is used to display the definition of an exception parameter. 11- In an inheritance situation, you may not pass arguments to a base class constructor. 12- A derived class may become a base class if another class is derived from it. 13- The Standard Template Library (STL) contains templates for useful algorithms and data structures. 14- The STL provides many algorithms which are implemented as function templates and are included in the header file. 15- To solve a problem recursively, you must identify at least one case in which the problem can be solved without recursion.
True/ False 1- Class objects can be defined prior to the class declaration. 2- You must use the private access specification for all data members of a class. 3- If an array is partially initialized, the uninitialized elements will be set to zero. 4- A vector object automatically expands in size to accommodate the items stored in it. 5- With pointer variables, you can access but not modify data in other variables. 6- Assuming myValues is an array of int values and index is an int variable, both of the following statements do the same thing. 1. cout << myValues [index] << endl; 2. cout << * (myValues + index) << endl; 7- An alternative to using the open member function is to use the file stream object declaration itself to open the file. For example: fstream DataFile ("names.dat", ios::in | ios::out); 8- When passing a file stream object to a function, you should always pass it by reference. 9- The try/catch/throw construct is able to handle only one type of exception in a try block. 10- The try block of a try/catch construct is used to display the definition of an exception parameter. 11- In an inheritance situation, you may not pass arguments to a base class constructor. 12- A derived class may become a base class if another class is derived from it. 13- The Standard Template Library (STL) contains templates for useful algorithms and data structures. 14- The STL provides many algorithms which are implemented as function templates and are included in the header file. 15- To solve a problem recursively, you must identify at least one case in which the problem can be solved without recursion.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 17PE
Related questions
Question
![True/ False
1- Class objects can be defined prior to the class declaration.
2- You must use the private access specification for all data members of a class.
3- If an array is partially initialized, the uninitialized elements will be set to zero.
4- A vector object automatically expands in size to accommodate the items stored in it.
5- With pointer variables, you can access but not modify data in other variables.
6- Assuming myValues is an array of int values and index is an int variable, both of the
following statements do the same thing.
1.
cout << myValues [index] << endl;
cout << * (myValues + index) << endl;
7- An alternative to using the open member function is to use the file stream object declaration
2.
itself to open the file. For example:
fstream DataFile ("names.dat", ios::in | ios::out);
8- When passing a file stream object to a function, you should always pass it by reference.
9- The try/catch/throw construct is able to handle only one type of exception in a try block.
10- The try block of a try/catch construct is used to display the definition of an exception parameter.
11- In an inheritance situation, you may not pass arguments to a base class constructor.
12- A derived class may become a base class if another class is derived from it.
13- The Standard Template Library (STL) contains templates for useful algorithms and data
structures.
14- The STL provides many algorithms which are implemented as function templates and are
included in the <algorithm> header file.
15- To solve a problem recursively, you must identify at least one case in which the problem can be
solved without recursion.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6ca4b84d-c518-47ce-bd33-9b129e18b52b%2F601cd87c-0ce7-40c2-9d3b-8fcf961689a1%2F26gx3o_processed.jpeg&w=3840&q=75)
Transcribed Image Text:True/ False
1- Class objects can be defined prior to the class declaration.
2- You must use the private access specification for all data members of a class.
3- If an array is partially initialized, the uninitialized elements will be set to zero.
4- A vector object automatically expands in size to accommodate the items stored in it.
5- With pointer variables, you can access but not modify data in other variables.
6- Assuming myValues is an array of int values and index is an int variable, both of the
following statements do the same thing.
1.
cout << myValues [index] << endl;
cout << * (myValues + index) << endl;
7- An alternative to using the open member function is to use the file stream object declaration
2.
itself to open the file. For example:
fstream DataFile ("names.dat", ios::in | ios::out);
8- When passing a file stream object to a function, you should always pass it by reference.
9- The try/catch/throw construct is able to handle only one type of exception in a try block.
10- The try block of a try/catch construct is used to display the definition of an exception parameter.
11- In an inheritance situation, you may not pass arguments to a base class constructor.
12- A derived class may become a base class if another class is derived from it.
13- The Standard Template Library (STL) contains templates for useful algorithms and data
structures.
14- The STL provides many algorithms which are implemented as function templates and are
included in the <algorithm> header file.
15- To solve a problem recursively, you must identify at least one case in which the problem can be
solved without recursion.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
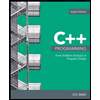
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
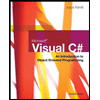
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
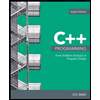
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
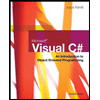
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,